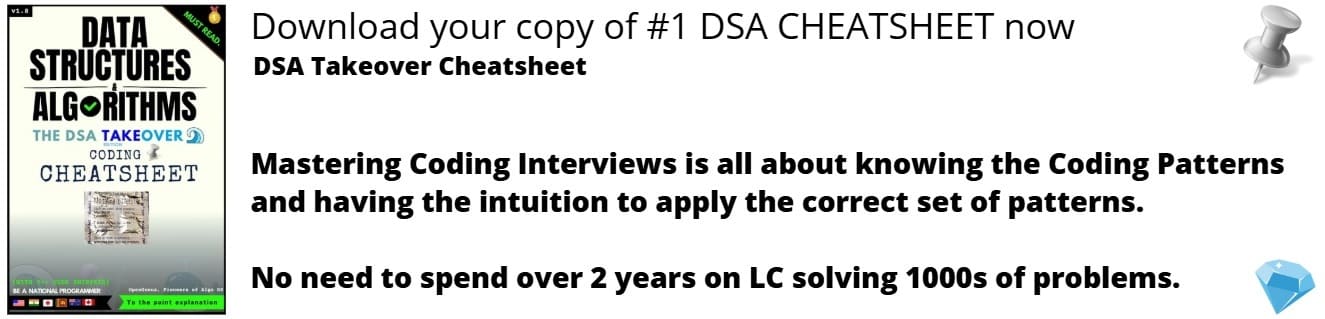
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
This article describes the process of creating a guessing game through a Console Application using C#. By the end of reading this, you will know how to handle user output/input, exception handling, while loops, and selection statements with if/else if/else.
First, we'll define what our game is. Our guessing game will have the user guess a number from 1 to 100. We will check to make sure the proper input is being used. If the guess is too low, or too high, we will tell them appropriately. The user can continue to guess until they get the right number. Finally, when the correct number is guessed, we will tell the user and end the game.
Create a new console application using the IDE of your choice and let's get started!
Let's first generate a random number between 1 and 100. We create a new Random object, then use the Next method to generate a number. Note the first number is inclusive, the last number is exclusive. This is why we need (1, 101) to generate a 1-100 number.
// Generate a random number between 1 and 100
Random rand = new();
int answer = rand.Next(1, 101);
We'll give a greeting next.
Console.WriteLine("Let's play a guessing game!");
Next, we're going to use a while loop. This will use the parameter of true, meaning this will continue to loop infinitely unless we specify the break keyword, which we will see later. You can specify any statement here that results in a boolean value, but this is not our purpose here.
Then we'll set a variable for the user's guess and give the user a message to enter a guess. Note we use Console.Write here rather than Console.WriteLine. This is to allow the user input to be on the same line as the message.
// Start loop
while (true)
{
int guess;
Console.Write("Enter a number between 1 and 100: ");
}
Before we go further, I'd like to point out a personal preference that I do with most of my console projects when I use Console commands a lot. With this project, we will be using Console.WriteLine() frequently; you can use what is called a using static directive so that we can simply use WriteLine() instead. This is purely preference but going forward, I'll be using this through the article.
At the very top of the file, simply add:
using static System.Console;
At this point, you can remove Console from the Write and WriteLine statements.
Your full code should now look like this:
using static System.Console;
// Generate random number between 1 and 100
Random rand = new();
int answer = rand.Next(1, 101);
WriteLine("Let's Play a guessing game!");
// Starts loop
while (true)
{
int guess;
Write("Enter a number between 1 and 100: ");
}
Next, we're going to get the user input. ReadLine is used for this. When the user hits their enter key, ReadLine will trigger. We're going to use something called exception handling in order to make sure that the user entered in a number. If you'd like more information on how exception handling works, see my article here that dives deeper into the topic.
Exception Handling in C#Add this after the Write statement that asks for a number. Note that we use the keyword continue in the catch block. This will force the code to go back to the beginning of the while (true) loop, printing the enter a number message again.
// Try to parse the string into an int
try
{
guess = int.Parse(ReadLine());
}
catch
{
WriteLine("\nYour guess needs to be a number.");
continue;
}
Now we'll check to make sure that the user entered in a number between 1 and 100. This is done using an if statement. The condition(s) in the paranthesis must evaluate to a boolean value (true or false). Here we will check if the guess is greater than 100 or less than one. If so, we print an error message and again continue to the beginning of the loop.
if (guess > 100 || guess < 1)
{
WriteLine("Your guess needs to be between 1 and 100.");
continue;
}
Finally, we'll see if the guess is too high, too low, or correct. We will be doing this using if/else if/else. It works pretty much exactly how it sounds. The first if statement gets checked. If it is found to be false, it goes to the next statement, in this case being an else if statement. The final statement, else, gets executed if no other statements are found to be true. One final thing to explain is break. This will break out of the loop, thus ending our program.
Let's see how this is done; add the following code below your earlier if statement.
if (guess < answer)
{
WriteLine($"\nYour guess of {guess} is too low.");
}
else if (guess > answer)
{
WriteLine($"\nYour guess of {guess} is too high.");
}
// Winner!
else
{
WriteLine($"\n\nYou guessed correctly! The number is {answer}!");
WriteLine($"Hit enter to close.");
ReadLine();
break;
}
Running this will play your newly created guessing game!
Now that you've built a guessing game, try adding more to it! You could have a limited number of guesses, difficulty levels, or whatever else you desire!
Putting everything we've learned together, here's our final code:
using static System.Console;
// Generate random number between 1 and 100
Random rand = new();
int answer = rand.Next(1, 101);
WriteLine("Let's Play a guessing game!");
// Start loop
while (true)
{
int guess;
Write("Enter a number between 1 and 100: ");
// Try to parse the string into an int
try
{
guess = int.Parse(ReadLine());
}
catch
{
WriteLine("\nYour guess needs to be a number.");
continue;
}
// Guess is not a valid number
if (guess > 100 || guess < 1)
{
WriteLine("Your guess needs to be between 1 and 100.");
continue;
}
if (guess < answer)
{
WriteLine($"\nYour guess of {guess} is too low.");
}
else if (guess > answer)
{
WriteLine($"\nYour guess of {guess} is too high.");
}
// Winner!
else
{
WriteLine($"\n\nYou guessed correctly! The number is {answer}!");
WriteLine($"Hit enter to close.");
ReadLine();
break;
}
}