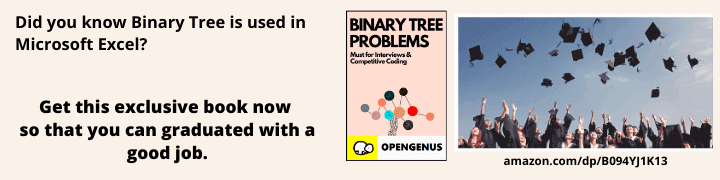
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A for loop allows us to write a loop that is executed a specific number of times. The loop enables us to perform n number of steps together in one line.
Syntax
for (initialization expr; test expr; update expr)
{
// body of the loop
// statements we want to execute
}
In for loop, a loop variable is used to control the loop. First initialize this loop variable to some value, then check whether this variable is less than or greater than counter value. If statement is true, then loop body is executed and loop variable gets updated according to update expression. Steps are repeated till exit condition comes.
Flow-diagram
Here is stepwise flow of control in for loop −
The initialisation step is executed first, and only once. This step declare and initialize any loop control variables. You are not required to put a statement here, as long as a semicolon appears.
Next, the condition is evaluated. If it is true, the body of the loop is executed. If it is false, the body of the loop does not execute and flow of control jumps to the next statement just after the for loop.
After the body of the for loop executes, the flow of control jumps back up to the increment statement. This statement can be left blank, as long as a semicolon appears after the condition.
The condition is now evaluated again. If it is true, the loop executes and the process repeats itself (body of loop, then increment step, and then again condition). After the condition becomes false, the for loop terminates.
Example
write a program to print 'OpenGenus' 10 times using for loop.
int main ()
{
// for loop execution
for( int a = 10; a < 20; a = a + 1 )
{
cout << "OpenGenus"<< endl;
}
return 0;
}
Output
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
OpenGenus
All the best!