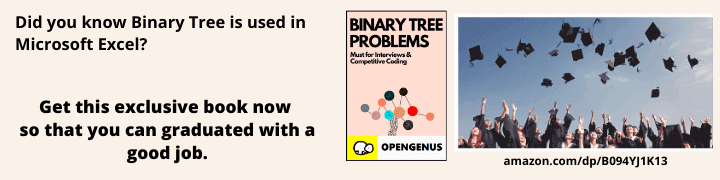
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction to List in Python
Lists are a staple data structure in any python programmer’s toolkit. It is not only versatility but efficient also. In this OpenGenus article, I will discuss a variety of methods in the standard library to add an element or an iterable to a list in Python.
We will discuss the following methods to add elements to a list:
- Append()
- Insert()
- Extend()
- List concatenation
- Slice
- List comprehension
Table of contents
- Prerequisites
- append()
- insert()
- extend()
- List concatenation
- Slice
- List comprehension
- Key takeaways
- Conclusion
- References
Prerequisites
The definition of a list is as follows, a dynamic data structure, which accepts multiple datatypes if desired and can be changed. The list data structure has a starting index of 0.
Note: Time complexities are for CPython.
Note: n stands for the number of elements in the list and k is for the value or size of the parameter.
append()
The first method that I want to discuss is the append() function. The method append() adds an element to the end of a list.
ls = [1, 2, 3, 4, 4]
ls.append(3)
# [1, 2, 3, 4, 4, 3]
The time complexity of this operation is O(1)
insert()
Another method is insert() which adds the element in a specified position. The method takes two arguments. First, the index – the position- and the element that you want to add.
Note: insert() returns None.
Ls = [1, 2, 3, 4, 4]
Ls.insert(0, 3)
# [3, 1, 2, 3, 4, 4]
Its time complexity is O(n)
extend()
The extend() function takes as an argument an iterable and it adds its elements to the end of the list it is appending them to.
Ls = [1, 2, 3, 4, 4]
Ls.extend([“cars”])
# [3, 1, 2, 3, 4, 4, “cars”]
The time complexity is O(k)
List concatenation
List concatenation is when + is used to join an element to a list. With this method it is significant to mention that every time you add an element you make a new list, taking more space.
Ls = [1, 2, 3, 4, 4]
Ls += [“cars”]
# [3, 1, 2, 3, 4, 4, “cars”]
The time complexity is O(n^2).
Slice
A slice is a way to access a range of elements in a list.
This is the syntax for a slice:
list[start_index : end_index : step)
- A step decides the by what value the start_index increases by
Note: All of the arguments are optional.
Note: the returned list ends at end_index -1 because a list’s index starts at 0.
You can think of a slice as a formula to find the last item and the starting item in a list. First let’s rename the arguments.
- Start_index = t
- Any index = n
- Step = c
For example
For the list [2,4,6,8,10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30]. Where,
- t = 2
- c = 2
Making the formula 2n+2. You can generate an arithmetic sequence of new indexes.
Figure 1: A table with values and the new indexes using the formula 2n+2
We will be working with the slice [2:7:2].
The first item is (2*2 + 2) = index 6. Which corresponds to value 6.
The last item is (2*7 + 2) = index 14. Which corresponds to value 6.
The range is now is [6, 8, 10, 12, 14].
Now you need to remove every second value, since we are going is steps of two. Making the final solution [6, 10, 14].
I know that this method could be considered unnecessary way to think about it when you can just count. The idea is to present another way to think about it and show a link between maths and programming.
Here are more examples that you are likely to see in the wild:
languages = ["Python", "Swift", "C++"]
print(languages[-1]) #negative indexing
# C++
print(languages[-3:-1]) # last one is not included
# ['Python', 'Swift']
#slicing
my_list = ['p','r','o','g','r','a','m','i','z']
print(my_list[2:5]) # 2 to 4
# ['o', 'g', 'r']
print(my_list[5:]) # 5 to the end
# ['a', 'm', 'i', 'z']
print(my_list[:]) # whole list
# ['p', 'r', 'o', 'g', 'r', 'a', 'm', 'i', 'z']
print(my_list[:5]) # beguining to 4
# ['p', 'r', 'o', 'g', 'r']
The time complexity is O(k) for slicing.
List comprehension
List comprehension is another way create or iterate through a list.
This is the syntax of list comprehension:
[expression for element in list]
You can use one or more if statements in a list comprehension to filter undesirable elements.
This is the syntax:
[expression for element in list if expression]
Look at the following examples:
Example 1: using if
even_numbers = [even_num for even_num in range(20) if even_num % 2 == 0]
print(even_numbers)
# [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
Time complexity is
O(n)
Example 2: using if else
[element if expression else element for element in list ]
odd_even = ["Even" if any_num % 2 == 0 else "Odd" for any_num in range(5)]
print(odd_even)
# ['Even', 'Odd', 'Even', 'Odd', 'Even']
Time complexity is O(n)
Example 3: nested for loop
This example prints a number and whether it is even or odd for the numbers 0 to 9 as a tuple. Then prints that again. Making a 2d list.
Syntax for the nexted loop
[[expression for element in list] for element in list]
nums = [[("Even",any_num) if any_num % 2 == 0 else ("Odd",any_num) for any_num in range(10)] for any_num in range(2)]
print (nums)
#[[('Even', 0), ('Odd', 1), ('Even', 2), ('Odd', 3), ('Even', 4), ('Odd', 5), ('Even', 6), ('Odd', 7), ('Even', 8), ('Odd', 9)], [('Even', 0), ('Odd', 1), ('Even', 2), ('Odd', 3), ('Even', 4), ('Odd', 5), ('Even', 6), ('Odd', 7), ('Even', 8), ('Odd', 9)]]
Time complexity is O(n2)
Key takeaways
- append adds an element to the end of a list
- insert() add the element in the index specified.
-
- can add an element or an iterable.
- extend() adds an iterable’s contents to the end of the list.
- You can slice a list to add an element or iterable to it.
- List comprehension enables you to filter elements during the creation of the list.
- You can make a multi-dimensional list with list comprehension.
Conclusion
There a variety of ways to add an element to a list. There is no significant difference between then so which one you use is up to you.