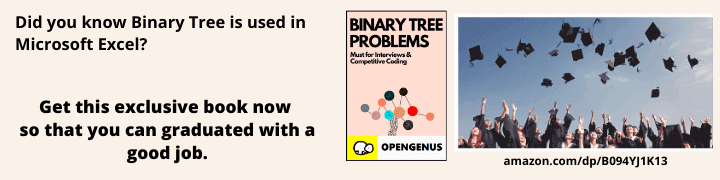
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
Ping pong, a classic arcade game loved by generations, has been a source of entertainment and competition for players of all ages. Now, imagine creating your own version of this beloved game and taking it to new heights. With the power of Python and the Pygame library, you can embark on this exciting journey of coding your very own ping pong game.
In this article at OpenGenus, we will walk you through the process of building a simple yet engaging ping pong game using Pygame.
Getting Started
Before diving into the code, let's set up the development environment. Ensure you have Python installed on your system (Python 3.x recommended) and install the Pygame library using the following command:
pip install pygame
Requirements and Rules
1. Game Window: We'll create a game window using Pygame with a width of 800 pixels and a height of 400 pixels. This window will serve as the playing area for our Ping Pong game.
2. Paddles and Ball: The game will have two paddles, one for each player, and a ball moving between them. The paddles will be represented by rectangular shapes, while the ball will be represented by a circular shape.
3. Controls: Players will use the 'W' and 'S' keys to control the left paddle (moving it up and down) and the up and down arrow keys to control the right paddle.
4. Scoring: The game will continue until one player scores a point. Each time the ball passes the opponent's paddle, the scoring player will earn a point, and the ball will reset to the center for the next round.
5. Collision Detection: We'll implement collision detection to make sure the ball bounces off the paddles when it comes into contact with them. This will add an element of skill and strategy to the game.
6. Game Loop: The game will run in a continuous loop, handling user input, updating the positions of the paddles and ball, detecting collisions and scoring, and redrawing the window to provide a smooth and interactive gaming experience.
The Game Mechanics
In our ping pong game, two players control paddles on opposite sides of the screen, and a ball moves between them. The objective is to bounce the ball off the paddles, trying to make it pass the opponent's paddle to score points. The game continues until one player scores a point, and then the ball resets to the center for another round.
Creating the Game Window
The first step is to set up the game window using Pygame. We initialize Pygame and create a window with a width of 800 pixels and a height of 400 pixels, giving us ample space for the ping pong action.
import pygame
# Initialize Pygame
pygame.init()
# Set up the game window
width = 800
height = 400
window = pygame.display.set_mode((width, height))
pygame.display.set_caption("Ping Pong")
The Paddles and Ball
Next, we'll define the paddles and the ball:
# Set up the paddles
paddle_width = 10
paddle_height = 60
paddle_speed = 5
left_paddle_pos = pygame.Rect(50, height // 2 - paddle_height // 2, paddle_width, paddle_height)
right_paddle_pos = pygame.Rect(width - 50 - paddle_width, height // 2 - paddle_height // 2, paddle_width, paddle_height)
# Set up the ball
ball_radius = 10
ball_speed_x = 3
ball_speed_y = 3
ball_pos = pygame.Rect(width // 2 - ball_radius // 2, height // 2 - ball_radius // 2, ball_radius, ball_radius)
ball_direction = random.choice([-1, 1])
The heart of our Ping Pong game lies in setting up the paddles and the ball. These elements are the players' interfaces with the game, making their movements and interactions critical to the gameplay experience.
Paddle Properties
We start by defining the properties of the paddles. Each paddle has a width, a height, and a speed. The paddle_width
and paddle_height
variables determine the dimensions of the paddles, while paddle_speed
controls how fast they can move. These properties collectively shape the behavior of the paddles in the game.
Paddle Positions
The positions of the paddles are defined using pygame.Rect()
objects. The left_paddle_pos
and right_paddle_pos
variables specify the initial positions of the left and right paddles, respectively. The pygame.Rect()
constructor takes four arguments: the x-coordinate
, y-coordinate
, width
, and height
. By positioning the paddles at the appropriate coordinates, we ensure they are ready for action when the game starts.
Paddle Movement: Putting Players in Control
In our Ping Pong game, player interaction is key to the excitement. The ability to control the paddles and strategically maneuver them to hit the ball adds an element of skill and engagement. Let's dive into the code that enables paddle movement and empowers players to take charge of the game.
Controlling the Paddles
We'll implement paddle movement by listening to user input and adjusting the positions of the paddles accordingly. Players can control the left paddle using the 'W' and 'S' keys, while the up and down arrow keys control the right paddle.
# Move the paddles
keys = pygame.key.get_pressed()
if keys[pygame.K_w] and left_paddle_pos.y > 0:
left_paddle_pos.y -= paddle_speed
if keys[pygame.K_s] and left_paddle_pos.y < height - paddle_height:
left_paddle_pos.y += paddle_speed
if keys[pygame.K_UP] and right_paddle_pos.y > 0:
right_paddle_pos.y -= paddle_speed
if keys[pygame.K_DOWN] and right_paddle_pos.y < height - paddle_height:
right_paddle_pos.y += paddle_speed
Understanding Paddle Movement
The code above captures keyboard input using pygame.key.get_pressed()
. This function returns a list of boolean values representing the state of all keys. When a key is pressed, the corresponding value in the list becomes True
.
For the left paddle, if the 'W' key is pressed and the paddle's top edge is above the screen's top boundary, we decrease the y-coordinate of the left_paddle_pos
object to move it up. If the 'S' key is pressed and the paddle's bottom edge is below the screen's bottom boundary, we increase the y-coordinate to move it down.
The same logic applies to the right paddle, where the up and down arrow keys control its movement. The conditions ensure that the paddles remain within the vertical boundaries of the game window.
Ball Properties
Similar to the paddles, the ball has its own properties that influence its behavior. The ball_radiu
s variable defines the size of the ball. Larger values create a larger ball, while smaller values yield a smaller one. The ball_speed_x
and ball_speed_y
variables control the horizontal and vertical speeds of the ball, respectively. These speeds determine how quickly the ball moves in each direction.
Ball Position
The position of the ball is defined using another pygame.Rect()
object. The ball_pos
variable specifies the initial position of the ball on the screen. The ball starts at the center of the game window, thanks to the calculations involving the window's dimensions and the ball's radius.
Ball Direction
Finally, we introduce an element of randomness to the game by determining the initial direction of the ball. The ball_direction
variable randomly chooses either -1 or 1, effectively dictating whether the ball moves to the left or right at the start of the game. This adds an element of unpredictability and keeps players on their toes from the very beginning.
With these foundational elements in place, our game is ready to come to life. As we move forward, we'll delve into the game loop, collision detection, scoring mechanisms, and more, bringing our Ping Pong game to fruition. The careful setup of paddles, ball, and their properties ensures that players will have an engaging and interactive experience as they face off in this classic arcade-style game.
The Game Loop
The heart of the game lies in the game loop. This loop will ensure the smooth execution of the game, continuously updating the positions of the paddles and the ball, and handling user input.
The ball's movement is calculated by adding the ball_speed_x
and ball_speed_y
to its current ball_pos.x
and ball_pos.y
respectively. The ball_speed_x
and ball_speed_y
are the horizontal and vertical speeds of the ball, respectively. By multiplying the ball_speed_x
with ball_direction
, we determine the direction of the ball.
# Game loop
running = True
while running:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Move the paddles
# (Add paddle movement code here)
# Move the ball
ball_pos.x += ball_speed_x * ball_direction
ball_pos.y += ball_speed_y
# Check for collisions and scoring
# (Add collision and scoring code here)
# Clear the window
window.fill(BLACK)
# Draw the paddles and ball
pygame.draw.rect(window, WHITE, left_paddle_pos)
pygame.draw.rect(window, WHITE, right_paddle_pos)
pygame.draw.ellipse(window, WHITE, ball_pos)
# Update the window
pygame.display.update()
# Quit the game
pygame.quit()
The Game Loop Sequence
1. Event Handling: The loop starts by iterating through the events in the event queue using pygame.event.get()
. It checks if any events have occurred, such as the user closing the game window. If the "Quit" event is detected, the running variable is set to False
, causing the loop to terminate and the game to close.
2. Paddle Movement: The code for paddle movement (explained in the previous section) is executed within the loop. This ensures that the paddles respond to player input during each iteration of the loop.
3. Ball Movement: The code for moving the ball (explained earlier) is also executed within the loop. This ensures that the ball's position is updated continuously, simulating its motion across the screen.
4. Collision Detection: Collision detection logic (to be implemented) checks whether the ball collides with the paddles or other game elements. Depending on the collision results, the ball's direction may change.
5. Scoring Logic: The scoring mechanism (to be implemented) determines if a player has scored a point by letting the ball pass their opponent's paddle. If a point is scored, the ball's position is reset, and the game continues.
6. Rendering: The window is cleared and filled with a black background using window.fill(BLACK)
. This prevents the remnants of previous frames from affecting the current frame.
7. Drawing: The paddles and the ball are drawn on the window using pygame.draw.rect() and pygame.draw.ellipse() functions, respectively. This ensures that the visual representation of the game is updated based on the current positions of the game elements.
8. Updating the Window: The window is updated using pygame.display.update(). This step makes the current frame visible to the player, displaying any changes made during this iteration of the loop.
Collision Detection and Scoring
To add interaction to the game, we implement collision detection between the ball and the paddles. When the ball comes into contact with a paddle, its direction is reversed, mimicking a bounce.
We also check for scoring conditions. If the ball goes beyond the left or right boundary of the screen, a point is scored. The ball resets to the center, and the game continues.
if ball_pos.colliderect(left_paddle_pos) or ball_pos.colliderect(right_paddle_pos):
ball_direction *= -1
# Check for collisions with walls
if ball_pos.y <= 0 or ball_pos.y >= height - ball_radius:
ball_speed_y *= -1
# Check for scoring
if ball_pos.x <= 0 or ball_pos.x >= width - ball_radius:
# Reset ball position
ball_pos.x = width // 2 - ball_radius // 2
ball_pos.y = height // 2 - ball_radius // 2
# Reset ball speed
ball_speed_x = 3
ball_speed_y = 3
ball_direction = random.choice([-1, 1])
Conclusion
Congratulations! You've successfully built a simple yet engaging ping pong game using Pygame. This project is just the beginning, and there are many exciting possibilities for adding more features, such as keeping score, implementing different difficulty levels, or creating a two-player mode.
Through this project at OpenGenus, you've explored game development concepts and gained experience working with Pygame. Now, you can use your newfound knowledge to create even more exciting games or explore other creative coding projects.
Remember, the world of coding is vast and full of opportunities. Continue learning, experimenting, and honing your skills to become a master of game development and beyond. Happy coding and enjoy playing your very own ping pong game!