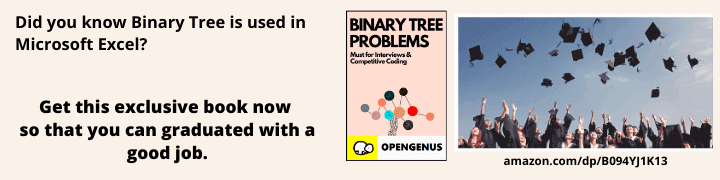
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explored Union() function in Python with multiple examples.
Table of contents:
- Union() function in Python
- Python Union() Function on Two Sets
- Python Union() Function on Three Sets
- Python Set Union Using the | Operator
- Python Set Union() Method on String
- Conclusion
Union() function in Python
The Python Union()
method in sets creates a fresh set that includes all the elements found in the initial set.
The union of two sets refers to a set that combines all the elements from both sets. When sets A and B are united, the resulting set includes every element from A and every element from B, without any duplicates.
-
The 'U' symbol is used to represent the union of sets.
-
The
union()
method returns a set that combines all the elements from the original set and the specified set(s). -
You can provide multiple sets by separating them with commas.
-
The input does not necessarily have to be a set; it can be any iterable object.
-
If an item appears in multiple sets, the resulting set will only include one instance of that item.
-
In Python, the syntax for the set
union()
function is as follows:
- Syntax: set1.union(set2, set3, set4….)
- Parameters: zero or more sets
- If no parameter is provided, the
union()
function returns a copy of the original set. Otherwise, it returns a new set that contains the union of all the specified sets (set1, set2, set3, and so on) along with set1.
Python Union() Function on Two Sets
Here's an example of performing the union() function on two Python sets:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1.union(set2)
print(union_set)
Output:
{1, 2, 3, 4, 5}
In this example, we have two sets set1 and set2. The union() function is called on set1 with set2 as the parameter. It returns a new set union_set that contains all the elements from both set1 and set2. The output shows the resulting set with elements {1, 2, 3, 4, 5}.
Python Union() Function on Three Sets
Here's an example of performing the union() function on three Python sets:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
set3 = {5, 6, 7}
union_set = set1.union(set2, set3)
print(union_set)
Output:
{1, 2, 3, 4, 5, 6, 7}
In this example, we have three sets: set1, set2, and set3. The union() function is called on set1 with set2 and set3 as additional parameters. It returns a new set union_set that contains all the elements from set1, set2, and set3, without any duplicates. The output shows the resulting set with elements {1, 2, 3, 4, 5, 6, 7}.
Python Set Union Using the | Operator
Here's an example of using the | operator to find the union of two Python sets:
set1 = {1, 2, 3}
set2 = {3, 4, 5}
union_set = set1 | set2
print(union_set)
Output:
{1, 2, 3, 4, 5}
In this example, the | operator is used between set1 and set2 to find their union. It performs the same operation as the union() function. The resulting set union_set contains all the elements from both set1 and set2, without any duplicates. The output shows the resulting set with elements {1, 2, 3, 4, 5}.
Python Set Union() Method on String
The union() method is specifically used for sets and cannot be directly applied to strings in Python. However, you can convert strings to sets and then perform the union operation. Here's an example:
string1 = "hello"
string2 = "world"
set1 = set(string1)
set2 = set(string2)
union_set = set1.union(set2)
print(union_set)
Output:
{'d', 'e', 'h', 'l', 'o', 'r', 'w'}
In this example, we convert the strings string1 and string2 into sets using the set() function. Then, we apply the union() method on set1 with set2 as the parameter. The resulting set union_set contains all the unique characters from both strings. The output shows the resulting set with elements {'d', 'e', 'h', 'l', 'o', 'r', 'w'}.
Conclusion
In conclusion, the union()
function in Python is a powerful tool for combining sets and creating a new set that contains all the unique elements from the original set(s). It allows you to easily merge multiple sets together, eliminating any duplicate elements in the process. The union()
function provides flexibility by accepting any iterable object, not just sets. Whether you're working with numbers, strings, or other iterable data, the union()
function can streamline the process of creating a unified set. By leveraging this function, Python developers can efficiently handle data manipulation and set operations, enhancing the flexibility and efficiency of their code.