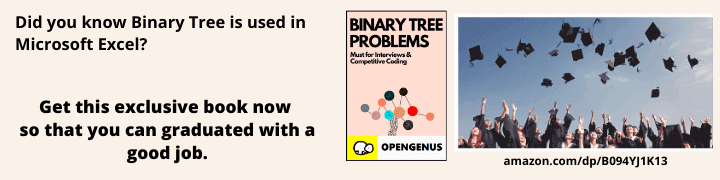
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Pigeonhole sorting is a sorting algorithm that is suitable for sorting lists of elements where the number of elements and the number of possible key values are approximately the same.
Where key here refers to the part of a group of data by which it is sorted, indexed, cross referenced, etc.It is used in quick, heap and many other sorting algorithm.
If we consider number of elements (n) and the length of the range of possible key values (N) are approximately the same. It requires O(n + N) time.
Working of Pigeonhole Sort
-
Find minimum and maximum values in array. Let the minimum and maximum values be āminā and āmaxā respectively. Also find range as max-min+1.
-
Set up an array of initially empty āpigeonholesā the same size as of the range calculated above.
-
Visit each element of the array and then put each element in its pigeonhole. An element arr[i] is put in hole at index arr[i] ā min.
-
Start the loop all over the pigeonhole array in order and put the elements from non- empty holes back into the original array.
In Pigeonhole sort, we need not compare two data to sort them and hence, it is an in-place sorting algorithm
Example
As you can see a lot of blocks are empty, this algorithm requires a lot of space even for a small but widely spread data.
Explaining the steps mentioned above :
When we implement point 3 in working of Pigeonhole Sort we do the following :
Step 1 : 9-1 = 8 => buk[8] = arr[0]
Step 2 : 2-1 = 1 => buk[1] = arr[1]
Step 3 : 3-1 = 2 => buk[2] = arr[2]
Step 4 : 8-1 = 7 => buk[7] = arr[3]
Step 5 : 1-1 = 0 => buk[0] = arr[4]
Step 6 : 6-1 = 5 => buk[5] = arr[5]
Step 7 : 9-1 = 8 => buk[8] = arr[6]
After implementing the array we get :
Difference between Counting and Pigeonhole sort
The ONLY difference between counting and pigeonhole sort is that in the secondary sort we move items twice i.e one on the input array and other in the bucket array. While in first sort we only move the items once.
Implementation
- C++
- Python
C++
#include <bits/stdc++.h>
using namespace std;
/* Sorts the array using pigeonhole algorithm */
void pigeonholeSort(int arr[], int n)
{
// Find minimum and maximum values in arr[]
int min = arr[0], max = arr[0];
for (int i = 1; i < n; i++)
{
if (arr[i] < min)
min = arr[i];
if (arr[i] > max)
max = arr[i];
}
int range = max - min + 1; // Find range
// Create an array of vectors. Size of array
// range. Each vector represents a hole that
// is going to contain matching elements.
vector holes[range];
// Traverse through input array and put every
// element in its respective hole
for (int i = 0; i < n; i++)
holes[arr[i]-min].push_back(arr[i]);
// Traverse through all holes one by one. For
// every hole, take its elements and put in
// array.
int index = 0; // index in sorted array
for (int i = 0; i < range; i++)
{
vector::iterator it;
for (it = holes[i].begin(); it != holes[i].end(); ++it)
arr[index++] = *it;
}
}
// Driver program to test the above function
int main()
{
int arr[] = {9,2,3,8,1,6,9};
int n = sizeof(arr)/sizeof(arr[0]);
pigeonholeSort(arr, n);
printf("Sorted order is : ");
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
return 0;
}
Python
def pigeonhole_sort(a):
# size of range of values in the list
# (ie, number of pigeonholes we need)
my_min = min(a)
my_max = max(a)
size = my_max - my_min + 1
# our list of pigeonholes
holes = [0] * size
# Populate the pigeonholes.
for x in a:
assert type(x) is int, "integers only please"
holes[x - my_min] += 1
# Put the elements back into the array in order.
i = 0
for count in range(size):
while holes[count] > 0:
holes[count] -= 1
a[i] = count + my_min
i += 1
a = [9,2,3,8,1,6,9]
print("Sorted order is : ", end = ' ')
pigeonhole_sort(a)
for i in range(0, len(a)):
print(a[i], end = ' ')
Complexity
- Worst case time complexity:
Ī(n+N)
- Average case time complexity:
Ī(n+N)
- Best case time complexity:
Ī(n+N)
- Space complexity:
Ī(n+N)
where n is the number of elements and N is the range of the input data