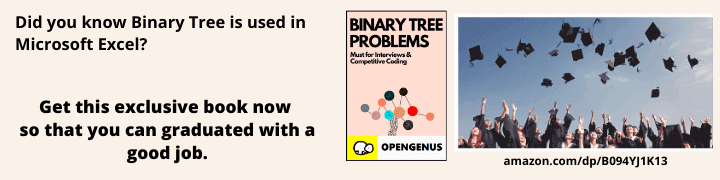
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we are going to learn about the different ways to pause a program in C++. With the help of this method or function, you will be able to pause any C++ program during execution.
A program is used to pause when a user wants to input or read some important data. The Different Pause method provides some time so the user can read data or instructions properly and choose the next action. In other words, the pause () method makes the software more readable and easier to use.
As C++ is the most popular and useful programming language, it provides different methods and functions to pause programs.
Here is a list of some methods or functions that are used to pause the C++ program.
- pause () function
- getc() function
- getchar() function
pause() function
The pause () function is a window method that is used to pause the currently executing program. This method is window-specific and will not work on any other operating system except Windows and DOS.
When a pause () operation gets terminated, it continues to execute the remaining program.
The pause() method pauses the running program’s execution until the user presses the enter key.
How Pause Method Works?
When a user calls the pause method, the entire program code is suspended. Then system () function makes a call to the operating system. It opens a shell and searches for the pause method in the entire library. Then allocate resources and execute the pause () method. Then wait for user input and release memory when the operation is completed. Exit and continue with the remaining program. Finally, display data.
Syntax
system("pause")
Advantage :
pause() method is simple and easy to understnad().
Disadvantage :
Not portable.
This method will not work on Linux or MacOS.
Make system call and allcoacte extra resource.
The pause () method can be used to pause a C++ application, as seen in the example code below.
Program Code
1. //c++ program to pause program by pause() method
2. #include <iostream>
3. #include <cstdlib>
4. using namespace std;
5. int main()
6. {
7. for (int i=0; i<=10; i++)
8. {
9. cout << "i = " << i << endl;
10. if (i == 5) {
11. // Call the pause command
12. cout << "Program Is Paused\n";
13. system("pause");
14. cout << "pause program terminated\n";
15. cout << "Resuming program \n";
16. }
17. }
18. return 0;
19. }
Output :
i = 0
i = 1
i = 2
i = 3
i = 4
i = 5
Program Is Paused
pause program terminated
Resuming program
i = 6
i = 7
i = 8
i = 9
i = 10
Explanation
Here, when i values become equal to 5, the pause method gets called. When the user press enters to terminate the program, resuming program gets executed.
Okay, let's take another example.
In this example, we have a paused program with a specific period (milliseconds).
Program Code
1. #include <ctime>
2. #include <iostream>
3. void pause(int dur);
4. int main()
5. {
6. std::cout<<"Pausing for 5 second... \n";
7. pause(5);
8. std::cout<<". \n";
9. std::cout<<". \n";
10. std::cout<<". \n";
11. std::cout<<". \n";
12. std::cout<<". \n";
13. std::cout<<"DONE\n";
14. return 0;
15. }
16. void pause(int dur)
17. {
18. int temp = time(NULL) + dur;
19. while(temp > time(NULL));
20. }
Output :
Program is pausing for 5 second..
.
.
.
.
.
.
DONE
Explanation
Here, to pause the program for 5 seconds, we have passed the pause method with a time duration of a millisecond. Here, the user doesn’t need to press any enter to continue execution.
getchar ()
The getchar () method, like the pause () method, is used in a pause C++ program.
getchar() is an inbuilt function in C++. which is equivalent to the get(stdin) function. The getchar () method reads the next character from standard inputs and outputs.
When the user presses any key except "ENTER", the system gets paused. and when he presses "ENTER", it accepts the character of the entered line.
This pause will not end. So it is recommended to users read all the data before performing a pause operation.
Syntax :
int getchar(void);
Return Type :
This function doesn’t have any parameters.
It returns an int value.
Program Code
1. //c++ program to pause program using getchar() method.
2. #include <iostream>
3. #include <vector>
4. #include <thread>
5. #include <chrono>
6. using std::cout;
7. using std::cin;
8. using std::endl;
9. using std::vector;
10. using std::copy;
11. using std::this_thread::sleep_for;
12. using namespace std::chrono_literals;
13. //driver code
14. int main()
15. {
16. int flag;
17. cout << "your program is paused!\n";
18. cout << "Press Enter to continue\n";
19. flag = getchar();
20. cout << "\nContinuing \n";
21. //make sleep program 200ms
22. sleep_for(200ms);
23. cout << "..........\n";
24. cout << "..........\n";
27. //this will print after 200ms or sleep period complete
28. cout << "\nProgram Completed!";
29. return EXIT_SUCCESS;
30. }
Output :
your program is paused!
Press Enter to continue
Continuing
..........
..........
Program Completed!
Explanation :
Here, we have passed the getchar() method to pause the program. If the user presses the key "ENTER", it accepts the characters of the entered line. When the sleep period is over, the reaming program starts to execute.
getc()
The getc() is also used to pause a program . This function is present in the standard input-output library. This function belongs to the C library.
This function reads the next character from the stream.
Syntax :
getc(FILE *stream)
It is the FILE object's pointer which identifies the stream type for operation . The stream contains three standard file streams:
- stdin
- stdout
- stderr
Where stdin is used to take input taken from a user's keyboard.
It makes a user wait for a user to continue program execution.
Program Code
1. //c++ program to pause program using getc() method.
2. #include <iostream>
3. #include <vector>
4. #include <thread>
5. #include <chrono>
6. using std::cout;
7. using std::cin;
8. using std::endl;
9. using std::vector;
10. using std::copy;
11. using std::this_thread::sleep_for;
12. using namespace std::chrono_literals;
13. //driver code
14. int main()
15. {
16. int flag;
17. cout << "your program is paused !\n";
18. cout << "Press Enter to continue\n";
19. flag = getc(stdin);
20. cout << "\nContinuing .";
21. //make sleep program 50ms
22. sleep_for(50ms);
23. cout << "..........";
24. //this will print after 50ms or sleep period complete
25. cout << "\nDone";
26. return EXIT_SUCCESS;
27. }
Output :
your program is paused !
Press Enter to continue
Continuing ...........
Done
Explanation
Here , we have passed the get(stdin)as a argument to pause program . which correspond to console input . when user press enter key getc() function accept it and make program pause for entire sleep period .When sleep period get complete it print done message.