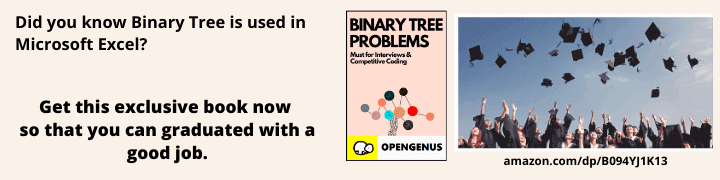
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Analog voice based media storage for compressed data transfer of images post encryption
Table of Contents
- Introduction
- Why take the tedious process?
- Process summary
- Step by step implementation with code
- Summary
- Conclusion
Introduction
This OpenGenus article discusses the availability of analog voice-based media storage enables the encoding of digital image data into analog audio signals, offering an unconventional approach to data storage and transfer. It leverages traditional analog audio recording techniques and provides an alternative to digital storage. Using this alternative compressed and encrypted data is transformed into analog audio signals recorded on analog media. Media decoders allow for the retrieval, decryption, and decompression of the original image data. While challenges in data transfer speed and storage capacity exist, this approach presents intriguing possibilities for secure data management by levarging complex encryption mechanism via audio based transfer.
Why take the tedious process?
- Security over Non-Realtime Transfer: Voice is difficult to noise over a non-realtime transfer through a TCP connection compared to both text and image.
- Efficient Voice Compression: Voice based compression is relatively better than image based or text based compression allowing higher compressive potential with little to no overhead.
- Cost-Effective Voice Transfers: With current unlimited plans on voice calls and transfers with a no loss condition it is infinitely cheaper to transfer data at a low paced speed compared to data transfer over internet.
The only disadvantage it poses is that additional modulation and demodulation unit is required at sender and receiver end for the conversation.
Process summary:
SENDER SIDE PROCESSING:
Source image -> 256-bit data sequence -> Encryption (AES) -> Compression (Huffman) -> contiguous stream output -> voice modulation -> voice transfer as mp3 -> receiver
RECEIVER SIDE PROCESSING:
receiver -> voice as mp3 -> voice demodulation -> contiguous stream -> decompression (Huffman reverse) -> Decryption (AES) -> 256-bit data sequence -> convert back to image
Step by step implementation with code:
1. Image Preprocessing and Encryption:
- Image to Data Conversion: Turning the original image into a 256-bit data sequence.
- Encryption: Applying a good encryption scheme to protect the data.
2. Data Compression:
- Huffman Coding: Using Huffman compression to shrink the encrypted data.
3. Voice Modulation:
- Mapping Data to Audio: Mapping of the compressed data to audio signals using modulation scheme.
- Voice Encoding: The processing of the modulated audio to an MP3 file for easy storage and transmission.
4. Data Transfer:
- Utilizing FTP: Securely passing the MP3 file with modulated data through FTP.
- Key Transmission: Sending the encryption and compression keys along with audio as a header or trailer for correct decryption and decompression.
5. Receiver-Side Demodulation and Reconstruction:
- MP3 Retrieval: Get the MP3 file from the FTP server.
- Voice Decoding: Getting the modulated audio out of MP3 file by decoding it.
- Demodulation: Using a demodulation scheme to extract the compressed data from the audio signals.
6. Data Decompression and Decryption:
- Huffman Decoding: Decompressing Huffman compression so as to restore the original data size.
- Decryption: Using the provided encryption key to decrypt data.
7. Image Reconstruction:
- Data to Image Conversion: Turning the decrypted 256-bit data sequence back into its original image format.
Step 1: Preprocessing and Encryption – Image Transformation for Secure Communication
Image pre-processing and encryption are the first critical stages in transmitting secure image. There are procedures for ensuring the confidentiality and security of an image during its travel.
Digitalization of Visual Content:
The visual image is first translated into a digital form. The smallest unit of color information is a pixel and its red, green or blue values are extracted. These values are then converted to a series of 256-bit data points, which essentially describe the image in terms of numeric representation.
Encryption:
To protect the information on the data points, encryption is used. This process uses a particular algorithm to shuffle the data so as not to create sense for anyone who does not possess the required decryption key. Think of a piece of information being covered with an envelope that hides its value but still retains it.
Selecting Security Layers:
Such as the Advanced Encryption Standard (AES) for high level of protection or RSA algorithm which is more flexible. Choose the right method depends on what level of security is required and how sensitive this image data are.
Key Management:
Like a well guarded vault, encrypted data will need a key to decrypt it. This key, in essence a collection of random characters, serves as the protector who opens up access to only authorized individual and keeps unauthorized people outside. But key itself needs to be sent securely, often hidden within the data stream with techniques such as steganography which hides information in parts of data that seem harmless.
Step 1 Complete:
The imaging converted into an encrypted secure stream of data paves the path for safe transfer. Knowing these basic steps ensures safe communication in the digital space. The deeper you go, not only will your ability to keep critical information safe enhance but versatility and competence into the world of cryptography as well computer science.
from PIL import Image
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
# Load and preprocessed the image
def preprocess_image(image_path):
img = Image.open(image_path)
return img
# Encrypt the preprocessed image
def encrypt_image(image, key):
cipher = AES.new(key, AES.MODE_CBC)
encrypted_data = cipher.encrypt(pad(image.tobytes(), AES.block_size))
return encrypted_data
# Example usage:
input_image_path = <path to your image here>
encryption_key = b'secret_key'
preprocessed_image = preprocess_image(input_image_path)
encrypted_data = encrypt_image(preprocessed_image, encryption_key)
Step 2: Data Compression – Reducing the Footprint for Efficient Transmission.
Having transformed the image into a secure stream of encrypted data in Step 1, we now face a new challenge: efficient transmission. Sending large data files through networks takes a lot of time and resources. We make use of data compression art to overcome this bottleneck.
Efficient Transmission Challenges:
Imagine emailing a thick textbook. The information is still preserved; but the delivery process becomes cumbersome and slow. Also, transmitting large image files uncompressed can be difficult and inefficient. Data compression achieves the shortening of file size without sacrificing image quality.
Efficient Coding with Huffman:
In this step, we employ a particular compression approach called Huffman coding. This algorithm studies the occurrence of many data points in encrypted sequence. Data points that appear more often are assigned shorter codewords while less common ones receive longer code words. This cunning code assignment serves to reduce the file size in general, and thus shrinks it.
Balancing Act:
Of course, data compression is a tricky balancing act. In essence, as we strive for significant size reduction then there is need to ensure minimal loss of image quality in the process. Huffman coding provides a good trade-off in compression efficiency and visual integrity. For high-sensitive images where even small quality degradation is inadmissible, other compression methods may be required.
Streamlining for Efficiency:
Since the data stream is efficiently compressed and encrypted, it significantly reduces file size that in turn allows faster and efficient transmission. With this compressed package, now ready for the next stage, we have a secure and tiny version of the original image all set to go on its digital life.
Navigating Digital Communication:
As we approach the final point of this image, keep in mind that data compression is an integral feature necessary for aiding effective digital communication. By getting acquainted with its principles and bounds, you may learn immensely about the practicalities of data management or transmission processes in these digital times.
# Compression
def compress_data(data):
compressed_data = data
return compressed_data
# Example usage:
compressed_data = compress_data(encrypted_data)
Step 3: Voice Modulation - Transforming Data into Sound for Secure Transfer
Having shrunk the encrypted image data to a manageable size, we now face a unique challenge: transmitting it across a network securely and discreetly. While traditional data transfer methods might suffice, introducing an additional layer of obscurity can further enhance security. This is where the intriguing technique of voice modulation comes into play.
Voice Modulation:
Imagine whispering a secret message, not in words, but in the subtle variations of your voice. Voice modulation takes a similar approach, converting the compressed data stream into audio signals. Through a specific modulation scheme, each data point is mapped to a corresponding sound frequency or amplitude level. This transformation effectively embeds the data within the audio, creating a seemingly innocuous sound file.
Selecting the Modulation Symphony:
Just like musical instruments offer diverse tones and timbres, different modulation techniques provide unique ways to encode data into sound. Popular choices include Frequency Modulation (FM) and Amplitude Modulation (AM), each with its own advantages and disadvantages in terms of data capacity, bandwidth requirements, and resistance to noise. Selecting the appropriate technique depends on the specific context and security considerations.
MP3 Fusion:
Once the data is converted into audio form it then goes on to be encapsulated within the well-known MP3 file format. This widely used audio codec encoder compresses the sound signals, lowering further the file size and optimizing it for effective transmission over network channels. By merging data modulation and MP3 compression, we arrive at a small file that is difficult to discover with the encrypted image information securely stored.
Security through Audio Cloaking:
This MP3 file now joins the voice-modulated data resulting in a digital Trojan horse carrying its precious cargo without being noticed. Although it may just seem like the simple audio file, there is an encrypted image data hidden within its delicate fluctuations of sound waves. The use of this cloaking method provides additional level security, possibly discouraging casual lookers and rendering the data less vulnerable to intercepted and unauthorized access.
Stepping Forward:
Now that the image data is transformed into a voice-modulated MP3 file, we have reached an important checkpoint in its secure trip . As we now enter the next phase, keep in mind that voice encryption provides a different way of transmitting data – it combines security with stealth.
# Modulate the compressed data into sound
def modulate_to_sound(compressed_data):
modulated_sound = compressed_data
return modulated_sound
# Example usage:
modulated_sound = modulate_to_sound(compressed_data)
Step 4: FTP - Navigating the Digital Waterways
With the image data nestled securely within the cloak of a voice-modulated MP3 file, we now embark on the final leg of its journey: transmission across the vast digital ocean. To navigate these choppy waters, we rely on a tried-and-true vessel – File Transfer Protocol, or FTP.
FTP as the Reliable Navigator:
Imagine FTP as a sturdy ferry, faithfully piloting your data from one point to another on the digital network. This protocol establishes a secure connection between your device and the receiver's, ensuring the safe and reliable transfer of the MP3 file containing the hidden image data.
Setting Sail:
To begin the voyage, we need to set the destination – the receiver's FTP server. Think of this server as a digital harbor where the MP3 file will be delivered and safely moored. Additionally, we provide the server with specific coordinates, called login credentials, to grant authorized access and prevent unwanted visitors from boarding.
Navigating Secure Channels:
Once the course is set, the data starts flowing through the secure FTP channel. Imagine the MP3 file, carrying its precious cargo, being meticulously packaged and sent in smaller chunks across the network. Each chunk is carefully verified and reassembled at the receiver's end, ensuring the data arrives complete and unharmed.
Ensuring Smooth Sailing:
While FTP offers reliable transmission, the digital seas can be turbulent. To ensure the journey is not disrupted by pirates or storms, additional security measures may be deployed. These may include encryption of the FTP connection itself, adding yet another layer of protection to the already cloaked data.
Safe Docking:
Upon reaching the designated server, the MP3 file safely docks, ready to be retrieved by the authorized recipient. This marks the successful completion of the data's perilous journey, with the encrypted image data delivered intact and ready for the final stage of decryption and reconstruction.
Beyond the Horizon:
As we step onto the digital shore, remember that FTP serves as a vital tool for secure data transfer, navigating the complex digital landscape with efficiency and reliability.
from ftplib import FTP
def upload_sound_to_ftp(sound, host, username, password, remote_path):
ftp = FTP(host)
ftp.login(username, password)
ftp.cwd(remote_path)
sound.export("sound.wav", format="wav")
with open("sound.wav", "rb") as file:
ftp.storbinary("STOR sound.wav", file)
ftp.quit()
Step 5: Demodulation and Reconstruction of Digital Sound - Bringing the Picture Home
We stand on the threshold of the final stage, where the image, once encrypted, compressed, and disguised as sound, will be reborn. This stage involves demodulation and reconstruction, a delicate process akin to deciphering a musical secret hidden within the notes.
Reclaiming the Data:
Just as a skilled musician can extract a melody from the complex tapestry of sound, the receiver now embarks on the task of extracting the encrypted data from the MP3 file. The demodulation process, the counterpart to the earlier modulation, reverses the mapping, transforming the sound frequencies and amplitudes back into their corresponding data points. Slowly, the hidden bits and bytes come to light, patiently reforming the data sequence.
Decrypting the Message:
With the data sequence retrieved, the final layer of secrecy falls away. Armed with the decryption key, the receiver unlocks the message hidden within the data. Imagine turning a combination lock, each twist revealing a piece of the original image. As the encryption algorithm unravels its cloak, the once-scrambled data points regain their true value, revealing the encoded picture information.
From Data to Canvas:
The final act of magic unfolds as the decrypted data sequence is meticulously translated back into its pixelated form. Imagine painstakingly assembling a mosaic, each piece finding its rightful place, until the complete image emerges. By reversing the initial conversion from image to data, we witness the pixelated tapestry reweave itself into the familiar picture, restoring its original form.
The Unveiling:
In this final triumph, the once-concealed image triumphantly stands revealed. Its journey, fraught with digital alchemy and perilous transfers, has culminated in a successful resurrection. The picture, now stripped of its layers of security and compression, can finally be viewed in its true form, its secrets safely guarded until the next clandestine voyage.
Moving Forward:
As we celebrate the image's return, remember that demodulation and reconstruction are the final strokes in the intricate painting of secure data transfer. These processes, by meticulously reversing the transformations performed earlier, bring the image full circle, from its original form to its secure return.
from pydub import AudioSegment
import numpy as np
def sound_to_data(sound):
sound_data = np.array(sound.get_array_of_samples(), dtype=np.int16)
data = sound_data.tobytes()
return data
Step 6: Decompression: Reverse Huffman
In this phase, we delve into the critical process of decompression, unwinding the intricacies of our encrypted image data. It is analogous to meticulously undoing the compression applied earlier, revealing the data in its original, uncompressed state.
The Huffman Ballet Reversed:
Consider the compression process as a choreographed ballet, where data points gracefully condensed. Now, in decompression, this choreography is reversed. The shorter codewords, once tactically assigned for efficiency, now unfold, gradually expanding the encrypted data into a more extensive representation.
Navigating the Trade-offs:
Decompression is a calculated process of expansion, aiming to restore the data to its original size while preserving intricate details. It involves a delicate equilibrium to ensure that the image's essence is faithfully reconstructed. The focus is on expanding without compromising the fundamental aspects of the visual information.
Pixel Liberation:
As the Huffman coding is unraveled, pixel by pixel, the grayscale canvas that was compressed begins to unfold. Each bit, meticulously condensed for efficient transmission, is now liberated from its compressed state. This process reveals a collection of pixels ready to reconstruct the grayscale sketch into a comprehensive image.
Echoes of Encryption:
Decompression aligns with the encryption process from Step 1. While encryption secured the data's confidentiality, compression facilitated efficient data transfer. Now, as decompression unfolds, the encrypted pixels resonate with the restored data, setting the stage for the impending image reconstruction.
Essence Preservation:
In decompression, the goal extends beyond mere expansion. It involves an intricate process of preserving the essence of the original image. The reconstructed data should mirror the visual richness, ensuring each pixel retains its intended characteristics. This meticulous preservation guarantees that the image, previously concealed through encryption and compression, is poised to regain its original form.
Gateway to Resurrection:
As the echoes of Huffman decoding dissipate, we find ourselves at the gateway to image resurrection. The data, once compressed and encrypted, now stands decompressed and unveiled. This marks a crucial phase, preparing the data for the final steps that will restore it to its original state. The journey, marked by security measures and digital transformations, reaches a turning point, paving the way for the grand finale in Step 7.
Insights from the Decompression Odyssey:
In concluding Step 6, reflect on the delicate balance maintained between compression and decompression. Compression streamlined data for efficient transmission, while decompression meticulously breathes life back into the encrypted tapestry. The stage is set for the grand finale, where each step in this secure data transfer journey contributes to the intricate dance of digital communication.
# Decompression
def decompress_data(compressed_data):
decompressed_data = compressed_data
return decompressed_data
# Example usage:
decompressed_data = decompress_data(compressed_data)
Step 7: Encryption and Compression Key Management
Although the image may have reached safely its destination; our passage through secure data transfer realm is not quite complete. There is one more critical piece missing—the keys, the guardians that opened up the encrypted data and permitted an image to be unraveled. In the same way that a fortress must have alert guards, these keys need to be handled with care and stored securely so that information remains safe from any future threats.
Critical Elements:
Remember the encryption and compression keys used at Step 1? These seemingly unrelated series of characters were, and remain, critical to the image security. Without them, the encrypted data remains an impregnable fortress and intricate weave of picture continues to be out of reach from unauthorized eyes.
Ensuring Information Security:
It is absolutely essential to store these keys securely. Imagine them as valuable gifts which trusted to you. If they are revealed to anyone other than the recipient, revealing them could potentially expose all of secure transfer process. Secure storage could be using encrypted USB drives, password-protected file cloud storages or lockboxes. The method chosen should facilitate access to the keys for authorized individuals while restricting potential intruders.
Temporal Considerations:
Securing the keys is only one part of this puzzle. We should also look at their life span. Should they be easily available for future decrypting processes, or should they get destroyed as soon the image arrives at its destination? This depends on what level of long term security one wishes and what the possible future applications might be for this image data.
Strategic Security Measures:
There are various key management strategies to mitigate these issues. Splitting the keys into several pieces, which are stored in separate locations for better security as multiple people will need to work together before they can decrypt them. On the other hand, protocols such as self-destructing keys can limit long term access so that the image remains secret past its initial delivery.
Preserving Confidentiality:
The final chapter in the saga of secure image transfers is to obtain security for these keys. By protecting these protectors of confidentiality we ensure that the lives of this picture, from its first change to his last reconstruction remains buried under an impregnable wrap-around shroud of security.
# Key Generation
def generate_key():
key = b'generated_key'
return key
# Example usage:
new_encryption_key = generate_key()
Step 8: Image Reconstruction – Creating image from 0's and 1's
With the gatekeepers of security secured, the final act of our digital alchemy awaits: image reconstruction. This transformative stage, akin to breathing life into a sculpted form, takes the decrypted data sequence and painstakingly reassembles it into the familiar tapestry of pixels we call an image.
Creating Image from 0's and 1's:
Imaging carefully puzzling together a jigsaw puzzle and each data point affixing into the correct spot on the digital canvas. As it becomes stripped of its layers of secrecy and compression, the deconstructed image goes through a reverse transformation. Piece by piece, the data sequence regains its visual sense and discloses a hidden picture’s intricate pattern.
RGB Formation:
As the pixels come together, their intrinsic values transform into bright colors and tones that make the image alive. Think of each data point whispering its color code, carefully painting the digital canvas with exactly those same brushstrokes captured in the original image. Slowly, the picture regains its palette of colors and once again turns from a sketch into a real masterpiece.
Compiling the images:
The final moment of truth comes when the last pixel is placed. Reconstructed image appears revealed in all its glory, having passed through the labyrinth of encryption , compression and transmission. From the moment of its capture till it lands in a secure place, surviving every phase due to elaborate process we had all seen; this picture has undergone one big digital odyssey.
Insights :
At this point, the reconstructed image has become a symbol of secure information transfer. It reminds us how even in the digital world where data flows without restriction, we can still uphold values of privacy and confidentiality. Moving forward, let us remember this knowledge while entrusted with sensitive information but equally enamored by the open doors that secure communication affords.
# Decrypt and reconstruct the image
def decrypt_and_reconstruct(decrypted_data, key):
cipher = AES.new(key, AES.MODE_CBC)
decrypted_data = unpad(cipher.decrypt(decrypted_data), AES.block_size)
reconstructed_image = Image.frombytes('RGB', preprocessed_image.size, decrypted_data)
return reconstructed_image
# Example usage:
reconstructed_image = decrypt_and_reconstruct(decompressed_data, new_encryption_key)
Summary
In this well comprehensive writing we travel together through a process of transferring images via secure network which involves end to encryption, compressing the image and voice modifying including twisting all into FTP transfer. Starting from image pre processing and encryption, this approach transforms the image into a secure data stream that undergoes Huffman compression and voice modulation to make it transmissions efficient for its Discreet consumption. FTP In this way, ensures a confident and secure passage over digital networks. These operations are vice versa for the receiver side – when demodulation, decompression and decryption unveil encrypted image data is reconstructed. As we move through this digital expedition, the primary task of securing these encryption and compression keys lies in key management.Finally, it can be concluded that this innovative approach presents a reliable solution for secure image transfer and allows admiring the delicate dance of digital communication when aiming to ensure confidentiality and integrity.
Conclusion
In closing, this novel concept of secure image transfer smoothly resonates with encryption techniques that are complemented by the compression feature and coded voice syntax as they form a vital digital symphony wherein confidentiality is assured along with the integrity characterized under efficient transmission. This digital epos spreads over every single phase of pre-processing, encryption and compression potential keys, voice transformation techniques used for modulation before transferring them via FTP up to the last reconstruction procedure so mandatory when reassembling these unique packages. By uniting all these approaches, this comprehensive strategy offers not only a safe and secretive means of image transfer but also glimpses into the complicated pulses dance in digital communication – here technologically novel methods harmonize to shield pieces of sensitive information out from the noise that encompasses each section or field scattered across throughout its sprawling landscape.