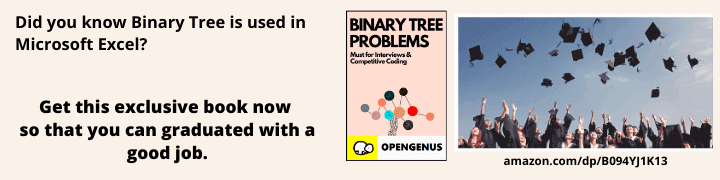
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of content
- Introduction
- The Difference
- Types of Smart Pointers
- Time and Space Complexity
- Conclusion
Key Takeaways
- Raw pointers can lead to memory leaks and dangling pointers, while smart pointers automate memory management, offering safer and more reliable code.
- Deep dive into types of Smart pointers : unique_ptr, shared_ptr, weak_ptr
- The role of smart pointers in automatically managing dynamically allocated resources such as memory, and how they help prevent common issues like memory leaks and dangling pointers.
Introduction
Smart pointers are essential tools in modern C++ programming for managing memory and resources effectively. They ensure that we don't encounter issues like memory leaks or resource leaks by automatically handling the cleanup when objects are no longer needed.
The concept of RAII, or "Resource Acquisition Is Initialization," is closely tied to smart pointers. It emphasizes the idea of initializing resources along with object creation, which ensures that resources are properly managed throughout their lifecycle.
Using smart pointers from the Standard Library (found in the
RAII, or "Resource Acquisition Is Initialization," is a handy rule in C++ that says: when you create an object, make sure it also acquires any resources it needs. Then, when the object goes out of scope and gets destroyed, it automatically releases those resources. This ensures that resources are managed safely and efficiently without causing memory leaks or other problems. Smart pointers help implement RAII by automatically handling resource cleanup when objects are destroyed.
The Difference
RAW POINTERS | SMART POINTERS | |
---|---|---|
Raw pointers in C++ are basic memory address holders without built-in memory management. | Smart pointers are a part of the C++ Standard Library. | |
Memory allocation and deallocation are done manually using new and delete operators respectively. | Manages memory automatically using the RAII (Resource Acquisition Is Initialization) idiom. | |
Can lead to errors such as null pointer dereferencing, memory leaks, and dangling pointers due to manual memory management. | More safer, as they offer null-checking, automatic deallocation, and ownership management, reducing common pointer-related errors. | |
More lightweight in terms of overhead as they only store a plain memory address. | Smart pointers in C++ involve some extra memory management overhead, but their safety and convenience make them worth using. | |
Provides low-level control over memory management, allowing for unconventional use cases or optimizations, but requires careful handling. | Offers a higher level of abstraction with limited flexibility compared to raw pointers but ensures safer and more manageable memory handling in most scenarios. |
Types of Smart Pointers
C++ provides three main types of smart pointers:
Unique_ptr:
It was introduced in C++11 which provides exclusive ownership of the dynamically allocated object. This means that at any given time, only one std::unique_ptr instance can own the resource. When the std::unique_ptr goes out of scope or is explicitly reset, it automatically deallocates the associated memory.
Key Features:
-
Exclusive Ownership: It ensures exclusive ownership of a resource to prevent accidental sharing or deletion.
-
Automatic Deallocation: It automatically deallocates memory when it goes out of scope.
-
Move Semantics: It supports move semantics, preventing unintended resource sharing by enabling transfer of ownership between objects but disallowing copying.
#include <iostream>
#include <memory>
using namespace std;
int main() {
// Creating a unique_ptr to manage an integer dynamically allocated
unique_ptr<int> ptr(new int);
*ptr = 10;
// Printing the value
cout << "Value: " << *ptr << std::endl;
// unique_ptr automatically deallocates memory when it goes out of scope
return 0;
}
Shared_ptr:
Shared_ptr serves as a refined pointer in C++, offering shared ownership. This special capability allows many smart pointers to work together to control one object that was allocated dynamically. It keeps track of how many pointers are using the shared resource. When this count reaches zero, meaning no pointers are using the resource anymore, the memory is automatically deallocated. This mechanism ensures efficient memory management, preventing memory leaks and facilitating robust resource sharing within C++ programs.
Key Features:
-
Shared ownership: Multiple parts of a program can share and manage the same resource safely.
-
Automatic memory management: Memory is deallocated automatically when the last shared_ptr owning the resource goes out of scope or is reset.
-
Thread-safe reference counting: The reference counting mechanism is thread-safe for multithreaded environments.
#include <memory>
#include <iostream>
using namespace std;
int main() {
unique_ptr<int> ptr = make_unique<int>();
*ptr = 10;
cout << *ptr << std::endl;
// Ownership transfer
unique_ptr<int> ptr2 = move(ptr);
if (ptr == nullptr) {
cout << "ptr is null after move." << std::endl;
}
return 0; // ptr2 goes out of scope here, memory is deallocated
}
Weak_ptr:
It observes an object controlled by a shared_ptr without affecting its reference count. It's useful for breaking cyclic dependencies and observing shared objects without prolonging their lifespan, promoting better memory management in C++ programs.
Key Features:
-
Non-owning Reference: Unlike shared_ptr, It provides a non-owning reference to a dynamically allocated object, allowing access without affecting its lifetime or reference count.
-
Prevention of Circular Dependencies: It breaks cyclic dependencies among shared_ptr instances, preventing memory leaks and ensuring proper memory management.
-
Automatic Expiration Handling: weak_ptr's lock() method offers safe access to objects by returning a shared_ptr if the object exists, or an empty shared_ptr to prevent dangling pointers.
A dangling pointer is a pointer that still holds an address of a memory location after that memory has been deallocated. Accessing or dereferencing this pointer can lead to unpredictable behavior or crashes.
#include <iostream>
#include <memory>
using namespace std;
int main() {
shared_ptr<int> sharedPtr = make_shared<int>(10);
weak_ptr<int> weakPtr = sharedPtr;
// Check if the object still exists before accessing it
if (auto ptr = weakPtr.lock()) {
cout << "Value: " << *ptr << endl; // Accessing the object's value
} else {
cout << "Object no longer exists" << endl;
}
// Resetting the shared pointer
sharedPtr.reset();
// Trying to access the object again
if (auto ptr = weakPtr.lock()) {
cout << "Value: " << *ptr << endl; // This won't execute as the object has been deleted
} else {
cout << "Object no longer exists" << endl;
}
return 0;
}
Time and Space Complexity
Unique_ptr
Time Complexity: When using std::unique_ptr, basic operations like creating, deleting, accessing, moving ownership, and resetting the pointer take the same amount of time, no matter how big the data structure is. This constant time is represented by O(1). So, whether you have a small or large data structure, these operations happen in a fixed and predictable time.
Space Complexity: Same as raw pointers, O(1). The std::unique_ptr object itself typically requires minimal space overhead.
The space complexity of raw pointers in C++ is typically constant and depends on the architecture of the system.
Shared_ptr
Time Complexity: The time complexity of shared_ptr in C++ is typically O(1) for various operations like construction, destruction, dereferencing, creating new shared_ptr instances, and incrementing/decrementing reference counts. However, in multithreaded scenarios, synchronization may add extra overhead. Overall, shared_ptr efficiently handles pointer management and reference counting, making it widely favored for dynamic resource management in C++ programs.
Space Complexity: O(1) for the shared_ptr object itself. However, it also keeps a control block containing the reference count and other management data, which adds some overhead. Additionally, each shared_ptr holds a pointer to the managed object, which has its own space complexity.
Weak_ptr
Time Complexity: Operations like construction, destruction, and lock/unlock operations are O(1), but accessing the underlying shared pointer may have a complexity equivalent to accessing a raw pointer.
Space Complexity: Similar to shared_ptr, O(1) for the std::weak_ptr object itself and additional space for the control block.
Conclusion
Smart pointers in C++ represent a significant advancement in memory management, providing automated resource cleanup, clear ownership tracking, and adherence to the RAII principle. They enhance code safety, readability, and developer productivity by reducing the occurrence of memory-related bugs and minimizing manual memory management overhead. By choosing the appropriate smart pointer type for the task at hand and following best practices, developers can write safer, more efficient code with minimal effort, leading to improved software quality and reliability. Overall, smart pointers revolutionize memory management in C++, enabling developers to focus on solving higher-level problems while ensuring robust and deterministic resource management.