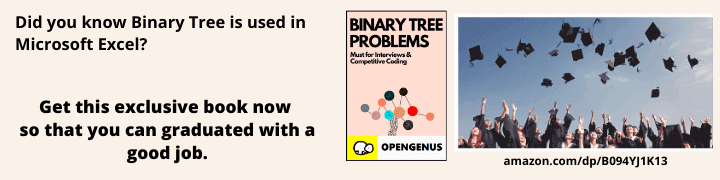
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Key Takeaways (Lazy initialization in C++)
- Lazy initialization is a technique where the creation of an object is deferred until it is actually needed. This can improve performance by avoiding the creation of objects that are never used.
- std::unique_ptr is the preferred method for lazy initialization. It is safe, efficient, and thread-safe. It also ensures automatic destruction of the object when it is no longer needed.
- std::optional is a good choice for lazy initialization when a nullable state is required or when the object is not thread-safe. It allows safe initialization in a single thread and later usage in other threads.
- Raw pointers should be used for lazy initialization only when absolutely necessary. They require manual memory management and lack inherent thread safety, so caution must be exercised when using them.
Lazy initialization is a powerful technique in programming that defers the creation of an object until it's actually needed. This optimization method conserves resources and enhances application performance. In C++, lazy initialization can be implemented using different approaches. In this article, we'll explore three methods: utilizing std::unique_ptr
, std::optional
, and raw pointers.
Table of Contents:
- Understanding Lazy Initialization
- Implementing Lazy Initialization with
std::unique_ptr
- Implementing Lazy Initialization with
std::optional
- Implementing Lazy Initialization with Raw Pointers
- Conclusion
Understanding Lazy Initialization:
Lazy initialization ensures that resource-intensive objects are created only when they are required, thus optimizing resource usage and improving efficiency. Consider a scenario where a ConfigurationManager object holds critical configuration data. Instead of creating it immediately upon program startup, lazy initialization allows us to instantiate it only when the configuration data is needed.
Implementing Lazy Initialization with std::unique_ptr
:
std::unique_ptr
is a smart pointer provided by the C++ Standard Library. It manages the memory of another object and ensures proper deallocation when the object is no longer needed. Here's how lazy initialization using std::unique_ptr
can be implemented:
#include <iostream>
#include <memory>
class ConfigurationManager {
public:
void LoadConfig() {
std::cout << "Loading configuration..." << std::endl;
// Simulate loading configuration data
}
void UseConfig() {
std::cout << "Using configuration..." << std::endl;
// Logic to use configuration data
}
};
class LazyConfigManager {
public:
static ConfigurationManager& GetInstance() {
static std::unique_ptr<ConfigurationManager> instance = nullptr;
if (!instance) {
instance = std::make_unique<ConfigurationManager>();
instance->LoadConfig(); // Lazy initialization
}
return *instance;
}
};
int main() {
std::cout << "Lazy Initialization Example with std::unique_ptr" << std::endl;
LazyConfigManager::GetInstance().UseConfig(); // Lazy initialization occurs here
return 0;
}
Expected Output:
Lazy Initialization Example with std::unique_ptr
Loading configuration...
Using configuration...
Code Explanation:
std::unique_ptr
: A smart pointer that owns a dynamically allocated object and ensures automatic cleanup when the pointer goes out of scope.std::make_unique<ConfigurationManager>()
: Creates a new instance ofConfigurationManager
usingstd::unique_ptr
.LazyConfigManager::GetInstance()
: Static function responsible for creating and returning theConfigurationManager
instance. It uses a staticstd::unique_ptr
to ensure only one instance is created.
Advantages:
- Automatic Cleanup: Ensures automatic destruction of the object, preventing memory leaks.
- Exception Safety: Provides exception safety; handles cleanup even in the presence of exceptions.
- Readability and Simplicity: Code using
std::unique_ptr
is concise, readable, and easy to understand. - Thread Safety (with Synchronization): Can be made thread-safe with additional synchronization mechanisms, ensuring safe initialization in multithreaded environments.
Disadvantages:
- No Nullable State:
std::unique_ptr
always owns an object, making it non-nullable. This might not be suitable for cases where a null state is necessary.
Implementing Lazy Initialization with std::optional
:
std::optional
is introduced in C++17 and provides a way to represent optional objects. It handles the object's initialization and destruction automatically. Here's how lazy initialization using std::optional
can be done:
#include <iostream>
#include <optional>
class ConfigurationManager {
public:
void LoadConfig() {
std::cout << "Loading configuration..." << std::endl;
// Simulate loading configuration data
}
void UseConfig() {
std::cout << "Using configuration..." << std::endl;
// Logic to use configuration data
}
};
class LazyConfigManager {
private:
std::optional<ConfigurationManager> instance;
public:
ConfigurationManager& GetInstance() {
if (!instance.has_value()) {
instance.emplace();
instance->LoadConfig(); // Lazy initialization
}
return *instance;
}
};
int main() {
std::cout << "Lazy Initialization Example with std::optional" << std::endl;
LazyConfigManager manager;
manager.GetInstance().UseConfig(); // Lazy initialization occurs here
return 0;
}
Expected Output:
Lazy Initialization Example with std::optional
Loading configuration...
Using configuration...
Code Explanation:
std::optional
: Introduced in C++17,std::optional
provides a way to represent optional objects. It handles the object's initialization and destruction automatically.instance.emplace()
: Constructs the object in-place within thestd::optional
.LazyConfigManager::GetInstance()
: Function responsible for creating and returning theConfigurationManager
instance. It usesstd::optional
to manage the object's lifecycle.
Advantages:
- Automatic Cleanup:
std::optional
automatically manages the object's lifecycle, ensuring proper destruction. - Nullable State: Allows for a nullable state, providing clear semantics for optional objects.
Disadvantages:
- Availability (Before C++17):
std::optional
is available in C++17 and later versions, limiting its use in older projects or environments. - Overhead: Might have slight overhead due to additional state tracking compared to raw pointers.
Implementing Lazy Initialization with Raw Pointers:
Lazy initialization using raw pointers involves manual memory management. Here's how it can be implemented:
#include <iostream>
class ConfigurationManager {
public:
void LoadConfig() {
std::cout << "Loading configuration..." << std::endl;
// Simulate loading configuration data
}
void UseConfig() {
std::cout << "Using configuration..." << std::endl;
// Logic to use configuration data
}
};
class LazyConfigManager {
private:
ConfigurationManager* instance = nullptr;
public:
ConfigurationManager& GetInstance() {
if (!instance) {
instance = new ConfigurationManager();
instance->LoadConfig(); // Lazy initialization
}
return *instance;
}
~LazyConfigManager() {
delete instance;
}
};
int main() {
std::cout << "Lazy Initialization Example with Raw Pointers" << std::endl;
LazyConfigManager manager;
manager.GetInstance().UseConfig(); // Lazy initialization occurs here
return 0;
}
Expected Output:
Lazy Initialization Example with Raw Pointers
Loading configuration...
Using configuration...
Code Explanation:
Raw Pointers: Pointers that directly store the memory address of another object.
new ConfigurationManager()
: Manually allocates memory for a newConfigurationManager
object.LazyConfigManager::~LazyConfigManager()
: Destructor responsible for cleaning up the allocated memory.
Advantages:
- Simplicity: Simple and straightforward implementation, especially for developers accustomed to manual memory management.
- Control: Provides fine-grained control over object creation and destruction.
Disadvantages:
- Manual Cleanup: Requires manual memory management, leading to a risk of memory leaks if not handled correctly.
- Lack of Nullable State: Raw pointers do not inherently provide a nullable state, making it difficult to distinguish between uninitialized and initialized states.
- Exception Safety: Lacks exception safety; managing exceptions during initialization can be error-prone.
Conclusion
Lazy initialization is a crucial optimization technique in C++ that conserves resources and enhances application performance. Among the various methods available, each has its own strengths and use cases.
std::unique_ptr
: This is the preferred method for lazy initialization in C++. It is safe, efficient, and thread-safe.std::unique_ptr
ensures automatic destruction of the object when it is no longer needed. It can also be made thread-safe with additional synchronization mechanisms.std::optional
: This is a good choice for lazy initialization when a nullable state is required. It is also suitable for lazy initialization of objects that are not thread-safe.std::optional
allows safe initialization in a single thread and later usage in other threads.- Raw Pointers: Raw pointers should be used for lazy initialization only when absolutely necessary. They require manual memory management and lack inherent thread safety. As a result, caution must be exercised when using raw pointers for lazy initialization.
By carefully considering the specific requirements of your application and understanding the advantages and limitations of each method, you can choose the most suitable approach for implementing lazy initialization in your C++ programs. Employing the right technique ensures efficient resource management, improved performance, and enhanced code readability.