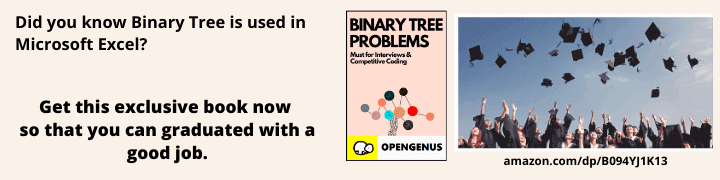
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of content
- Depth Introduction
- Key Features
- Various Operations
- Insertion
- Accessing
- Deletion
- Menu Driven Code
- Complexity Analysis
- Conclusion
Depth Introduction
The vector of arrays in C++ is an excellent tool for efficiently managing fixed-size arrays within a vector. This approach is particularly advantageous for organizing grouped data where each array shares a uniform size. In this structure, each element in the vector is an array of a consistent size. This method is commonly employed when there's a necessity for a collection of arrays with identical sizes.
A vector of arrays is a data structure in C++ that merges the adaptability of a standard vector (from the Standard Template Library) with the robustness of arrays."
Key Takeaways: Vector of Arrays
- Fixed sized array within the vector
- Sequential Access
- Grouped Data Managing
- Operation Explored: Insertion, Deletion, Accessing
Key Features
Grouped Data Management:
- It is especially useful when dealing with grouped data, where each group can have different data with same sizes.
Sequential Access:
- Elements in the vector can be accessed sequentially, similar to accessing elements in a standard array.
Various Operations
In this article, we will explore various operations and experiments that can be performed on arrays of vectors, as detailed below:
Insertion
To simplify the process of placing arrays into a vector, we first create the arrays and then insert the complete array into the vector. This approach streamlines our task and enhances organizational efficiency.
#include<bits/stdc++.h>
using namespace std;
int main(){
//Declaration of vector of array
vector<array<int, 4>> vectorofarray;
//Above 4 define the size of arrays which will be inserted to vector
//creating arrays
array <int, 4> array1 = {1,2,3,4};
array <int, 4> array2 ={5,6,7,8};
//inserting arrays into vector
vectorofarray.push_back(array1);
vectorofarray.push_back(array2);
}
Accessing
To access the elements in a vector of arrays, we can use nested loops. The outer loop goes through each array inside the vector, and the inner loop iterates through the elements of each array. Here's how you can do it:
// Iterate through each array in the vector
for (const auto& arr : vectorofarray) {
// Iterate through each element in the current array
for (const int& element : arr) {
// Print the element followed by a space
cout << element << " ";
}
//Move to next line
cout <<"\n";
}
/*
Output:
1 2 3 4
5 6 7 8
*/
Deletion
In C++, if you have a list of arrays stored in a vector and you want to remove a specific array at a particular position, you can do it using the erase() function.
if we want to delete the array located at index i in a particular, we can use vector_name.erase(vector_name.begin() + i). This will remove the array at index i from the vector. Take a look at it in the code below:
cout<<"\nBefore Deletion\n";
for (const auto& arr : vectorofarray) {
for (const int& element : arr) {
cout << element << " ";
}
cout <<"\n";
}
//Deleting second array from vector
vectorofarray.erase(vectorofarray.begin()+1);
cout <<"\nAfter Deletion\n";
for (const auto& arr : vectorofarray) {
for (const int& element : arr) {
cout << element << " ";
}
cout <<"\n";
}
/*
Output:
Before Deletion
1 2 3 4
5 6 7 8
After Deletion
1 2 3 4
*/
Menu Driven Code
In the code provided below, I've created a user-friendly, menu-driven program allowing array insertion, deletion, and display within a vector. The program utilizes functions to handle these operations and a menu-driven interface to allow the user to choose the desired action.
Here's an overview of what the code does:
Main Function: It contains the menu where the user selects the operation they want to perform - Insertion (1), Deletion (2), or Displaying (3). The program continues to execute until the user inputs a number outside the range of 1-3.
Insert Function (insert): Prompts the user to input the number of arrays they want to insert and their elements. It pushes these arrays into the vector.
Display Function (Display): Displays all the arrays in the vector if it's not empty.
Delete Function (Delete): Asks the user for the index of the array they want to delete, then deletes that array from the vector.
#include<bits/stdc++.h>
using namespace std;
void insert(vector<array<int, 4>>& vectorofarray);
void Display(vector<array<int, 4>>& vectorofarray);
void Delete(vector<array<int, 4>>& vectorofarray);
int main(){
int n = 1;
vector<array<int, 4>> vectorofarray;
while(n > 0 && n < 4){
cout << "\nEnter your choice:\n1:Insertion\n2:Deletion\n3:Displaying\n";
cout << "To exit, select any option other than 1, 2, or 3.\nYour Choice:";
cin >> n;
switch(n){
case 1:
insert(vectorofarray);
break;
case 2:
Delete(vectorofarray);
break;
case 3:
Display(vectorofarray);
break;
}
}
}
void insert(vector<array<int, 4>>& vectorofarray) {
int no_of_Array;
cout <<"\nEnter no. of array to be inserted in vector: ";
cin >> no_of_Array;
for(int i = 0; i < no_of_Array; i++){
cout <<"Enter " << i+1<<" array element\n";
array <int, 4> arrayy;
for(int j = 0; j < 4; j++){
cin >> arrayy[j];
}
vectorofarray.push_back(arrayy);
}
}
void Display(vector<array<int, 4>>& vectorofarray){
if(vectorofarray.empty()){
cout << "\nVector is empty\n";
}
else{
cout << "\n Displaying\n";
for (const auto& arr : vectorofarray) {
for (const int& element : arr) {
cout << element << " ";
}
cout <<"\n";
}
}
}
void Delete(vector<array<int, 4>>& vectorofarray){
int index;
if(vectorofarray.empty()){
cout << "\nVector is empty\n";
}
else{
cout<<"\nBefore Deletion\n";
for (const auto& arr : vectorofarray) {
for (const int& element : arr) {
cout << element << " ";
}
cout <<"\n";
}
cout <<"\nTo remove a specific array at a particular position, please enter the index of the vector.\n";
cin >> index;
if(index > -1 && index < vectorofarray.size()){
vectorofarray.erase(vectorofarray.begin()+index);
cout <<"\nAfter Deletion\n";
for (const auto& arr : vectorofarray) {
for (const int& element : arr) {
cout << element << " ";
}
cout <<"\n";
}
}
else{
cout <<"\nOut of bound\n";
}
}
}
Complexity Analysis
Let's analyze the time and space complexity of the entire program:
Time Complexity:
In this program, the time complexity for different operations is as follows:
- Insertion (insert function): O(n * m)
- Display (Display function): O(n * m)
- Deletion (Delete function): O(n)
Here, n represents the number of arrays in the vector, and m signifies the fixed size of each array.
Space Complexity:
The primary space complexity in the program arises from the storage of the vector of arrays:
- Vector of Arrays: O(n * m)
This space complexity is directly related to the number of arrays (n) and the size of each array (m). Overall, the space complexity scales linearly with the number of arrays and their sizes.
Conclusion
The exploration of the vector of arrays in C++ illustrates a robust approach to managing grouped data with arrays of uniform sizes within a vector structure. It showcases the efficiency of this approach, enabling sequential access and streamlined organization of arrays. The article dives into various operations, providing code examples for insertion, accessing, and deletion, along with a menu-driven program for practical implementation.
Moreover, a vector of vectors often presents enhanced flexibility and ease of use as compared to a vector of arrays in terms of dynamic size, simplified management, versatility, and streamlined syntax.