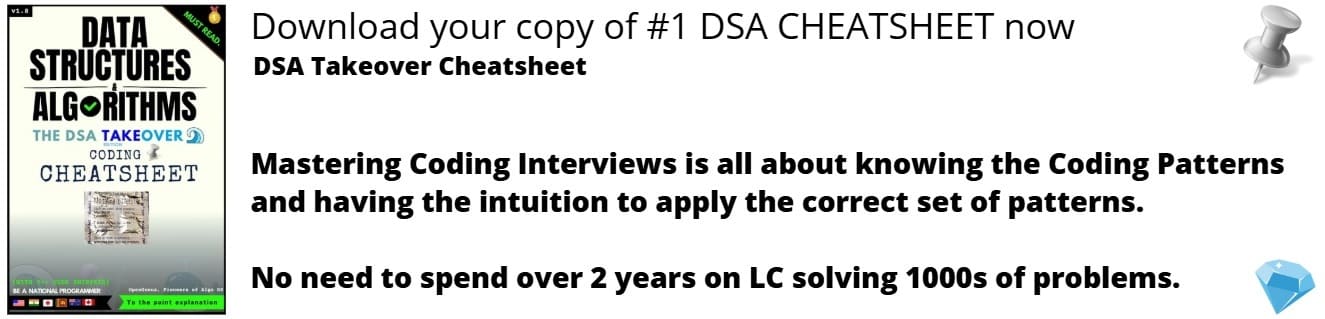
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes
Storage class is used to define the lifetime and visibility of a variable and/or function within a C++ program.These specifiers precede the type that they modify.
Lifetime refers to the period during which the variable remains active and visibility refers to the module of a program in which the variable is accessible.
The following storage classes can be used in a C++ program:
- Automatic
- External
- Static
- Register
- Mutable
Table:
1. Automatic Storage Class:
- Automatic storage class assigns a variable to its default storage type.
- auto keyword is used to declare automatic variables.
- However,if a variable is declared without any keyword inside a function,it is automatic by default.
- This variable is visible only within the function.
- It is declared and its lifetime is same as the lifetime of the function as well.
- Once the execution of function is finished,the variable is destroyed.
Syntax:
datatype var1[= value];
or
auto datatype var1[= value];
Example:
auto int x;
float y =5.67; // both these have the same storage classes
2. External Storage Class:
- External storage class assigns variable a reference to global variable declared outside the given programme.
- extern keyword is used to declare external variables.
- They are visible throughout the programe and its lifetime is same as the lifetime of the programe where it is declared.
- This is visible to all the functions present in the programe.
Syntax:
extern datatype var1;
Example1:C++ program to create and use external storage.
First File : main.cpp
#include <iostream>
int count ;
extern void write_extern();
main() {
count = 5;
write_extern();
}
Second File support.cpp
#include <iostream>
extern int count;
void write_extern(void) {
std::cout << "Count is " << count << std::endl;
}
3. Static Storage Class:
- Static storage class ensures a variable has the visibility mode of a local variable but lifetime of an external variable.
- static keyword is used.
- It can be used only within the function where it is declared but destroys only after the program execution has finished.
- Therefore, making local variables static allows them to maintain their values between function calls.
- When a function is called, the variable defined as static inside the function retains its previous value and operates on it.
Syntax:
static datatype var1[= value];
Example:
static int x = 35;
static float addition;
4. Register Storage Class:
- The register storage class is used to define local variables that should be stored in a register instead of RAM.
- It has its visibility and lifetime same as automatic variable.
- register keyword is used.
- The purpose of creating register varaiable is to increase access speed and make program run faster.
- If there is no space in the register, these variables are stored in main memory and act similar to variables of automatic storage class.
- The register should only be used for variables that require quick access such as counters.
Syntax:
register datatype var1[= value];
Example:
register char z;
register int rollno;
5. Mutable Storage Class:
- The In C++, a class object can be kept constant using keyword const. This doesn't allow the data members of the class object to be modified during program execution.
- mutable specifier applies only to class objects.
- mutable keyword is used.
- It allows a member of an object to override const member function.
- That is, a mutable member can be modified by a const member function.
Syntax:
mutable datatype var1;
Example:
mutable char a;
Question
The storage class is used to specify control of two different properties:
both
scope of variables
storage lifetime of variables
none
The storage class is used to specify control of two different properties: storage lifetime and scope(visibility) of variables.