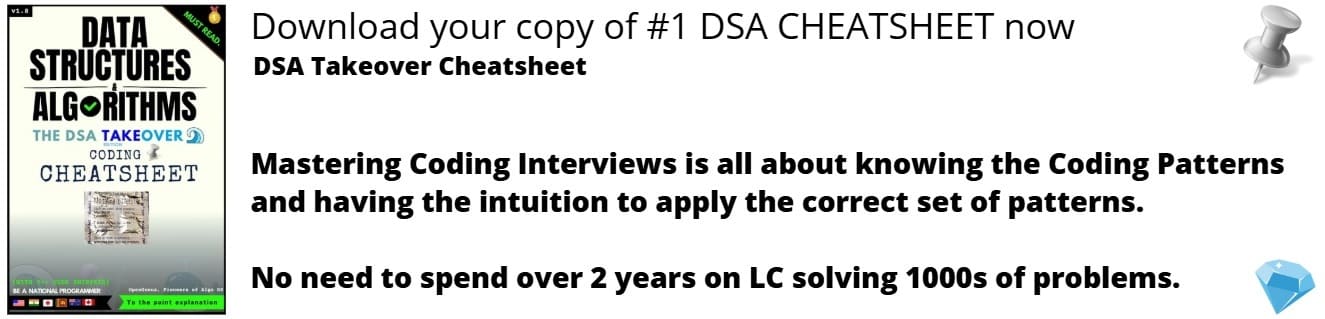
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
"Stream" in C++ refers to the flow of data in memory or between memory and external devices (files, screens, etc.). instream is an input stream, and ostream is an output stream.
You can use the stream classes in the standard library to do input and output on strings. This is a substitute in C++ language for the sprintf and sscanf functions in C language.
There is basic_istringstream<> for reading, basic_ostringstream<> for writing, and basic_stringstream<> for both reading and writing . There are also wide-character versions of each: basic_wistringstream<> , basic_wostringstream<> , basic_wstringstream<> .
The symbol <> means they are all class templates.
In this article we are going to learn about istringstream and explore how to use it for various input cases using C++ code examples.
Table of Content:
- Introduction to stringstream & istringstream
- istringstream - Reading from a string stream
- Streaming integer from a string with std::istringstream
- Streaming mixed data types from a string with std::istringstream
- Uses of istringstream
- Conclusion
Introduction to stringstream & istringstream
What is stringstream?
A string stream is a data stream in which a string object or character array in memory is used as an input and output object , that is, data is output to a string stream object or data is read in from a string stream object, also known as a memory stream.
Stringstream is used to extract numbers from strings, separate strings with blanks, and change formats. To use stringstream, include a library called sstream.
To use a variable of the stringstream class, declare it like this:
#include <sstream>
using namespace std;
std::stringstream ss;
The < sstream > library defines three types:
- istringstream
- ostringstream
- stringstream
which are used to perform stream input, output and input and output operations respectively.
So, istringstream is a variant of stringstream for input operations only.
istringstream - Reading from a string stream
Concept:
To read from the string stream, pass the string to the istringstream object and then use the >> operator to receive the value to the variable.
Normally we use istringstream just like cin and >> and getline() to parse text into pieces(tokens).
The >> (extraction operator) uses spaces to separate strings into tokens.
Implementation:
Here is a C++ code to expand on the meaning and working of above statement.
// Part of iq.opengenus.org
#include <iostream>
#include <sstream>
int main () {
std::string str = "1 2 3 4 5";
std::istringstream iss (str);
for (int n=0; n<5; n++)
{
int mult;
iss >> mult;
std::cout << mult*2 << '\n';
}
return 0;
}
Output:
2
4
6
8
10
Explanation:
We first create a string object here str, for istringstream.
Next we create an object for istringstream here iss, and give str as input in it.
Now using the >> extractor operator we can separate these into pieces or tokens based on the spaces left in between the string given.
Here mult variable receives string each starting after and ending before a space and the following code works successfully.
Streaming integer from a string with std::istringstream
In the below code we are going to see how we can stream an integer and float from a string by using isstringstream.
Implementation:
// Part of iq.opengenus.org
#include <iostream>
#include <sstream>
int main()
{
std::string str("12.24");
std::istringstream iss(str);
int i;
double f;
iss >> i >> f;
std::cout << i << " " << f << std::endl;
}
Output:
12 0.24
Note : If we were to use int f in the above code, it would have given us an error. Reason, being it is necessary to use the right data type to represent the string when streaming.
Streaming mixed data types from a string with std::istringstream
In the below code we are going to see how we can stream different data types from a string by using isstringstream.
Implementation :
Here our goal is to extract information from the given statement using istringstream.
// Part of iq.opengenus.org
#include <iostream>
#include <sstream>
using namespace std;
int main() {
string statement = "Opengenus 14 C++";
string prefix;
string Num;
string language;
istringstream inputStringStream;
inputStringStream.str(statement);
inputStringStream >> prefix;
cout << "Prefix: " << prefix << endl;
inputStringStream >> Num;
cout << "number: " << Num << endl;
inputStringStream >> language;
cout << "Language: " << language << endl;
}
Output:
Prefix: Opengenus
number: 14
Language: C++
Uses of istringstream
- The foremost use is to simply stream a string as input in C++ language.
- It can be used as a boolean to determine if the extraction operation succeeded or failed.
- To extract from string even with multiple data types by creating an object of istringstream.
Conclusion
In this article at OpenGenus, we learnt about the basic of stringstream and how to use istringstream to input a string and also its various uses.