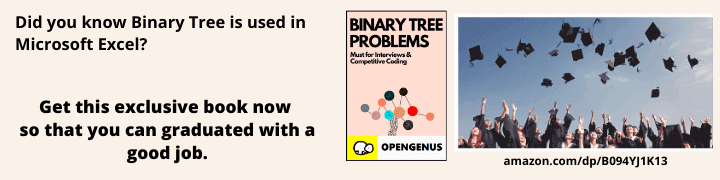
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have presented 50 interview questions based on struct (Structure) in C++ and C Programming Language. Each question is provided with the answer and detailed explanation.
Table of Content
- Multiple Choice Questions
- Descriptive Questions
- Practical Questions
Multiple Choice Questions
1. Which of the following statements is true about C++ structs?
a) Structs can have member functions.
b) Structs can only have member variables.
c) Structs can inherit from other structs.
d) Structs cannot have constructors.
Answer: a) Structs can have member functions.
Explanation:
In C++, structs are very similar to classes, but with a few key differences. One of the main differences used to be that classes could have member functions (also known as methods) while structs could not.
2. Which keyword is used to define a struct in C++?
a) class
b) struct
c) typedef
d) using
Answer: b) struct
Explanation:
In C++, a struct is a user-defined data type that allows you to group together different variables of various data types into a single unit.
3. What is the default access specifier for members of a struct in C++?
a) public
b) private
c) protected
d) friend
Answer: a) public
Explanation:
In C++, when you define a struct, the default access specifier for its members is "public."
4. Which of the following is NOT a valid way to access a member of a struct in C++?
a) Using the dot operator (.)
b) Using the arrow operator (->)
c) Using the double colon operator (::)
d) Using the dot operator with the struct's name followed by the member name
Answer: c) Using the double colon operator (::)
Explanation:
In C++, the double colon operator (::) is known as the scope resolution operator. It is used to access members of a namespace or static members of a class.
5. Which of the following statements is true about the memory allocation for a struct in C++?
a) Memory is automatically allocated on the heap.
b) Memory is automatically allocated on the stack.
c) Memory allocation depends on the struct's size.
d) Memory allocation is managed by the operating system.
Answer: b) Memory is automatically allocated on the stack.
Explanation:
In C++, when you create an instance of a struct, the memory for that struct is automatically allocated on the stack by default.
6. What is the maximum size of a structure in C++?
a) 64 bits
b) 128 bits
c) 256 bits
d) It depends on the compiler and platform.
Answer: d) It depends on the compiler and platform.
Explanation:
In C++, the maximum size of a structure (struct) is not fixed and can vary based on the compiler and the platform on which the code is being compiled and executed.
7. What is the keyword used to define an alias for a structure in C++?
a) typedef
b) struct
c) alias
d) using
Answer: a) typedef
Explanation:
In C++, the "typedef" keyword is used to define an alias for a structure (or any other data type).
8. Which of the following is not a valid structure declaration in C++?
a) struct Point { int x, y; };
b) struct { int x, y; } Point;
c) typedef struct { int x, y; } Point;
d) struct Point { int x, y; } p;
Answer: b) struct { int x, y; } Point;
Explanation:
The reason is that when using the struct keyword to define a structure, you need to provide a name for the structure, so it becomes a user-defined data type that can be used to create instances.
9. Which of the following statements is true about structure initialization in C++?
a) Structures cannot be initialized at the time of declaration.
b) Default values are automatically assigned to structure members.
c) Initial values can be assigned to structure members using braces {}.
d) Structures can only be initialized using constructor functions.
Answer: c) Initial values can be assigned to structure members using braces {}.
Explanation:
In C++, structures can be initialized at the time of declaration, and you can assign initial values to their members using curly braces {}.
10. Which operator is used to allocate memory for a structure dynamically in C++?
a) new
b) malloc
c) allocate
d) create
Answer: a) new
Explanation:
In C++, the "new" operator is used to dynamically allocate memory for a structure (or any other data type) on the heap.
11. Which of the following statements is true about the size of an empty structure in C++?
a) An empty structure does not occupy any memory.
b) The size of an empty structure is 1 byte.
c) The size of an empty structure is 0 bytes.
d) The size of an empty structure is compiler-dependent.
Answer: c) The size of an empty structure is 0 bytes.
Explanation:
In C++, an empty structure, also known as a "null" or "empty" struct, is a structure that does not have any data members defined within it.
12. What is the scope of a structure tag name in C++?
a) Local to the structure definition only.
b) Global to the entire program.
c) Local to the structure members only.
d) Limited to the file in which the structure is defined.
Answer: a) Local to the structure definition only.
Explanation:
In C++, the scope of a structure tag name is limited to the structure definition itself.
13. Which of the following statements is true about nested structures in C++?
a) Structures cannot be nested in C++.
b) Nested structures cannot have member functions.
c) Nested structures can access members of the enclosing structure directly.
d) Nested structures can only be declared inside a function.
Answer: c) Nested structures can access members of the enclosing structure directly
Explanation:
In C++, you can have structures (or classes) nested within other structures. Such nested structures are also known as "nested structs" or "structs within structs."
14. What is the purpose of the sizeof operator in C++?
a) It returns the size of a structure in bytes.
b) It returns the number of elements in an array.
c) It returns the size of a class object.
d) All of the above.
Answer: d) All of the above.
Explanation:
The sizeof operator in C++ is a versatile operator that serves multiple purposes.
15. Which keyword is used to define a constant member in a structure in C++?
a) const
b) constant
c) readonly
d) constexpr
Answer: a) const
Explanation:
In C++, the "const" keyword is used to define a constant member in a structure.
Descriptive Questions
1. What is a struct in C++?
-> A struct in C++ is a user-defined data type that allows you to group together different types of variables under a single name. It is similar to a class, but with default public access for its members. Structs can contain member variables, member functions, and constructors.
2. How is a struct different from a class in C++?
-> In C++, a struct and a class are very similar. The main difference is that the default access specifier for members of a struct is public, while for a class, it is private. Another difference is that structs are typically used for simple data structures, while classes are used for more complex data structures with encapsulation and abstraction.
3. Can a struct in C++ have member functions?
-> Yes, a struct in C++ can have member functions. It can have both member variables and member functions, similar to a class. The member functions can operate on the struct's data members and provide behavior specific to the struct's purpose.
4. How do you define and initialize a struct variable in C++?
-> To define and initialize a struct variable in C++, you can use the following syntax:
struct MyStruct {
int a;
float b;
char c;
};
int main() {
MyStruct myVar = { 10, 3.14, 'X' };
// Alternatively:
// MyStruct myVar;
// myVar.a = 10;
// myVar.b = 3.14;
// myVar.c = 'X';
return 0;
}
We can either initialize the struct variable at the point of declaration or assign values to its member variables separately.
5. What are the members of a struct?
The members of a struct can be variables, functions, or other structs. They are declared inside the curly braces of the struct definition.
6. What is the difference between passing a struct by value and by reference?
When passing a struct by value, a copy of the struct is made, and any modifications made inside the function do not affect the original struct. When passing by reference, the original struct is passed, allowing modifications to affect the original data.
7. Can a struct have access specifiers in C++?
No, access specifiers (like public, private, protected) are not applicable to structs. All members of a struct have the same access level, which is public by default.
8. Can a struct have a member that is a pointer to another struct in C++?
Yes, a struct can have a member that is a pointer to another struct. This allows for creating relationships and accessing related data using the pointer.
9. How do we declare an array of structs in C++?
To declare an array of structs in C++, we use the struct type followed by square brackets and the array name. For example, struct MyStruct arr[5];
declares an array of 5 MyStruct structs.
10. Can a struct have a member function that is overloaded in C++?
Yes, a struct can have member functions that are overloaded. Overloaded member functions have the same name but different parameter lists, allowing for different behaviors based on the arguments passed.
11. What is the difference between declaring a struct and defining a struct in C++?
Declaring a struct involves specifying its name and members, but no memory is allocated. Defining a struct includes both the declaration and memory allocation for struct instances.
12. How do we declare a struct member as mutable in C++?
To declare a struct member as mutable, you use the mutable keyword before the member declaration. This allows the member to be modified even within const member functions.
13. Can a struct have a member function that is a template in C++?
Yes, a struct can have member functions that are templates. This allows for generic programming, where the same functionality can be applied to different types.
14. What is the purpose of using a typedef for a struct in C++?
The purpose of using a typedef for a struct is to create an alias or alternate name for the struct type, making it easier to use and read in code.
15. How do you access a struct member using a reference to a const struct in C++?
To access a struct member using a reference to a const struct, you use the dot operator (.) just like with a regular struct object. The struct being referenced must be const-qualified.
16. How do you declare a struct member as private in C++?
In C++, struct members do not have access specifiers like public or private. By default, all members of a struct have the same access level, which is public.
17. How do you declare a struct member as public in C++?
In C++, struct members do not have access specifiers like public or private. By default, all members of a struct have the same access level, which is public.
18. What is the difference between a struct and an anonymous struct in C++?
A struct has a name and can be declared separately, while an anonymous struct does not have a name and is typically declared inline within another struct or class.
19. How do you access a struct member using a pointer to a struct member in C++?
To access a struct member using a pointer to a struct member, you use the arrow operator (->*)
followed by the member name. For example, if ptr
is a pointer to a struct member x, you can access it as ptr->*x.
20. How do you declare a constant struct member function in C++?
To declare a constant struct member function, you place the const keyword after the function declaration. For example, void functionName() const;
.
21. Can a struct have a member function that is a friend of a namespace in C++?
No, a struct member function cannot be a friend of a namespace. Friend functions are limited to being friends of classes or other functions.
22. Can a struct have a member function that is a friend of an enumeration in C++?
No, a struct member function cannot be a friend of an enumeration. Friend functions are limited to being friends of classes or other functions.
23. How do you define a nested struct within another struct in C++?
To define a nested struct within another struct, you simply declare the nested struct inside the parent struct's definition. For example:
struct ParentStruct {
struct NestedStruct {
// Nested struct members
};
// Parent struct members
};
24. How do you declare a struct member as protected in C++?
In C++, struct members do not have access specifiers like protected
or private
. By default, all members of a struct have the same access level, which is public
.
25. How do we define a struct within a namespace in C++?
To define a struct within a namespace, you declare the struct inside the namespace scope. For example:
namespace MyNamespace {
struct MyStruct {
// Struct members
};
}
Practical Questions -
1. Write a C++ struct named "Person" with member variables for name and age. Include a member function "displayInfo()" that prints the name and age of the person.
struct Person {
std::string name;
int age;
void displayInfo() {
std::cout << "Name: " << name << std::endl;
std::cout << "Age: " << age << std::endl;
}
};
int main() {
Person person1;
person1.name = "John Doe";
person1.age = 30;
person1.displayInfo();
return 0;
}
Output:
Name: John Doe
Age: 30
2. Create a struct named "Rectangle" with member variables for width and height. Write a function "calculateArea()" outside the struct that takes a Rectangle object as a parameter and returns the area of the rectangle.
struct Rectangle {
double width;
double height;
};
double calculateArea(const Rectangle& rectangle) {
return rectangle.width * rectangle.height;
}
int main() {
Rectangle myRectangle;
myRectangle.width = 5.0;
myRectangle.height = 3.0;
double area = calculateArea(myRectangle);
std::cout << "Area: " << area << std::endl;
return 0;
}
Output:
Area: 15
3. How do you declare a pointer to a struct?
A: To declare a pointer to a struct, you use the asterisk (*) operator:
MyStruct* myPointer;
4. How do you initialize a struct using the constructor syntax?
struct MyStruct {
int memberVariable;
MyStruct(int value) : memberVariable(value) {}
};
5. How do you define a struct with a constructor that has default arguments?
struct MyStruct {
int memberVariable;
MyStruct(int value = 0) : memberVariable(value) {}
};
6. : How do you copy the contents of one struct into another struct?
struct MyStruct {
int memberVariable;
// ...
};
MyStruct struct1;
MyStruct struct2;
struct1 = struct2; // Contents of struct2 are copied into struct1
7. Write a struct declaration for a Point that has two member variables, x and y, both of type int.
struct Point {
int x;
int y;
};
8. Write a function called calculateArea that takes a Rectangle struct as an argument and returns its area (width * height).
double calculateArea(Rectangle r) {
return r.width * r.height;
}
With this article at OpenGenus, you must have a good practice of Struct in C++ / C Programming Language.