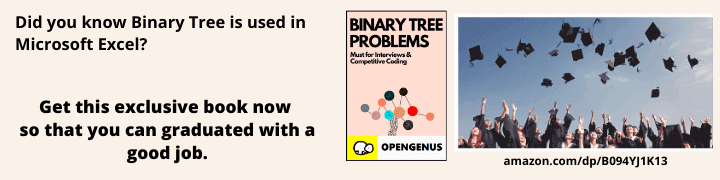
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have demonstrated multiple approaches to swap two numbers without using a third temporary variable.
Table of contents:
- Problem statement: Swap two numbers
- Approach 1: Using + and -
- Approach 2: Using * and /
- Approach 3: Using XOR
Problem statement: Swap two numbers
The problem is to swap the numerical values in two variables without a third variable. The most common approach is as follows:
- Get 2 variables to be swapped: var1 and var2
- Create a new temporary variable var3
- Store value of var2 in var3
- Assign value of var1 to var2. The original value of var2 is lost but is stored in var3.
- Assign value of var3 to var1.
- Both var1 and var2 have been swapped.
In this problem, third variable var3 should not be used. This is the challenge and is one of the most common interview question.
Approach 1: Using + and -
In this approach, the key idea is to use addition and subtraction to keep the value of two variables from getting lost.
The steps of this approach is as follows:
- Get 2 variables to be swapped: var1 and var2
- Store the sum of the two variables in var1 (var1 + var2).
- Assign the value of subtracting var2 from var1 to var2. This is the value of var1. Note the value of var2 is not lost as it is stored in var1 as a sum.
- Assign the value of subtracting var2 from var1 to var1. This is the original value of var2.
a=a+b;
b=a-b;
a=a-b;
The step by step approach is as follows:
a = 5, b = 10
On doing a=a+b: a = 15, b = 10
On doing b=a-b: a = 15, b = 5
On doing a=a-b: a = 10, b = 5
Following is the complete C implementation to swap two numbers without using a third temporary variable by using + and - operators:
// Part of iq.opengenus.org
#include<stdio.h>
int main()
{
int a=5, b=10;
printf("Before swap: a=%d ; b=%d",a,b);
a=a+b;
b=a-b;
a=a-b;
printf("\nAfter swap: a=%d ; b=%d",a,b);
return 0;
}
Approach 2: Using * and /
In this approach, the idea is similar to our previous approach. The key idea is to use multiplication and division to keep the value of two variables from getting lost.
The steps of this approach is as follows:
- Get 2 variables to be swapped: var1 and var2
- Assign the multiplied value var1 * var2 to var1.
- Assign the value of var1 divided by var2 to var2.
- Assign the value of var1 divided by var2 to var1.
a=a*b;
b=a/b;
a=a/b;
The step by step approach is as follows:
a = 5, b = 10
On doing a=a*b: a = 50, b = 10
On doing b=a/b: a = 50, b = 5
On doing a=a/b: a = 10, b = 5
Following is the complete C implementation to swap two numbers without using a third temporary variable by using * and / operators:
// Part of iq.opengenus.org
#include<stdio.h>
int main()
{
int a=5, b=10;
printf("Before swap: a=%d ; b=%d",a,b);
a=a*b;
b=a/b;
a=a/b;
printf("\nAfter swap: a=%d ; b=%d",a,b);
return 0;
}
Approach 3: Using XOR
The key idea of this approach is to use XOR operation. The property of XOR is if a number or data is XORed twice in a given expression, we get back the original expression.
- 2 numbers: a, b
- a XOR b = new number
- a XOR b XOR b = a
- b XOR a XOR b = a
Learn:
This is considered to be the most efficient approach as XOR is a bitwise operation and computers are optimized for bitwise operations compared to other operations like addition.
Following is the complete C implementation to swap two numbers without using a third temporary variable by using the concept of XOR:
// Part of iq.opengenus.org
#include<stdio.h>
int main()
{
int a=5, b=10;
printf("Before swap: a=%d ; b=%d",a,b);
a=a^b;
b=a^b;
a=a^b;
printf("\nAfter swap: a=%d ; b=%d",a,b);
return 0;
}
With this article at OpenGenus, you must have the complete idea of how to swap two numbers efficiently without using a third temporary variable.