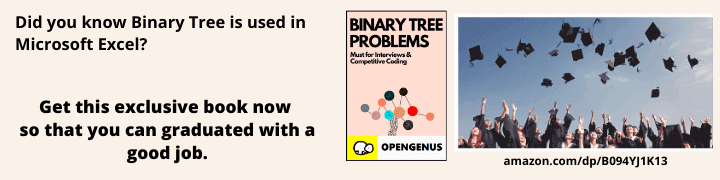
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
If you are a developer interested in making a Crud or you have already done it before, you may think to yourself what is the best ORM, why some ORM's are so complicated to use and connect to the API? And if you are already comfortable with an ORM why would you change it to prisma?
Imagine you are going to create a simple single page application with a minimal server, with some request operations and need to model a database to connect with your API. As it is a side project you don’t want the complexity of sequlize, you don’t need to create all of these files just to have a relationship between tables. And you won’t want to use typeorm because of it’s not so easy to understand documentation and lots of bugs on an ORM that doesn’t have a staff of full time developers to fix these bugs.
So the answer for this simple full-stack project is using the prisma ORM!
Difference between ORMs
You might be asking, what is the difference between all these ORM’s? Aren’t they supposed to model a database with some migrations and connect with API? Well, that is true, but most of them are more complex than they should be.
Let’s take sequelize as an example, to config the ORM using the MVC pattern you should have a config file, models files for each table, a database file for connecting the API, a configuration file for configuration the path of the migrations and possible seeds. The syntax of each model, migration and configuration files are not easy to understand at first. Migrations will generate a file, you will write what you want the table to have and what will the ORM do if something goes wrong, after that you will migrate this migration file.
With prisma you just have to use prisma init for it to add the folders needed on your workspace, create the table with a very simple syntax and then use prisma migrate dev to create the tables you need. Check the simplicity of the syntax, pay attention on the relationships that are being created with just 1 line:
generator client {
provider = "prisma-client-js"
}
datasource db {
provider = "postgresql"
url = env("DATABASE_URL")
}
model User {
id Int @id @default(autoincrement())
name String @unique
email String @unique
password String
post Post[]
created_at DateTime @default(now())
}
model Post {
id Int @id @default(autoincrement())
content String
UserId Int
created_at DateTime @default(now())
postrelation User @relation(fields: [UserId], references: [id])
}
Now let’s see an example of sequelize:
I won’t create the relationship between users and post because a script in another file will be needed, this is just a syntax example
const User = sequelize.define("User", {
id: {
type: DataTypes.INTEGER,
primaryKey: true,
autoIncrement: true
},
name: {
type: Sequelize.STRING,
allowNull: false,
unique: true
},
email: {
type : DataTypes.STRING,
isUnique :true,
allowNull:false,
validate:{
isEmail : true
}
},
password: {
type : DataTypes.STRING,
allowNull:false,
},
createdAt: {
type: Sequelize.DATE,
},
{
timestamps: false,
});
Let’s see a typeorm example of the same table:
It’s recommended to create relationships between tables on different files, for simplicity’s sake I won’t start any relationships in this example.
import { CreateDateColumn } from "typeorm";
@Entity()
export class User extends BaseEntity {
@PrimaryGeneratedColumn()
id: number
@Column({ unique: true })
name: string
@Column({ unique: true })
email: string
@Column()
password: string
@CreateDateColumn({ type: "timestamp", default: () => "CURRENT_TIMESTAMP(6)" })
public created_at: Date;
}
It is pretty obvious that prisma has a very simple syntax with most of the tools that other ORM’s have, the learning curve is faster to reach full proficiency, and if the prisma ORM doesn’t have the validatation you need, you can use zod for validating data directly on the API, that way the database will be reached only with validated data, making every request more optimal.