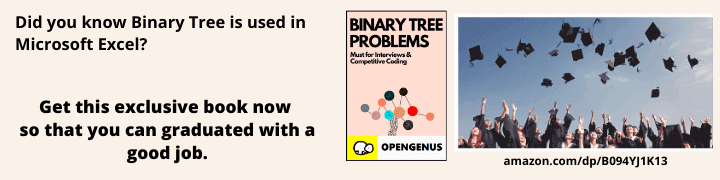
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
-
Introduction
- Brief overview of the project
- Explanation of the technologies used (HTML, CSS, JavaScript)
-
HTML Structure
- Setting up the basic HTML structure
- Creating a container for the piano keys
-
CSS Styling
- Styling the piano keys using CSS
- Differentiating between white and black keys
- Implementing visual styles for keys
-
JavaScript Functionality
- Defining an array of notes
- Creating functions to generate and play keys
- Adding event listeners for key presses
- Incorporating audio playback for each note
-
Testing
- Instructions for testing the project
- Expected behavior when interacting with the virtual piano
-
Conclusion
- Recap of the project's achievements
- Encouragement to explore further and customize the project
Introduction
In this hands-on tutorial at OpenGenus, we will embark on a musical journey by building a fully playable piano using the dynamic trio of web development: HTML, CSS, and JavaScript. You don't need a grand piano or extensive musical knowledge – all you need is your web browser and a creative spirit.
Technologies Used
Before diving into the details, let's briefly discuss the technologies that power this project:
- HTML (Hypertext Markup Language): We'll use HTML to structure our web page and create the foundation for our virtual piano.
- CSS (Cascading Style Sheets): CSS will be our artistic brush, enabling us to style and visually differentiate the piano keys.
- JavaScript: JavaScript brings interactivity to our project. It'll help us generate keys, produce musical notes, and create a responsive user experience.
Now, let's get started by setting up our HTML structure.
HTML Structure
Setting Up the Basic HTML Structure
Our journey begins with the canvas – the HTML structure. We'll create a simple container for our piano keys.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Playable Piano JavaScript | CodingNepal</title>
<link rel="stylesheet" href="styles.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div class="container">
<header>
<h2> PIANO</h2>
</header>
<ul class="piano-keys">
<li class="key white" data-key="a"><span>a</span></li>
<li class="key black" data-key="b"><span>b</span></li>
<li class="key white" data-key="c"><span>c</span></li>
<li class="key black" data-key="d"><span>d</span></li>
<li class="key white" data-key="e"><span>e</span></li>
<li class="key white" data-key="f"><span>f</span></li>
<li class="key black" data-key="g"><span>g</span></li>
<li class="key white" data-key="h"><span>h</span></li>
<li class="key black" data-key="i"><span>i</span></li>
<li class="key white" data-key="j"><span>j</span></li>
<li class="key black" data-key="k"><span>k</span></li>
<li class="key white" data-key="l"><span>l</span></li>
<li class="key white" data-key="m"><span>m</span></li>
<li class="key black" data-key="n"><span>n</span></li>
<li class="key white" data-key="o"><span>o</span></li>
<li class="key black" data-key="p"><span>p</span></li>
<li class="key white" data-key="q"><span>q</span></li>
</ul>
</div>
<script src="script.js"></script>
</body>
</html>
With our HTML framework ready, let's add some style to our piano keys.
CSS Styling
Styling the Piano Keys Using CSS
Our piano needs to look the part. CSS will help us define the appearance of our keys and make them visually appealing.
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
display: flex;
align-items: center;
justify-content: center;
background: #1F2428;
min-height: 100vh;
}
.container {
padding: 35px 40px;
border-radius: 20px;
background: #141414;
}
.container header {
color: #B2B2B2;
align-items: center;
justify-content: space-between;
}
header h2 {
font-size: 1.6rem;
text-align: center;
}
.piano-keys {
display: flex;
list-style: none;
margin-top: 40px;
}
.piano-keys .key {
cursor: pointer;
user-select: none;
position: relative;
text-transform: uppercase;
}
.piano-keys .black {
z-index: 2;
width: 40px;
height: 140px;
margin: 0 -22px 0 -22px;
border-radius: 0 0 5px 5px;
background: linear-gradient(#333, #000);
}
.piano-keys .black.active {
box-shadow: inset -5px -10px 10px rgba(255,255,255,0.1);
background:linear-gradient(to bottom, #000, #434343);
}
.piano-keys .white {
height: 230px;
width: 70px;
border-radius: 8px;
border: 1px solid #000;
background: linear-gradient(#fff 96%, #eee 4%);
}
.piano-keys .white.active {
box-shadow: inset -5px 5px 20px rgba(0,0,0,0.2);
background:linear-gradient(to bottom, #fff 0%, #eee 100%);
}
.piano-keys .key span {
position: absolute;
bottom: 20px;
width: 100%;
color: #A2A2A2;
font-size: 1.13rem;
text-align: center;
}
.piano-keys .black span {
bottom: 13px;
color: #888888;
}
Now, our piano keys have been given life through CSS. They are visually distinguishable as black and white keys, just like a real piano.
Let's move on to the most exciting part – making our piano playable.
JavaScript Functionality
Defining an Array of Piano keys
Every piano needs keys to play. We'll define an array of musical keys that our piano will produce.
This is our javascript file that makes our piano dynamic and interactive
// Define an array of piano keys
const pianoKeys = ["a", "b", "c", "d", "e", "f", "g", "h", "i", "j", "k", "l", "m", "n", "o", "p", "q"];
With our notes ready, we can create functions to generate keys and play sounds.
Creating Functions to Generate and Play Keys
We crafted a function to dynamically create piano keys based on our piano keys and their type (white or black).
function generatePianoKeys() {
const pianoKeysContainer = document.querySelector(".piano-keys");
// Loop through the array of keys and generate HTML elements for each key
pianoKeys.forEach((key) => {
const keyElement = document.createElement("li");
keyElement.className = "key";
keyElement.dataset.key = key;
keyElement.innerHTML = `<span>${key}</span>`;
// Add the key element to the piano keys container
pianoKeysContainer.appendChild(keyElement);
});
}
Adding Event Listeners for Key Presses
To make our piano truly interactive, we added an event listeners to each key. When a key is clicked, it will play the corresponding musical note.
Our piano keys are now ready to sing, but they need a voice. We incorporated audio playback.
function playNote(key) {
// Play the corresponding audio for the key (e.g., "tones/a.mp3")
const audio = new Audio(`tones/${key}.mp3`);
audio.play();
// Add a visual effect to the clicked key (if needed)
const clickedKey = document.querySelector(`[data-key="${key}"]`);
clickedKey.classList.add("active");
// Remove the visual effect after a short delay
setTimeout(() => {
clickedKey.classList.remove("active");
}, 150);
}
// Add click event listeners to each key
function addClickListeners() {
const pianoKeys = document.querySelectorAll(".key");
pianoKeys.forEach((keyElement) => {
keyElement.addEventListener("click", () => {
const key = keyElement.dataset.key;
playNote(key);
});
});
}
Incorporating Audio Playback for Each Note
We used HTML5 audio elements to produce the sweet melodies of our piano.Inside the "tones" folder,We placed an audio files named a.mp3
, b.mp3
, c.mp3
, and so on, matching the musical notes.
Your project directory structure should resemble this:
|-- index.html
|-- styles.css
|-- script.js
|-- tones/
| |-- a.mp3
| |-- b.mp3
| |-- c.mp3
| |-- ...
Our piano is almost ready. All that's left is to test it.
Testing
Instructions for Testing the Project
Open the index.html
file in your web browser,this is thenimage of the virtual keyboard we created below. You'll see a virtual piano waiting for your touch.
Expected Behavior When Interacting with the Virtual Piano
- Clicking on a white key will produce its corresponding musical note.
- Clicking on a black key will also produce its respective note.
- Experiment with different combinations to create your melodies.
Conclusion
This project at OpenGenus.org serves as an excellent starting point for further exploration and customization. You can add more features, improve the design, or even expand it into a full-fledged music application. Let your creativity flow and continue to explore the limitless possibilities of web development. Happy playing!