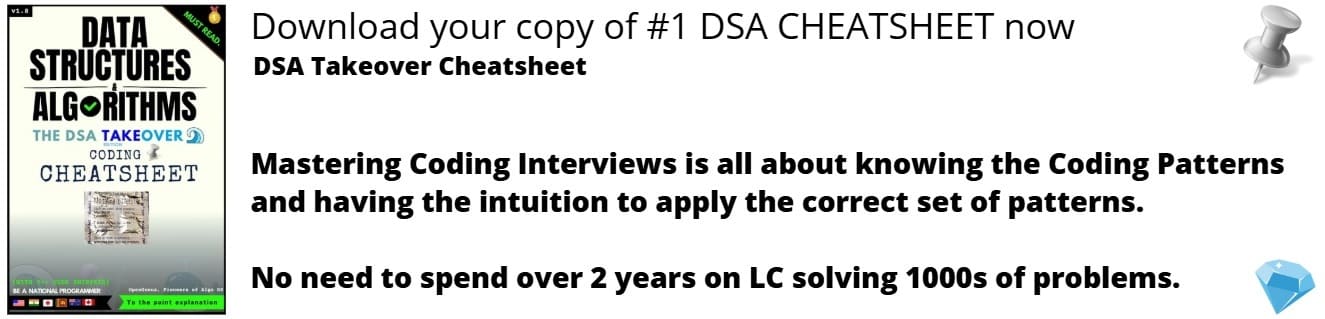
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In modern web development, making HTTP requests is a fundamental aspect of interacting with APIs and fetching data from servers. There are various libraries and tools available for this purpose, and one of the most popular and widely used is Axios. Axios is a promise-based JavaScript library that simplifies the process of making HTTP requests from both the browser and Node.js environments. In this tutorial at OpenGenus, we will dive into the world of Axios and learn how to use it effectively to handle HTTP requests in your web applications.
Table of Contents
- Prerequisites
- Getting Started with Axios
- Making GET Requests with Axios
- Making POST Requests with Axios
- Making DELETE Requests with Axios
- Making PUT Requests with Axios
- Adding Headers and Query Parameters
- Handling Errors
- Conclusion
-
Prerequisites
Before we begin, make sure you have a basic understanding of JavaScript and how to set up a web project with HTML, CSS, and JavaScript. Additionally, you should have a development environment set up on your computer.
-
Getting Started with Axios
Installation
To get started, you need to include Axios in your project. You can do this by either downloading the Axios library from a CDN or using npm (Node Package Manager) if you're working with Node.js.
CDN Method (for Browser-based projects)
Add the following script tag in your HTML file's <head>
or before your JavaScript code:
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
npm Method (for Node.js projects or projects using a bundler like Webpack)
In your project's root directory, open the terminal and run the following command:
npm install axios
Once installed, you can import Axios into your JavaScript code as follows:
const axios = require('axios');
-
Making GET Requests with Axios
Let's start by making a simple GET request using Axios. We will fetch data from a sample API and log the response to the console.
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this code snippet, we use the axios.get()
method to perform a GET request to the provided URL. The then()
method is used to handle the successful response, and the catch()
method is used to catch any errors that might occur during the request.
-
Making POST Requests with Axios
Axios also allows us to make POST requests to send data to the server. Let's create a new post on the same sample API using a POST request.
const newPost = {
title: 'New Post Title',
body: 'This is the body of the new post.',
userId: 1
};
axios.post('https://jsonplaceholder.typicode.com/posts', newPost)
.then(response => {
console.log('New post created:', response.data);
})
.catch(error => {
console.error('Error creating post:', error);
});
In this example, we use the axios.post()
method to send the newPost
object to the specified URL. The server should respond with the newly created post, which we then log to the console.
-
Making DELETE Requests with Axios
In addition to sending GET and POST requests, Axios also supports making DELETE requests to remove resources from the server. Deleting resources is a crucial aspect of interacting with APIs, especially when you want to remove data or records from the backend. Let's see how to perform a DELETE request using Axios:
const postIdToDelete = 1;
axios.delete(`https://jsonplaceholder.typicode.com/posts/${postIdToDelete}`)
.then(response => {
console.log('Post deleted successfully:', response.data);
})
.catch(error => {
console.error('Error deleting post:', error);
});
In the above example, we use the axios.delete()
method and provide the URL of the specific resource we want to delete. In this case, we're deleting a post with the ID postIdToDelete
. The .then()
method is used to handle the successful response after the resource is deleted, and the .catch()
method is used to catch and handle any errors that may occur during the deletion process.
-
Making PUT Requests with Axios
Besides creating and deleting resources, web applications often require updating existing data on the server. This can be achieved using either a PUT or PATCH request. A PUT request replaces the entire resource, while a PATCH request updates only the specified fields. Let's see how to perform an update request using Axios:
const postToUpdate = {
title: 'Updated Title',
body: 'This is the updated body of the post.'
};
const postIdToUpdate = 1;
axios.put(`https://jsonplaceholder.typicode.com/posts/${postIdToUpdate}`, postToUpdate)
.then(response => {
console.log('Post updated successfully:', response.data);
})
.catch(error => {
console.error('Error updating post:', error);
});
we send the updated data in the postToUpdate
object to the specified URL using axios.put()
. The server will process the request and return the updated resource in the response.
-
Adding Headers and Query Parameters
Often, when making requests, you may need to include headers or query parameters. Axios allows you to do this easily by passing an options object as the second argument.
For example:
const headers = {
Authorization: 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
const queryParams = {
param1: 'value1',
param2: 'value2'
};
axios.get('https://api.example.com/data', {
headers: headers,
params: queryParams
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.error('Error fetching data:', error);
});
In this example, we pass the headers
and params
options as part of the options object. This allows us to include custom headers, such as an authentication token, and specify query parameters for GET requests.
-
Handling Errors
Axios provides an easy way to handle errors in your requests. As you've seen in previous examples, you can use the .catch()
method to catch and handle any errors that occur during the request.
Additionally, Axios also considers HTTP status codes in the range of 200 to 299 as successful responses. Any status outside this range will be treated as an error. For example, a 404 Not Found or 500 Internal Server Error will trigger the .catch()
block.
Conclusion
In this tutorial at OpenGenus, we explored the basics of using Axios to make HTTP requests in web applications. We learned how to perform GET and POST requests, set headers and query parameters, handle errors, and make concurrent requests. Axios simplifies the process of working with APIs and provides a convenient way to interact with servers both in the browser and Node.js environments. It is a powerful tool that every modern web developer should have in their toolkit.