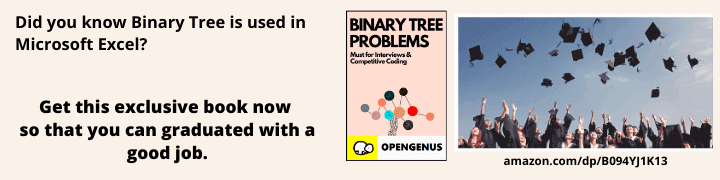
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Promises, a built-in feature of JavaScript, let you handle asynchronous actions in a way that is more readable and organized. In this article at OpenGenus, we'll demonstrate a few interview questions that pertain to JavaScript promises.
Section A
Multiple-choice question
Q1. What is a Promise in JavaScript?
a) A JavaScript object representing the eventual completion or failure of an asynchronous operation.
b) A built-in JavaScript function for handling errors.
c) A data type used for storing multiple values.
d) A method used for synchronizing multiple asynchronous operations.
Answer: a) A JavaScript object representing the eventual completion or failure of an asynchronous operation.
Explanation: A Promise in JavaScript represents the eventual outcome (either successful completion or failure) of an asynchronous operation, providing a cleaner way to handle asynchronous code.
Q2. How do you create a new Promise in JavaScript?
a) Using the new Promise()
constructor.
b) Using the Promise.resolve()
method.
c) Using the Promise.reject()
method.
d) All of the above.
Answer: d) All of the above.
Explanation: You can create a new Promise using the new Promise() constructor or using the static methods Promise.resolve() and Promise.reject().
Q3. Which of the following methods is used to handle a successful Promise?
a) .then()
b) .catch()
c) .finally()
d) All of the above.
Answer: d) All of the above.
Explanation: You can use the .then(), .catch(), and .finally() methods to handle a successful Promise, error handling, and cleanup operations, respectively.
Q4. What does the .then()
method do?
a) It is used to handle errors that occur during a Promise.
b) It is used to handle the fulfillment of a Promise.
c) It is used to handle the rejection of a Promise.
d) It is used to indicate that a Promise has completed.
Answer: b) It is used to handle the fulfillment of a Promise.
Explanation: The .then() method is used to handle the fulfillment of a Promise, meaning it executes the callback function when the Promise is resolved successfully.
-
Which of the following is true about Promise chaining?
a) Promises cannot be chained together.
b) Chaining promises allows sequential execution of asynchronous operations.
c) Chaining promises can only be done using the.then()
method.
d) Promise chaining is used only for error handling.Answer: b) Chaining promises allows sequential execution of asynchronous operations.
Explanation: Promise chaining allows you to chain multiple asynchronous operations, ensuring they execute in a specific order, one after the other. -
What does the
.catch()
method do?
a) It is used to handle successful resolutions of a Promise.
b) It is used to handle errors that occur during a Promise.
c) It is used to indicate that a Promise has completed.
d) It is used to handle rejections of a Promise.Answer: d) It is used to handle rejections of a Promise.
Explanation: The .catch() method is used to handle the rejection of a Promise, meaning it executes the callback function when the Promise is rejected with an error. -
Which method is used to handle clean-up operations that should be executed regardless of the Promise's outcome?
a).then()
b).catch()
c).finally()
d) None of the above.Answer: c) .finally()
Explanation: The .finally() method is used to specify a callback function that should be executed, regardless of whether the Promise is fulfilled or rejected, to perform cleanup operations. -
Which method is used to convert a callback-based asynchronous operation into a Promise-based operation?
a)setTimeout()
b)Promise.race()
c)Promise.all()
d)new Promise()
Answer: d) new Promise()
Explanation: To convert a callback-based asynchronous operation into a Promise-based operation, you create a new Promise using the new Promise() constructor. -
What does the
Promise.all()
method do?
a) It returns a new Promise that is rejected if any of the input Promises are rejected.
b) It returns a new Promise that is resolved with an array of values when all the input Promises are resolved.
c) It returns a new Promise that is resolved if any of the input Promises are resolved.
d) It returns a new Promise that is resolved after a specific timeout.Answer: b) It returns a new Promise that is resolved with an array of values when all the input Promises are resolved.
Explanation: Promise.all() takes an array of Promises and returns a new Promise that is resolved with an array containing the resolved values from all the input Promises. -
Which method is used to create a Promise that resolves or rejects after a specified delay?
a)Promise.race()
b)Promise.all()
c)Promise.reject()
d)setTimeout()
Answer: d) setTimeout()
Explanation: setTimeout() is used to create a delayed operation and is not directly related to creating Promises. However, Promises can be combined with setTimeout() to create a Promise that resolves or rejects after a specified delay.
Section B
Descriptive Questions
Q1. What is the purpose of Promises in JavaScript, and why are they useful?
In JavaScript, promises are used to manage asynchronous actions and offer a more structured and legible approach to construct asynchronous programming. They facilitate better error handling and the sequential execution of asynchronous operations in addition to preventing callback hell.
Q3. Explain the three possible states of a Promise.
- Pending: The initial state when the Promise is created and not yet fulfilled or rejected.
- Fulfilled: The state when the Promise is successfully resolved with a value.
- Rejected: The state when an error occurs, and the Promise is rejected with a reason or an error object.
Q4. How do you handle errors in Promise chains?
Errors in Promise chains can be handled using the .catch()
method at the end of the chain. It will catch any errors that occurred in any of the previous Promise rejections.
Q5. What is Promise chaining, and why is it beneficial?
Promise chaining is a technique in JavaScript for managing asynchronous operations in a sequential and readable way. It involves linking multiple promises together by attaching callbacks, creating a chain of operations. Each operation returns a new promise, allowing for the chaining of additional asynchronous tasks. Promise chaining improves code readability, centralizes error handling, ensures synchronization of tasks, and facilitates passing results between promises. While async/await is becoming more popular, understanding promise chaining remains valuable for working with promises directly or older codebases.
Q6. Explain the difference between using .then().catch()
and .then().finally()
for error handling in Promises.
.then().catch()
is used for error handling when the Promise is rejected, whereas .then().finally()
is used for executing code regardless of whether the Promise is fulfilled or rejected. The .finally()
block will be executed after the Promise is settled.
Q7. What is the purpose of the Promise.all()
method? Provide an example of its usage.
The Promise.all()
method is used when you have multiple Promises and need to wait for all of them to be fulfilled or rejected. It returns a new Promise that is resolved with an array of values when all the input Promises are resolved, or rejected if any of the Promises are rejected. Example usage:
const promise1 = new Promise((resolve) => setTimeout(resolve, 1000, 'Hello'));
const promise2 = new Promise((resolve) => setTimeout(resolve, 2000, 'World'));
const promise3 = new Promise((resolve) => setTimeout(resolve, 1500, '!'));
Promise.all([promise1, promise2, promise3])
.then((values) => console.log(values.join(' '))); // Output: Hello World !
Q8. What is the purpose of the Promise.race()
method? Provide an example of its usage.
The Promise.race()
method is used when you have multiple Promises and want to handle the result (fulfilled or rejected) of the first Promise that resolves or rejects. Example usage:
const promise1 = new Promise((resolve) => setTimeout(resolve, 1000, 'Hello'));
const promise2 = new Promise((resolve, reject) => setTimeout(reject, 500, 'Error'));
Promise.race([promise1, promise2])
.then((value) => console.log(value)) // Output: Error
.catch((error) => console.log(error)); // Output: Error
Q9. How do you handle multiple Promises simultaneously using Promise.all()
and Promise.race()
?
Promise.all()
is used to handle multiple Promises when you want to wait for all of them to be fulfilled or rejected. Promise.race()
is used when you want to handle the result of the first Promise that resolves or rejects.
Q10. Explain the concept of async/await and how it relates to Promises.
Async/await is a syntax introduced in ES2017 that simplifies asynchronous code by allowing you to write it in a more synchronous style. The async
keyword is used to declare an asynchronous function, and the await
keyword is used to pause the execution of the function until a Promise is fulfilled or rejected. It provides a more readable and intuitive way to work with Promises.
Q11. Can you provide an example of converting a callback-based function into a Promise-based function?
Sure! Here's an example of converting a callback-based setTimeout
function into a Promise-based function:
function delay(ms) {
return new Promise((resolve) => setTimeout(resolve, ms));
}
delay(2000).then(() => console.log('Delayed operation')); // Output after 2 seconds: Delayed operation
Q12. How do you cancel a Promise or clean up resources associated with it?
Promises in JavaScript do not have built-in cancellation. However, you can use other techniques such as using a flag variable or an external library specifically designed for Promise cancellation to handle cancellation and clean up resources associated with it.
Q13. What are some common mistakes or pitfalls to be aware of when working with Promises?
- Forgetting to handle Promise rejections using .catch()
, which can lead to unhandled Promise rejections.
- Mixing Promises and callbacks within the same codebase, which can lead to confusion and complexity.
- Not returning Promises from within .then()
callbacks when chaining Promises, which can break the chain.
- Not properly handling errors within Promise chains, leading to silent failures.
- Failing to understand the difference between Promise.resolve()
and Promise.reject()
, which can affect the behavior of Promises.