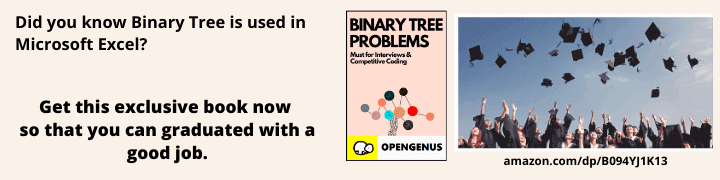
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
INTRODUCTION
SEO, or search engine optimization, is a practice aimed at improving a website's visibility and ranking on search engine results pages. It involves various strategies and techniques that optimize website content, structure, and design to make it more appealing to search engines and users alike. By implementing SEO, website owners strive to increase organic (non-paid) traffic to their site by targeting relevant keywords, improving website speed and performance, creating high-quality and engaging content, obtaining backlinks from reputable sources, and enhancing the overall user experience. Effective SEO practices can lead to higher search engine rankings, greater online visibility, and increased chances of attracting and retaining visitors, ultimately contributing to the success and growth of a website or online business.
When it comes to optimizing websites built with React, SEO plays a crucial role in ensuring that the content is discoverable by search engines. React, being a JavaScript framework, has some unique considerations for SEO. One important aspect is the implementation of server-side rendering (SSR) or prerendering to generate HTML content that search engines can easily crawl and index. This can be achieved using tools like Next.js, Gatsby, or custom SSR setups with Node.js. Additionally, integrating a Node package like Helmet can further enhance SEO capabilities by allowing the management of meta tags, title tags, and other crucial HTML elements. Helmet provides an easy and efficient way to control the SEO-related aspects of a React application. Later in this discussion, we will delve deeper into the implementation of Helmet and explore its benefits in optimizing React websites for search engines.
In order to make a React site visible to search engines, there are several important steps to consider:
- Server-Side Rendering (SSR)
- Proper Routing
- Metadata Optimization
- Generating and Submiting Sitemap
- Structured Data Markup
- Page Speed Optimization
- Mobile Responsiveness
- Backlink Building
These steps provide a foundation for making your React site visible to search engines. In the following discussions, we will explore each of these steps in more detail, discussing best practices and implementation techniques to optimize your React site for search engine visibility.
Server-Side Rendering (SSR)
Implement server-side rendering or prerendering to generate HTML content on the server that can be easily crawled and indexed by search engines. This ensures that search engines can understand and analyze the website's content.
For example:
// pages/index.js
import React from 'react';
import { renderToString } from 'react-dom/server';
const Home = () => {
return (
<div>
<h1>Welcome to My React App!</h1>
<p>This is an example of server-side rendering in React.</p>
</div>
);
};
export async function getServerSideProps() {
const appHTML = renderToString(<Home />);
return {
props: {
appHTML
}
};
}
export default function Index({ appHTML }) {
return (
<html>
<head>
<title>My React App</title>
</head>
<body>
<div id="root" dangerouslySetInnerHTML={{ __html: appHTML }} />
<script src="/path/to/your/bundle.js"></script>
</body>
</html>
);
}
In this example, we have a simple React component Home that renders some content. The getServerSideProps function is a special function provided by Next.js that runs on the server and generates the HTML content for the Home component using renderToString from react-dom/server.
The getServerSideProps function returns an object with the appHTML property containing the rendered React component's HTML.
The Index component is the entry point for the server-rendered page. It receives the appHTML as a prop and sets it as the inner HTML of the <div id="root">
element. This allows the server-rendered content to be displayed when the page loads.
Note that the example assumes you have a bundle.js file that contains the JavaScript code for your React application. Make sure to include the correct path to your bundle file in the <script>
tag.
By implementing server-side rendering in this way, search engines can easily crawl and index the generated HTML, allowing them to understand and analyze the content of your React application for better visibility in search results.
Proper Routing
Use a routing solution, such as React Router, to enable search engine-friendly URLs and navigation within your React application. This helps search engines index and crawl different pages of your site effectively.
For example:
// App.js
import React from 'react';
import { BrowserRouter, Route, Switch } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
import NotFound from './components/NotFound';
const App = () => {
return (
<BrowserRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</BrowserRouter>
);
};
export default App;
In this example, we have an App component that serves as the entry point for our React application. We use the BrowserRouter component from React Router to enable routing functionality.
Inside the Switch component, we define different routes using the Route component. Each route specifies a path and the corresponding component to render when that path is matched.
For example, the / path (root) is associated with the Home component, /about is associated with the About component, and /contact is associated with the Contact component.
The exact keyword is used for the root route to ensure that it only matches the exact path and not partial matches.
Finally, we include a catch-all route with no specified path (Route component={NotFound}) that will render the NotFound component for any unknown routes.
By implementing proper routing with React Router, we enable search engine-friendly URLs for different pages of our React application. This helps search engines effectively index and crawl the various pages of our site, enhancing the visibility and discoverability of our content in search results.
Metadata Optimization
Optimize the metadata of your React site, including meta tags, title tags, and descriptions. These elements provide essential information to search engines about the content and purpose of each page, influencing their ranking and visibility in search results.
For example we can use Helmate node package
In React, we can create dynamic meta tags for each of our pages, such as titles, descriptions, and even OGs (Open Graph). To implement this, we can use an excellent package called React Helmet. React Helmet is a React library that allows developers to manage and control a website's meta tags for improved SEO and user experience.
The steps to use React Helmet are:
- npm i react-helmet-async
- inside index.js import HelmetProvider and wrap the App compoenet with the HelmetProvider
- Add data-rh="true" to index.html
- Configure the Helmet on each page such as title, description, meta, link, etc or you can create a component to send the meta info to the helmet as prop.
To check if the above is working, you can install the Meta SEO Chrome Extension and view the meta and link info it will also provide you with tips.
//App.js
root.render(
<HelmetProvider>
<Provider store={store}>
<App/>
<Provider>
</HelmetProvider>
)
//index.html
<Helmet>
<title> OpenGenus IQ </title>
<meta property="og:image"
content="./opengenus.png"
title="opengenus"/>
<meta property="og:title" content="Opengenus"/>
<meta property="og:description" content="opengenus"/>
</Helmet>
Sitemap
A website sitemap, in the context of React or any web development, is a hierarchical representation of the pages or routes within a website. It serves as a blueprint or guide that outlines the structure and organization of the website's content.
In React, a website sitemap can be implemented by leveraging the routing system, such as React Router, which helps define the different routes and components associated with each page or section of the website.
Another way to improve your SEO is to let Google crawl your sitemap file. You can create a "sitemap.txt" file inside your "./public" folder and write your sitemap pages. Then, go to Google Search Console and add a meta tag. After verifying with Google that the tag is added, add a sitemap to Google with "/sitemap.txt" following your URL. There are many packages to generate this site map automatically. You can also upload your sitemap to Microsoft Bing's Webmaster Tool by verifying it with Google Console if you have already used it. This process might take some time for Google or other search engines to index your site.
Structured Data Markup
Implement structured data markup using formats like JSON-LD or Microdata. Structured data provides additional context to search engines about the content on your site, enabling rich snippets and enhancing the visibility and presentation of your pages in search results.
// components/Product.js
import React from 'react';
const Product = ({ name, price, description }) => {
const productSchema = {
'@context': 'https://schema.org/',
'@type': 'Product',
name: name,
image: 'https://example.com/product-image.jpg',
description: description,
offers: {
'@type': 'Offer',
price: price,
priceCurrency: 'USD',
},
};
return (
<div>
<h2>{name}</h2>
<p>{description}</p>
<p>Price: ${price}</p>
{/* JSON-LD structured data */}
<script type="application/ld+json">
{JSON.stringify(productSchema)}
</script>
</div>
);
};
export default Product;
In this example, we have a Product component that renders information about a product, including its name, price, and description. Inside the component, we define a productSchema object representing the structured data using JSON-LD format.
The productSchema object follows the schema.org vocabulary and defines properties such as @context, @type, name, image, description, and offers. These properties provide additional context to search engines about the product.
To include the JSON-LD structured data in the HTML, we use a <script>
tag with the type attribute set to application/ld+json. We stringify the productSchema object using JSON.stringify and place it within the <script>
tag.
By implementing structured data markup using JSON-LD or other formats, we provide search engines with additional information about the content on our site. This enables search engines to understand the context of our products, services, or other entities, and may enhance the visibility and presentation of our pages in search results, such as generating rich snippets with relevant information like prices, ratings, and availability.
Page Speed Optimization
Ensure your React site is optimized for fast loading speeds. Search engines prioritize websites that offer a smooth and quick user experience. Techniques such as code splitting, lazy loading, and optimizing image sizes can help improve page speed.
// components/Home.js
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const Home = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data asynchronously
const fetchData = async () => {
const response = await axios.get('https://api.example.com/data');
setData(response.data);
};
fetchData();
}, []);
return (
<div>
{data ? (
<div>
<h1>Welcome to My React App!</h1>
<p>{data.text}</p>
<img src={data.image} alt="Example" />
</div>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default Home;
In this example, we have a Home component that fetches data from an API endpoint using the axios library. The component starts by rendering a "Loading..." message until the data is fetched successfully.
To improve page speed, we implement the following techniques:
- Code Splitting: By default, React supports code splitting, which allows us to split our application code into smaller chunks. This enables lazy loading and loading only the necessary code when needed, improving initial page load time. React's built-in dynamic import mechanism, or tools like Webpack's code splitting capabilities, can be used to achieve code splitting.
- Lazy Loading: In this example, the data fetching process is triggered after the component is mounted, using the useEffect hook. This ensures that data is fetched asynchronously after the initial rendering, avoiding blocking the main thread and improving the perceived page load speed.
- Optimizing Image Sizes: To optimize image sizes, consider using tools like imagemin or squoosh to compress and resize images before including them in your React application. This helps reduce image file sizes, resulting in faster image loading times and improved overall page speed.
By implementing these page speed optimization techniques, we prioritize a smooth and quick user experience in our React application. Search engines also value fast-loading websites, so optimizing page speed can positively impact search engine rankings and overall user satisfaction.
Mobile Responsiveness
Make your React site fully responsive and mobile-friendly. With the increasing use of mobile devices for browsing, search engines give preference to websites that offer a seamless experience across different screen sizes.
Backlink Building
Focus on acquiring high-quality backlinks from reputable websites. Backlinks act as endorsements for your site's credibility and authority, positively impacting its visibility and search engine rankings.
Summary
In summary, SEO, or search engine optimization, is a practice aimed at improving a website's visibility and ranking on search engine results pages. It involves various strategies and techniques to optimize website content, structure, and design, ultimately attracting organic traffic and enhancing the user experience. When it comes to optimizing websites built with React, specific considerations must be taken into account. Server-side rendering (SSR) or prerendering is crucial to generate HTML content that search engines can crawl and index effectively. Proper routing, metadata optimization, sitemaps, structured data markup, page speed optimization, mobile responsiveness, and backlink building are additional essential steps to make a React site visible to search engines. By implementing these practices, website owners can improve their search engine rankings, increase online visibility, and ultimately contribute to the success and growth of their online presence.
In addition, implementing SEO for a React website involves specific techniques and tools. Using the React Helmet package allows for effective management and control of meta tags, title tags, and other crucial HTML elements. This enhances the website's metadata optimization and improves its visibility in search results. Moreover, employing proper routing with React Router enables search engine-friendly URLs and navigation within the React application. By optimizing page speed, leveraging code splitting and lazy loading techniques, and optimizing image sizes, website owners can improve the overall performance and loading speed of their React site. Mobile responsiveness is also critical, as search engines prioritize mobile-friendly websites. Lastly, backlink building from reputable sources helps establish the website's credibility and authority, leading to improved visibility and search engine rankings. By considering these factors and implementing effective SEO practices, React website owners can enhance their online presence and attract organic traffic from search engines.