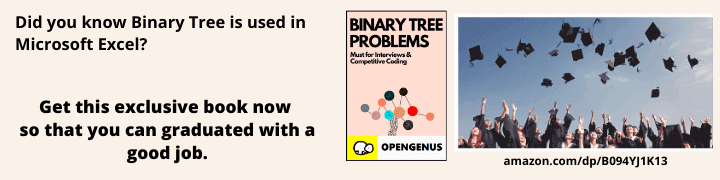
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the realm of computer science, data structures play a vital role in organizing and manipulating data efficiently. One such data structure is the queue. A queue is an abstract data type that follows the First-In-First-Out (FIFO) principle. JavaScript, being a versatile programming language, provides built-in support for implementing queues.
In this article at OpenGenus, we will delve into the queue data structure in JavaScript, understand its underlying principles, and learn how to implement and utilize queues effectively.
Table of contents:
- Understanding the Basics of a Queue
- The Role of the Event Queue
- Implementing a Queue Class
- Advantages of Queues
- Conclusion
Understanding the Basics of a Queue
A queue is a collection of elements that supports two primary operations: enqueue and dequeue. The enqueue operation adds an element to the back of the queue, while the dequeue operation removes the element from the front of the queue. This behavior mirrors a real-life queue, where the first person to enter is the first to be served.
In JavaScript, we can implement a queue using an array or a linked list. Let's start by using an array as the underlying data structure. Consider the following code snippet:
const queue = [];
// Enqueue operation
queue.push(1);
queue.push(2);
queue.push(3);
// Dequeue operation
const frontElement = queue.shift();
console.log(frontElement); // Output: 1
In the above example, we create an empty queue represented by the array queue
. We then perform three enqueue operations, adding elements 1, 2, and 3 to the queue. Finally, we execute a dequeue operation, which removes the frontmost element (1) and assigns it to the frontElement
variable.
The Role of the Event Queue
In addition to its use as a standalone data structure, the queue concept is crucial in event-driven programming. JavaScript utilizes an event queue to manage asynchronous tasks and event handling. When an event occurs or an asynchronous operation is completed, the corresponding callback function or event handler is enqueued into the event queue. The event loop, a fundamental part of JavaScript's event-driven architecture, continuously checks the event queue and processes the events in the order they were enqueued.
Consider the following example:
console.log("Start");
setTimeout(() => {
console.log("Async task 1");
}, 2000);
setTimeout(() => {
console.log("Async task 2");
}, 1000);
console.log("End");
In the above code, we have two asynchronous tasks scheduled using setTimeout()
. These tasks simulate time-consuming operations that will be executed after a certain delay. The execution order is as follows:
- Log "Start"
- Log "End"
- Wait for 1 second
- Log "Async task 2"
- Wait for 1 more second
- Log "Async task 1"
The event queue ensures that the asynchronous tasks are processed in the order they were enqueued, without blocking the main thread's execution.
Implementing a Queue Class
While using an array to implement a queue works well, it can be beneficial to create a custom queue class with dedicated methods for enqueue, dequeue, and other queue operations. This approach provides encapsulation and abstraction, making the code more readable and maintainable.
Let's create a simple Queue class in JavaScript:
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
if (this.isEmpty()) {
return null;
}
return this.items.shift();
}
isEmpty() {
return this.items.length === 0;
}
size() {
return this.items.length;
}
front() {
if (this.isEmpty()) {
return null;
}
return this.items[0];
}
clear() {
this.items = [];
}
}
In the above example, we define a Queue
class with an array items
as the underlying data structure. The class includes methods such as enqueue()
, dequeue()
, isEmpty()
, size()
, front()
, and clear()
, which correspond to the fundamental operations of a queue.
Using the Queue
class, we can rewrite the previous example as follows:
const queue = new Queue();
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
const frontElement = queue.dequeue();
console.log(frontElement); // Output: 1
Advantages of Queues
Queues offer several advantages in various programming scenarios. Here are a few benefits of using queues:
- Task scheduling: Queues are often used to manage task scheduling in concurrent systems. The tasks are enqueued and processed in a sequential order, ensuring fairness and proper resource allocation.
- Breadth-first search: Queues are integral to graph traversal algorithms, such as breadth-first search (BFS). The queue facilitates the exploration of nodes at the same level before moving to deeper levels.
- Buffering and synchronization: Queues can be used as buffers to temporarily hold data in scenarios where data production and consumption rates differ. They ensure that data is processed in a controlled and synchronized manner.
- Message passing: In distributed systems or inter-process communication, queues are used for message passing between different components or nodes. Messages are enqueued and processed in a coordinated fashion.
Conclusion
In conclusion, understanding the queue data structure is essential for any JavaScript developer. Whether it's managing data flow, handling events asynchronously, or implementing algorithms, queues provide a powerful and efficient way to tackle a wide range of programming challenges. By utilizing the built-in array or creating a custom queue class, you can harness the full potential of queues in JavaScript and build robust and elegant solutions.