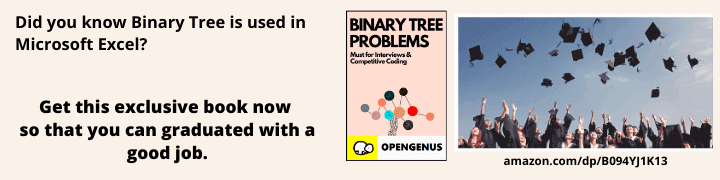
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Why Input Validation and Sanitization?
- Server-Side Validation with Express.js
- Input Sanitization with Validator.js
- Additional Input Validation Libraries
- Conclusion
Why Input Validation and Sanitization?
Input validation is the process of verifying that user input meets specific criteria, such as correct format, length, or type, before processing or storing it. By validating user input, we can ensure that the data is safe and appropriate for its intended use.
Input Validation Examples:
- Username Validation
- Password Validation
- Email Validation
- Numeric Input Validation
Input Sanitization Examples:
- HTML Tag Sanitization
- Escape Special Characters
- Preventing SQL Injection
- File Path Sanitization
Why Input Validation and Sanitization?
Input validation is the process of verifying that user input meets specific criteria, such as correct format, length, or type, before processing or storing it. By validating user input, we can ensure that the data is safe and appropriate for its intended use. Let's explore some common examples of input validation:
-
Username Validation:
- Requirement: Usernames should only contain alphanumeric characters (letters and numbers) and be at least 3 characters long.
- Validation: Use a regular expression to check if the username meets the criteria:
/^[a-zA-Z0-9]{3,}$/
.
-
Password Validation:
- Requirement: Passwords should be at least 8 characters long.
- Validation: Check if the length of the password is at least 8 characters.
-
Email Validation:
- Requirement: Email addresses should be in a valid format (e.g.,
user@example.com
). - Validation: Use a regular expression to verify the email format:
/^[^\s@]+@[^\s@]+\.[^\s@]+$/
.
- Requirement: Email addresses should be in a valid format (e.g.,
-
Numeric Input Validation:
- Requirement: Input should be a positive integer.
- Validation: Check if the input is a number and if it is greater than 0.
Input sanitization, on the other hand, involves cleaning user input to remove or neutralize potentially harmful characters or scripts that could lead to security vulnerabilities. By sanitizing user input, we can prevent attacks such as cross-site scripting (XSS) and protect the application from data corruption. Here are some examples of input sanitization:
-
HTML Tag Sanitization:
- Sanitization: Use a library like Validator.js to remove HTML tags from user input to prevent XSS attacks. Example:
validator.stripTags(input)
.
- Sanitization: Use a library like Validator.js to remove HTML tags from user input to prevent XSS attacks. Example:
-
Escape Special Characters:
- Sanitization: Escape special characters, such as
<
,>
, and&
, to prevent HTML injection attacks. Example:validator.escape(input)
.
- Sanitization: Escape special characters, such as
-
Preventing SQL Injection:
- Sanitization: Use parameterized queries or prepared statements when interacting with databases to prevent SQL injection attacks. This involves using placeholders for user input in SQL queries.
-
File Path Sanitization:
- Sanitization: Ensure that file paths provided by users are valid and do not allow access to unintended directories or files.
Implementing strong input validation and sanitization practices is crucial for several reasons:
- Sanitization: Ensure that file paths provided by users are valid and do not allow access to unintended directories or files.
-
Preventing Security Vulnerabilities: Proper validation and sanitization help protect against common web vulnerabilities, such as cross-site scripting (XSS) and SQL injection, by ensuring that user input cannot be used to execute malicious code or manipulate databases.
-
Ensuring Data Integrity: Validating user input helps maintain data integrity by ensuring that only valid and expected data is processed or stored. It reduces the chances of errors or data corruption caused by invalid input.
-
Enhancing User Experience: By providing immediate feedback on invalid input, you can improve the user experience by guiding users to correct any mistakes or omissions.
Now, let's explore some best practices and examples of input validation and sanitization using JavaScript.
1. Server-Side Validation with Express.js
Server-side validation is a crucial line of defense, as client-side validation can be bypassed. Express.js, a popular Node.js framework, allows us to implement server-side validation easily.
// .eslintrc.js
module.exports = {
env: {
node: true,
es2021: true,
},
extends: 'eslint:recommended',
parserOptions: {
ecmaVersion: 12,
},
rules: {
'no-console': 'off', // Allow console.log statements for demonstration purposes
},
};
// Example: Server-side validation using Express.js
const express = require('express');
const app = express();
// ... Express app configuration
app.post('/register', (req, res) => {
const { username, password, email } = req.body;
// Validate username: alphanumeric with a minimum length of 3 characters
if (!/^[a-zA-Z0-9]{3,}$/.test(username)) {
return res.status(400).json({ error: 'Invalid username' });
}
// Validate password: minimum length of 8 characters
if (password.length < 8) {
return res.status(400).json({ error: 'Invalid password' });
}
// Validate email format using regular expression
if (!/^[^\s@]+@[^\s@]+\.[^\s@]+$/.test(email)) {
return res.status(400).json({ error: 'Invalid email' });
}
// Process the registration logic
// ...
});
// ... Other routes and middleware
app.listen(3000, () => {
console.log('Server started on port 3000');
});
In the above example,we define a route /register that handles user registration. We validate the username, password, and email fields using regular expressions. If any validation check fails, we send a response with an appropriate error message.We have included an .eslintrc.js
file to define ESLint rules for the project. This helps enforce coding standards and catch potential issues. The server-side validation logic remains the same, validating the username, password, and email fields using regular expressions, and returning appropriate error responses if any validation check fails.
2. Input Sanitization with Validator.js
Validator.js is a library that provides various string validation and sanitization functions. We can leverage it to sanitize user input and remove potentially harmful characters.
// Example: Sanitizing user input with Validator.js
const validator = require('validator');
app.post('/comment', (req, res) => {
const { name, comment } = req.body;
// Sanitize the name by removing HTML tags
const sanitizedName = validator.stripTags(name);
// Sanitize the comment by escaping HTML characters
const sanitizedComment = validator.escape(comment);
// Store the sanitized data in the database
// ...
});
In this example,we utilize Validator.js to sanitize user input in a comment form. We use stripTags to remove any HTML tags from the name and escape to escape HTML characters in the comment. This helps prevent potential XSS attacks and ensures the data is stored safely.
we use Validator.js to sanitize user input in a comment form. We demonstrate how to remove HTML tags from the name using stripTags
and escape HTML characters in the comment using escape
. The sanitized data can then be securely stored in the database.
3. Additional Input Validation Libraries
Apart from the native JavaScript and Validator.js examples, there are several popular input validation libraries you can leverage in your projects:
- Joi: Joi is a powerful validation library known for its declarative approach. It allows you to define validation schemas and provides extensive validation capabilities.
- Yup: Yup is a simple and intuitive validation library with a fluent API. It offers easy schema-based validation and supports complex validation scenarios.
- Express Validator: Express Validator is a library specifically designed for input validation and sanitization in Express.js applications. It provides middleware and validator functions to simplify the validation process.
These libraries offer different features, syntaxes, and levels of customization. Choose the one that best fits your
project's requirements and aligns with your development preferences.
Conclusion
Implementing input validation and sanitization is crucial for building secure applications. By validating user input and sanitizing it to remove potential security risks, you can protect against attacks, ensure data integrity, and enhance the user experience.
In this article, we explored best practices for input validation and sanitization using JavaScript. We covered server-side validation with Express.js, input sanitization with Validator.js, and introduced popular input validation libraries like Joi, Yup, and Express Validator.
By applying these best practices and utilizing appropriate libraries, you can build robust and secure applications that protect against common vulnerabilities and maintain data integrity. Remember to consistently validate and sanitize user input throughout your application, paying close attention to potential security risks.