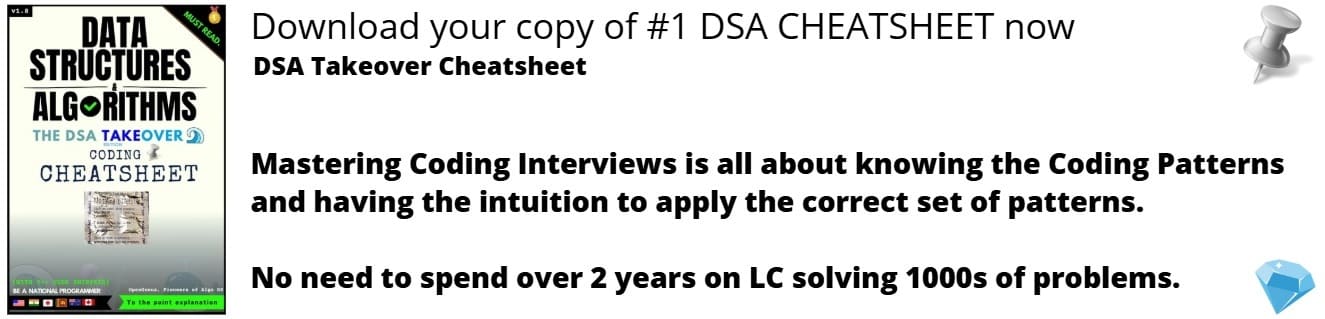
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Introduction
- HTMLAudioElement and Web Audio API
- Prerequisites
- Setting Up the HTML Structure
- Creating the Sound Buttons
- Styling the Sound Board
- Implementing the JavaScript Logic
- Explanation of JavaScript Code
- Testing the Sound Board
- Conclusion
Introduction
In today's digital age, interactive web applications have become increasingly popular, allowing users to engage with multimedia content in unique and exciting ways. One such application is a sound board, which enables users to play various sounds or audio clips at the click of a button. In this article at OpenGenus, we will guide you through the process of creating a dynamic sound board using HTML and JavaScript. This project will allow you to enhance your web development skills by incorporating user interactions and audio playback capabilities.
HTMLAudioElement and Web Audio API
To achieve audio playback in your dynamic sound board, you'll work with the HTMLAudioElement
interface and leverage the Web Audio API. This combination provides a powerful way to manipulate and control audio in web applications.
HTMLAudioElement Interface
The HTMLAudioElement
interface represents an <audio>
element in the DOM, providing methods and properties for controlling audio playback, volume, seeking, and more. By using the Audio
constructor, you can create instances of the HTMLAudioElement
to manage audio playback.
// Creating an HTMLAudioElement instance
const audio = new Audio('sound1.mp3');
// Playing the audio
audio.play();
// Pausing the audio
audio.pause();
// Adjusting the volume
audio.volume = 0.5; // Range: 0.0 (mute) to 1.0 (full volume)
Web Audio API
The Web Audio API offers a versatile system for creating and manipulating audio content directly within the browser. It's particularly useful for more advanced audio applications. While our sound board uses the HTMLAudioElement
, the Web Audio API is suitable for scenarios requiring lower-level audio processing.
// Using the Web Audio API to create an audio context
const audioContext = new AudioContext();
// Creating a buffer source node
const source = audioContext.createBufferSource();
// Loading audio data (replace with your own audio buffer)
const audioData = ...; // Load your audio data here
source.buffer = audioData;
// Connecting the source to the audio context's destination (speakers)
source.connect(audioContext.destination);
// Playing the audio using the Web Audio API
source.start();
Prerequisites
Before diving into the implementation, ensure you have a basic understanding of HTML, CSS, and JavaScript. You'll need a code editor of your choice and a modern web browser for testing your sound board.
Setting Up the HTML Structure
Begin by creating a new HTML file and structuring it to contain our sound board elements.
<!DOCTYPE html>
<html lang="en">
<head>
<!-- ... meta tags and title ... -->
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="soundboard">
<!-- Sound buttons will be added here -->
</div>
<script src="script.js"></script>
</body>
</html>
Creating the Sound Buttons
Next, let's add the buttons that users will click to play the sounds. Organize these buttons within an unordered list.
<!-- Inside the .soundboard div -->
<ul class="sound-list">
<li><button data-sound="sound1.mp3">Sound 1</button></li>
<li><button data-sound="sound2.mp3">Sound 2</button></li>
<!-- Add more buttons as needed -->
</ul>
Styling the Sound Board
Enhance the visual appeal of the sound board by applying basic styling. Create a styles.css
file and include the following styles.
/* styles.css */
.soundboard {
text-align: center;
margin: 50px auto;
}
.sound-list {
list-style: none;
padding: 0;
}
button {
padding: 10px 20px;
margin: 5px;
background-color: #3498db;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #2980b9;
}
Implementing the JavaScript Logic
Now comes the exciting part - adding interactivity to our sound board. Create a script.js
file and write the following code.
// script.js
const soundButtons = document.querySelectorAll('[data-sound]');
soundButtons.forEach(button => {
button.addEventListener('click', () => {
const soundFile = button.getAttribute('data-sound');
playSound(soundFile);
});
});
function playSound
(soundFile) {
const audio = new Audio(soundFile);
audio.play();
}
Explanation of JavaScript Code
In the JavaScript code, we begin by selecting all elements with the data-sound
attribute, which correspond to the sound buttons. Through a loop, we attach click event listeners to each button. When a button is clicked, the associated audio file's name is extracted from the data-sound
attribute. The playSound
function creates an Audio
object with the provided sound file and plays it, resulting in the audio clip being heard.
Testing the Sound Board
With the code in place, you can test your sound board by opening the HTML file in a web browser. Clicking on the sound buttons should play the corresponding audio clips, providing a delightful auditory experience.
Conclusion
This project has demonstrated how to incorporate user interactions and audio playback into a web application, while also introducing you to audio-related technologies in web development. As you continue your journey in web development, you can explore even more advanced audio features and create immersive experiences for your users. Share your creation with the world and let the sounds play!