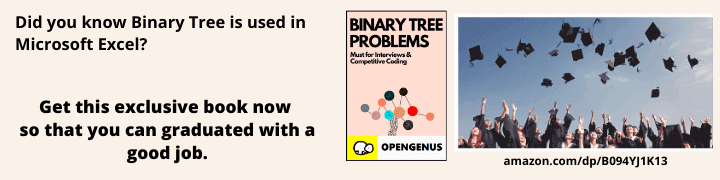
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we have explained how to redirect a webpage using JavaScript and natively in HTML. We have developed demos to demonstrate the ideas with code examples.
TABLE OF CONTENTS:-
- Introduction
- Redirect in HTML
- Redirect Using JavaScript
INTRODUCTION
Webpage (URL) redirection, which can also be called URL forwarding, is a popular procedure to give more than one URL address to a page, a form, or a whole Web site/application.
Redirects achieve a number of predetermined tasks which includes:
- Temporary redirects during site maintenance or downtime
- Permanent redirects to preserve existing links/bookmarks after changing the site's URLs, progress pages when uploading a file and others
A webpage redirect also inform search engines and webpage visitors that a particular webpage has permanently moved to a new location.
REDIRECT IN HTML
The purpose of this article to explain how to redirect a page to another page in HTML. We will highlight its application using examples. Sometimes you may need to redirect to another webpage because of the contents of a particular page has been moved to a new webpage.
Below are some of the method you can implement to achieve the target:
http-equiv attribute
of the meta tag in HTML. For a simple redirect from anHTML
page to another page, we can implement the tag and specify a link in the URL attribute. This is a client-side redirection and the browser will take the user to another page. It uses thehttp-equiv
attribute to provide an HTTP header for the value of the content attribute. The value òf the content in the attribute is set to seconds, for the page to be redirected.
Syntax:
<meta http-equiv="refresh" content="seconds; url=URL">
Code:
<!DOCTYPE HTML>
<html>
<head>
<title>
Redirect to Open Genus
</title>
<!-- meta tag to redirect to
iq.opengenus.org after few seconds -->
<meta http-equiv="refresh"
content="2;
url=https://iq.opengenus.org/">
</head>
<body>
<!-- Link to the destination page
in case the refresh does not work -->
You will be redirected to Open Genus in a moment.
<br>
If you are not redirected automatically,
<a href="https://iq.opengenus.org/">
click here
</a>.
</body>
</html>
Output:
Before
After couple of seconds
- Redirect using HTML Form Tag
In HTML you can redirect a webpage by the click of a button. In this scenario we will use a form. The form tag in HTML will be given an atrributeaction
, which allow the user to give the URL of the webpage where you want it redirected. The form tag in HTML also as an attribute calledmethod
. We will set the method attibute to: POSTJust set the method attribute to POST and then use mention the URL for the action attribute. Once the form is submitted it will be redirected to the URL and webpage c you submit the form, it will redirect you to the particular URL and webpage that coorelate with the URL.
Syntax:
<form action="URL" method="POST">
<input type="submit"/>
</form>
Code:
<html>
<head>
<title>Redirecting using Form Tags</title>
</head>
<body>
<form action="sample.com" method="POST">
<input type="submit"/>
</form>
</body>
</html>
The HTML form tags comes handy when you want to submit data during a user registration or log-in
- Redirect using HTML Anchor Tags
In a different settings where all you want to do is redirect the user, then anchor tags in HTML will serve the purpose. You will provide the reference or URL of the webpage that you want the user to redirect.
Syntax:
<a href="URL">TEXT</a>
Code:
<html>
<head>
<title>Redirecting using Anchor Tags</title>
</head>
<body>
<a href="https://www.sample.com">
Click Here to Redirect
</a>
</body>
</html>
Click Here to Redirect
We can also use a simple HTML button with onClick
property to redirect a webpage. For example:
<input type=button onClick="
parent.location='https:/iq.opengenus.org/'"
value='click here to visit home page'>
Also we can open in a new window. To open the webpage in a new window we can use onClick=parent.open()
<input type=button onClick="parent.open('https://iq.opengenus.org/')"
value='Home page in a new TAB' >
REDIRECT USING JAVASCRIPT:
In this article at OpenGenus, I am going to explain how to redirect a webpage using JavaScript. The application will be highlighted using examples. Sometimes you may need to redirect to another webpage because of the contents of a particular page has been moved to a new webpage.
Below are some of the method you can implement to achieve the target:
-
location.href: This is used to set or return the URL of the current page.
-
location.replace(): This is used to replace the current document with the specified one.
-
location.assign(): This is used for loading a new document.
-
location.href: This is used to set or return the URL of the current page.
Syntax:
location.href="URL"
**Code:**
<!DOCTYPE html>
<html>
<body>
<h2>Welcome to Open Genus</h2>
<p>This is the example of <i>location.href</i> way. </p>
<button onclick="myFunc()">Click me</button>
<!--script to redirect to another webpage-->
<script>
function myFunc() {
window.location.href = "https://iq.opengenus.org/";
}
</script>
</body>
</html>
Output:
Welcome to Open Genus
This is the example of location.href way.
<button onclick="myFunc()">Click me</button>
<!--script to redirect to another webpage-->
<script>
function myFunc() {
window.location.href = "https://iq.opengenus.org/";
}
</script>
- location.replace(): This is used to replace the current document with the one specified.
**Syntax: **
location.replace("URL")
Code:
<!DOCTYPE html>
<html>
<body>
<h2>Welcome to Open Genus</h2>
<p>This is the example of <i>location.replace</i> method. </p>
<button onclick="myFunc()">Click me</button>
<!--script to redirect to another webpage-->
<script>
function myFunc() {
location.replace("https://iq.opengenus.org/");
}
</script>
</body>
</html>
Output:
Welcome to Open Genus
This is the example of location.replace method.
<button onclick="myFunc()">Click me</button>
<!--script to redirect to another webpage-->
<script>
function myFunc() {
location.replace("https://iq.opengenus.org/");
}
</script>
- location.assign(): This is used for loading a new document.
Syntax:
location.assign("URL")
Code:
<!DOCTYPE html>
<html>
<head>
<title>Location assign() Method</title>
</head>
<body>
<h1>OpenGenus</h1>
<h2>Location assign() Method</h2>
<button onclick="load()">Click Here!</button>
<!-- Script to use Location assign() Method -->
<script>
function load() {
location.assign("https://iq.opengenus.org/100-interview-problems/");
}
</script>
</body>
</html>
Output:
OpenGenus
Location assign() Method
<!-- Script to use Location assign() Method -->
<script>
function load() {
location.assign("https://iq.opengenus.org/100-interview-problems/");
}
</script>
Its important to note that the output of all the stated methods will be similar expect location.replace()
approach. The approach removes the URL of the current document from the document history. It is advisable to use location.assign()
method if you want the option to navigate back to a specified page/document in a webpage.