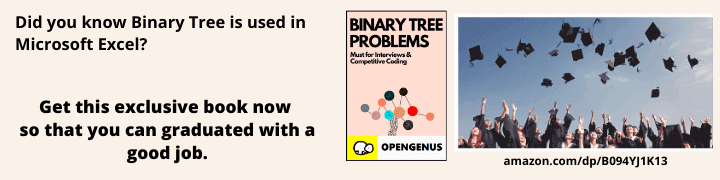
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Pagination is a common feature in web development that allows users to navigate through large sets of data or content. In this article at OpenGenus, we will explore how to implement pagination using HTML and CSS.
Pagination Basics
Pagination refers to the division of content into separate pages, where each page contains a fixed number of items. This is commonly used to display large sets of data such as search results, blog posts, or products in an online store.
The basic concept of pagination involves creating a series of links or buttons that allow users to navigate to the next or previous page. Additionally, links to the first and last pages may also be provided. Here's an example of what pagination might look like:
[1] [2] [3] ... [10] [Next]
In this example, the user is currently on page 1, and can click on the "Next" link to go to page 2. The ellipsis (...) represents additional pages that are not currently displayed.
Implementing Pagination in HTML
To implement pagination in HTML, we can use a combination of HTML tags and CSS styles. Let's start with the HTML.
First, we need to create a container element for our pagination links. We can use an unordered list (ul) element for this purpose:
<ul class="pagination">
<li><a href="#">1</a></li>
<li><a href="#">2</a></li>
<li><a href="#">3</a></li>
<li><span>...</span></li>
<li><a href="#">10</a></li>
<li><a href="#">Next</a></li>
</ul>
Here is the demo:
In this example, we have six list items (li). The first three contain links to the first three pages, the fourth contains a span element with an ellipsis, the fifth contains a link to the last page (10), and the last contains a link to the next page.
We can customize the appearance of our pagination links using CSS. Here's an example of some basic CSS styles:
.pagination {
display: flex;
list-style: none;
justify-content: center;
}
.pagination li {
margin: 0 5px;
}
.pagination a,
.pagination span {
display: block;
padding: 5px 10px;
border: 1px solid #ccc;
color: #333;
text-decoration: none;
}
.pagination a:hover {
background-color: #f5f5f5;
}
Here is a demo:
In this example, we're using Flexbox to center our pagination links within the container. We're also setting some basic styles for our links, including padding, borders, and colors. Finally, we're adding a hover effect to our links to make them more interactive.
Customizing Pagination Styles
Of course, the styles we've used here are just a starting point. You can customize the appearance of your pagination links in a variety of ways to match the look and feel of your website.
For example, you might want to change the colors of your links to match your brand colors, or add some animations to make the pagination more engaging. Here's an example of some more advanced CSS styles:
.pagination {
display: flex;
list-style: none;
justify-content: center;
}
.pagination li {
margin: 0 5px;
}
.pagination a,
.pagination span {
display: block;
padding: 5px 10px;
border: 1px solid #ccc;
color: #333;
text-decoration: none;
transition: background-color 0.2s ease;
}
.pagination a:first-child,
.pagination a:last-child {
border-radius: 5px;
}
In this example, we've added a transition effect to our links to make them fade in and out when the user hovers over them. We've also added some additional styles, including border radius for the first and last links, and a custom background color for the active link.
Implementing Pagination with Dynamic Data
In many cases, pagination is used to display large sets of dynamic data, such as search results or products in an online store. In these cases, we need to be able to generate our pagination links dynamically based on the number of items in our dataset.
Here's an example of how we might generate pagination links dynamically using JavaScript:
var pagination = document.querySelector('.pagination');
var itemsPerPage = 10;
var totalItems = 100;
var totalPages = Math.ceil(totalItems / itemsPerPage);
for (var i = 1; i <= totalPages; i++) {
var li = document.createElement('li');
var link = document.createElement('a');
link.href = '#';
link.textContent = i;
li.appendChild(link);
pagination.appendChild(li);
}
In this example, we're calculating the total number of pages based on the number of items per page and the total number of items in our dataset. We're then using a for loop to generate a list item and link element for each page, and appending them to our pagination container.
Of course, in a real-world scenario, we would likely be fetching our data from a server using AJAX or a similar technology, and generating our pagination links dynamically based on the response.
Conclusion
Pagination is an essential feature for displaying large sets of data or content on a website. By using a combination of HTML and CSS, we can create stylish and functional pagination links that allow users to navigate through our content with ease.
Whether you're building a search engine, an e-commerce site, or a content-heavy website, pagination is an essential tool that can help you improve user experience and make your website more user-friendly. So why not give it a try? With a little bit of HTML, CSS, and JavaScript, you can implement pagination on your website today.