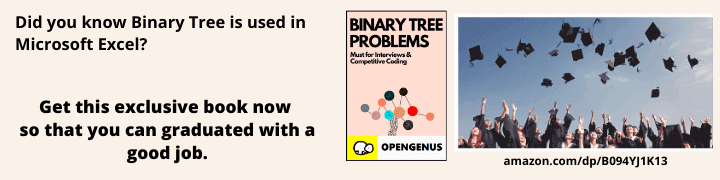
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore star rating systems in HTML, exploring how to implement them using HTML, CSS and their use cases, and examples of websites that utilize this concept.
Table of contents:
- Introduction
- Implementing Star Rating in HTML
- Styling the Star Rating with CSS
- Breakdown of CSS code
- Use Cases and Other Applications
- Examples of Websites Utilizing Star Rating Systems
- Conclusion
Introduction:
In the digital age, user ratings have become an essential component for evaluating the quality and popularity of products, services, and content. Star ratings, in particular, have gained widespread recognition as a user-friendly and intuitive way to express opinions and provide feedback.
Implementing Star Rating in HTML:
To create a star rating system in HTML, CSS, you need to consider a few key elements. First, let's focus on the HTML structure. The basic idea is to represent each star as a clickable element, allowing users to interact with them and indicate their rating. Here's a sample HTML structure for a five-star rating system:
<html>
<head>
<meta charset="UTF-8">
<link rel="stylesheet" type="text/css" href="style.css">
<title>Star rating using pure CSS</title>
</head>
The code begins with the HTML <head>
section, where the character encoding is set to UTF-8 using the <meta>
tag. This ensures proper rendering of characters and symbols. The next line includes an external CSS file named "style.css" using the <link>
tag. This file is responsible for styling the star rating elements. Lastly, the <title>
tag specifies the title of the HTML page as "Star rating using pure CSS."
<body>
<div class="rate">
<input type="radio" id="star5" name="rate" value="5" />
<label for="star5" title="text">5 stars</label>
<input type="radio" id="star4" name="rate" value="4" />
<label for="star4" title="text">4 stars</label>
<input type="radio" id="star3" name="rate" value="3" />
<label for="star3" title="text">3 stars</label>
<input type="radio" id="star2" name="rate" value="2" />
<label for="star2" title="text">2 stars</label>
<input type="radio" id="star1" name="rate" value="1" />
<label for="star1" title="text">1 star</label>
</div>
</body>
Inside the <body>
section, we have a container <div>
element with the class "rate." This container wraps the star rating elements.
Within the container, there are five sets of input radio buttons and associated labels. Each input represents a star rating option, while the corresponding label provides a textual representation of the rating.
For example, the first set consists of an input with id="star5"
, name="rate"
, and value="5"
. This represents a rating option of 5 stars. The associated label has for="star5"
to establish the connection between the input and the label. The title="text"
attribute can be used to display additional text when hovering over the rating.
The subsequent sets follow the same pattern, representing ratings from 4 stars down to 1 star.
Styling the Star Rating with CSS:
The following is the CSS code of the mentioned style.css file:
{
margin: 0;
padding: 0;
}
.rate {
float: left;
height: 46px;
padding: 0 10px;
}
.rate:not(:checked) > input {
position:absolute;
top:-9999px;
}
.rate:not(:checked) > label {
float:right;
width:1em;
overflow:hidden;
white-space:nowrap;
cursor:pointer;
font-size:30px;
color:#ccc;
}
.rate:not(:checked) > label:before {
content: '★ ';
}
.rate > input:checked ~ label {
color: #ffc700;
}
.rate:not(:checked) > label:hover,
.rate:not(:checked) > label:hover ~ label {
color: #deb217;
}
.rate > input:checked + label:hover,
.rate > input:checked + label:hover ~ label,
.rate > input:checked ~ label:hover,
.rate > input:checked ~ label:hover ~ label,
.rate > label:hover ~ input:checked ~ label {
color: #c59b08;
}
/* CSS source: allenhe from codepen */
Breakdown of CSS code
Now, let's see what the code actually does step by step:
* {
margin: 0;
padding: 0;
}
The asterisk (*
) selector selects all elements on the page. In this code, it sets the margin and padding of all elements to zero. This ensures that there are no default margins or padding interfering with the layout of the rating system.
.rate {
float: left;
height: 46px;
padding: 0 10px;
}
The .rate
selector targets the container <div>
element with the class "rate." It sets the container's float to left, allowing other content to flow around it. The height is set to 46 pixels, providing a fixed height for the rating system. The padding is set to 0 on the top and bottom and 10 pixels on the left and right, creating space around the rating elements.
.rate:not(:checked) > input {
position:absolute;
top:-9999px;
}
This selector targets the input elements inside the .rate
container that are not checked (:not(:checked)
). It positions those input elements absolutely, moving them off the screen with top: -9999px
. This technique hides the radio buttons visually but keeps them functional for user interaction.
.rate:not(:checked) > label {
float:right;
width:1em;
overflow:hidden;
white-space:nowrap;
cursor:pointer;
font-size:30px;
color:#ccc;
}
This selector targets the labels associated with the unchecked input elements. It floats the labels to the right, aligning them horizontally. The width is set to 1em, restricting the label width to the width of one character. This ensures that only one star symbol is visible. The overflow
property is set to hidden, preventing any overflow from being visible. The white-space
property is set to nowrap, preventing line breaks within the labels. The cursor is set to a pointer, indicating that the labels are clickable. The font size is set to 30 pixels, and the color is set to #ccc
, providing a gray color for the labels.
.rate:not(:checked) > label:before {
content: '★ ';
}
This selector targets the labels associated with the unchecked input elements and adds a star symbol before the label's content. The star symbol is defined using the content
property and the Unicode representation for a star (★
).
.rate > input:checked ~ label {
color: #ffc700;
}
This selector targets the labels that come after a checked input element. It sets the color of those labels to #ffc700
, providing a yellow color. This indicates the selected rating.
.rate:not(:checked) > label:hover,
.rate:not(:checked) > label:hover ~ label {
color: #deb217;
}
This selector targets the labels associated with the unchecked input elements when hovering over them. It sets the color of those labels to #deb217
, providing a darker yellow color. This creates a visual effect to indicate the potential rating when hovering.
.rate > input:checked + label:hover,
.rate > input:checked + label:hover ~ label,
.rate > input:checked ~ label:hover,
.rate > input:checked ~ label:hover ~ label,
.rate > label:hover ~ input:checked ~ label {
color: #c59b08;
}
This selector targets the labels associated with the checked input element when hovering over them, as well as labels before and after the checked label. It sets the color of these labels to #c59b08
, providing an orange color. This creates a visual effect to indicate the potential rating when hovering over checked stars.
Overall, this CSS code combines various selectors, pseudo-classes, and properties to style the star rating system, creating visual cues and interactions for the user.
Use Cases and Other Applications:
Star rating systems find applications in various domains, such as e-commerce, movie reviews, restaurant ratings, and content aggregators. They empower users to express their opinions easily and help others make informed decisions. Additionally, star ratings can be utilized in creative ways beyond their traditional application. For example, some websites use them to gamify user engagement by awarding badges or unlocking features based on accumulated ratings.
Examples of Websites Utilizing Star Rating Systems:
Numerous websites effectively incorporate star rating systems to gather user feedback. One notable example is Amazon, the popular e-commerce platform. Amazon employs star ratings to allow customers to review and rate products, aiding potential buyers in making purchasing decisions. The star rating system acts as a quick visual reference, complemented by detailed reviews and descriptions.
Another website that has taken star ratings to the next level is IMDb (Internet Movie Database). IMDb utilizes star ratings to evaluate movies and TV shows. Users can rate films on a scale of one to ten stars, contributing to the overall rating of a particular title. This user-driven approach provides valuable insights to movie enthusiasts and helps them decide which films to watch.
Conclusion:
Star rating systems in HTML, CSS provide an intuitive and user-friendly way for individuals to express their opinions and evaluate various products and services. By implementing this system, websites can enhance user engagement, provide valuable feedback, and aid decision-making processes. Whether it's e-commerce platforms like Amazon or movie databases like IMDb, star ratings have become a ubiquitous feature in the digital landscape, contributing to a more informed and interactive online experience.