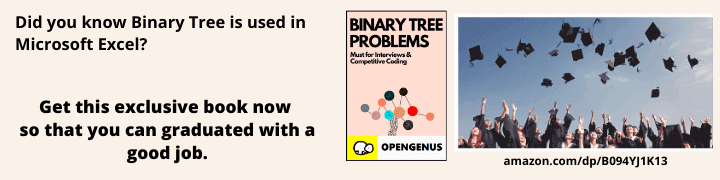
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Both javascript:void(0)
and #
are commonly used in the href attribute of HTML links, but they have different meanings and purposes. In this article, we have explored both in depth with code examples.
Table of contents:
Javascript:void(0)
and#
in href in HTML links- Code Examples of
Javascript:void(0)
and#
- Advanced use-cases of
Javascript:void(0)
and#
Javascript:void(0)
and #
in href in HTML links
javascript:void(0)
is used to indicate that the link does not have a destination URL and that its behavior is defined by JavaScript code. When a user clicks on a link with javascript:void(0)
in the href attribute, nothing happens by default. However, you can use JavaScript to define an action for the link, such as displaying a popup or executing a function. This technique is often used in web applications that rely heavily on JavaScript to provide interactivity and functionality.
On the other hand, the # symbol is used to create an internal link to a specific element on the same page. When a user clicks on a link with # in the href attribute, the browser will scroll to the element with the matching ID. For example, you can create a table of contents at the top of a long article and link each item to the corresponding section further down the page. This can help users navigate the content more easily.
In summary, javascript:void(0)
is used to define a link's behavior through JavaScript, while # is used to create internal links within the same page.
Here are some examples of how to use javascript:void(0)
and # in the href attribute of HTML links:
Code Examples of Javascript:void(0)
and #
Example using javascript:void(0):
<a href="javascript:void(0);" onclick="alert('Hello, world!')">Click me</a>
In this example, the link has no destination URL (javascript:void(0))
, but when the user clicks on it, the onclick
attribute triggers a JavaScript alert that displays a message.
Example using #:
<h2 id="section1">Section 1</h2>
<p>Some content goes here...</p>
<a href="#section1">Go to Section 1</a>
In this example, the h2 element has an id attribute that uniquely identifies it as "section1". The link has a href attribute with the value #section1, which creates an internal link to that element. When the user clicks on the link, the browser will scroll to the element with the matching ID.
Note that # can also be used as a placeholder in the href attribute for links that don't have a destination URL yet, but should be replaced with a valid URL later. For example:
<a href="#">Coming soon</a>
In this case, the link has no destination URL (#), but the text suggests that it will be added later.
Advanced use-cases of Javascript:void(0)
and #
Here are some advanced techniques that use javascript:void(0) and # in the href attribute of HTML links:
Advanced example using javascript:void(0):
<a href="javascript:void(0);" onclick="document.querySelector('#myForm').submit();">Submit form</a>
<form id="myForm" action="/submit" method="post">
<!-- form fields go here -->
</form>
In this example, the link has no destination URL (javascript:void(0))
, but when the user clicks on it, the onclick attribute triggers a JavaScript function that submits a form with the ID myForm. This can be useful in cases where you want to provide a submit button outside of the form, or if you want to customize the submit behavior with JavaScript.
Advanced example using #:
<a href="#" class="toggle-panel">Toggle panel</a>
<div id="myPanel" class="panel">
<!-- panel content goes here -->
</div>
<script>
document.querySelector('.toggle-panel').addEventListener('click', function(event) {
event.preventDefault();
document.querySelector('#myPanel').classList.toggle('active');
});
</script>
In this example, the link has a href attribute with the value #, which creates an internal link that doesn't do anything by default. However, when the user clicks on the link, a JavaScript function attached to the click event toggles the active class on an element with the ID myPanel. This can be useful for creating expandable/collapsible sections of a webpage.
Note that in both cases, it's important to use JavaScript responsibly and to make sure your website is accessible to all users, including those who may have JavaScript disabled or use assistive technologies.
An advanced example using # in the href attribute of an HTML link:
<nav>
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
</ul>
</nav>
<main>
<section id="section1">
<h2>Section 1</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</section>
<section id="section2">
<h2>Section 2</h2>
<p>Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
</section>
<section id="section3">
<h2>Section 3</h2>
<p>Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.</p>
</section>
</main>
<script>
const sections = document.querySelectorAll('main section');
const navLinks = document.querySelectorAll('nav a');
function setActiveSection() {
const currentScroll = window.pageYOffset;
let currentSection = sections[0];
sections.forEach((section) => {
if (section.offsetTop <= currentScroll) {
currentSection = section;
}
});
navLinks.forEach((link) => {
if (link.hash === `#${currentSection.id}`) {
link.classList.add('active');
} else {
link.classList.remove('active');
}
});
}
window.addEventListener('scroll', setActiveSection);
setActiveSection();
</script>
In this example, the links in the navigation menu use the # symbol to create internal links to sections on the same page. When the user clicks on a link, the browser scrolls to the corresponding section.
Additionally, the script at the bottom of the page uses JavaScript to dynamically add and remove the active class on the navigation links based on the user's scroll position. As the user scrolls through the page, the script determines which section is currently visible and applies the active class to the corresponding link in the navigation menu. This provides visual feedback to the user and helps them keep track of their position on the page.
Using javascript:void(0)
to prevent default link behavior:
In some cases, you may want to create a link that doesn't navigate to a new page or change the URL in the address bar, but instead triggers some JavaScript code to run. To accomplish this, you can set the href attribute to javascript:void(0) and use an event listener to handle the click event. Here's an example:
javascript <a href="javascript:void(0);" onclick="alert('Hello, world!')">Click me</a>
In this example, when the user clicks on the link, an onclick event is triggered, which displays an alert box with the message "Hello, world!".
Using # to create anchor links:
The # symbol is often used to create anchor links, which allow users to navigate to specific sections of a page. To create an anchor link, you first need to add an id attribute to the section of the page you want to link to, like this:
<section id="section1">
<h2>Section 1</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</section>
Then, you can create a link that points to that section by setting the href attribute to # followed by the id of the section:
<a href="#section1">Go to section 1</a>
In this example, when the user clicks on the link, the browser will scroll down to the section of the page with the id of section1.
Using # and JavaScript to create smooth scrolling:
While anchor links are useful for navigating to specific sections of a page, the default behavior can be jarring and abrupt. To create a smoother scrolling effect, you can use JavaScript to animate the scroll instead of relying on the browser's default behavior. Here's an example:
<a href="#section1" class="smooth-scroll">Go to section 1</a>
<section id="section1">
<h2>Section 1</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p>
</section>
<script>
const smoothScrollLinks = document.querySelectorAll('.smooth-scroll');
smoothScrollLinks.forEach(link => {
link.addEventListener('click', event => {
event.preventDefault();
const targetId = link.getAttribute('href');
const targetElement = document.querySelector(targetId);
targetElement.scrollIntoView({
behavior: 'smooth'
});
});
});
</script>
In this example, the link uses # to create an anchor link to the section with the id of section1, but instead of relying on the browser's default behavior, a JavaScript function attached to the click event animates the scroll using the scrollIntoView method with the behavior option set to smooth. This provides a more elegant and polished user experience.
With this article at OpenGenus, you must have the complete idea of Javascript:void(0) and # in href in HTML links.
Note that while these techniques can be powerful and useful, it's important to use them responsibly and to make sure your website is accessible to all users, including those who may have JavaScript disabled or use assistive technologies.