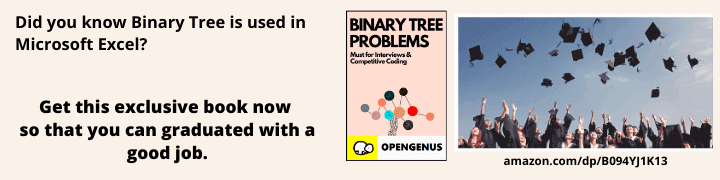
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents:
- Introduction
- Understanding Tooltips in HTML
- Basic Syntax
- Text and Formatting
- Customization
- Practical Examples and Use Cases
- Image Descriptions
- Hyperlink Explanations
- Form Field Assistance
- Best Practices for Implementing Tooltips
- Concise and Relevant
- Accessibility Considerations
- Avoid Overusing
- Responsive Design
- Advanced Tooltip Libraries
- Tippy.js
- Tooltipster
- Popper.js
- Conclusion
Introduction
In the vast realm of web development, HTML (Hypertext Markup Language) plays a crucial role in structuring and presenting content. One often overlooked yet highly valuable feature in HTML is the tooltip. Tooltips provide additional information or descriptions when users hover over an element, enriching the user experience and improving accessibility.
In this comprehensive guide at OpenGenus, we will delve into the world of tooltips in HTML, exploring their implementation, customization, and best practices.
I. Understanding Tooltips in HTML
1. Basic Syntax
In HTML, tooltips are created using the "title" attribute, which is added to an HTML element. For example, if you want to add a tooltip to a paragraph, you would use the following code:
<p title="This is a tooltip">Join OpenGenus for better oportunity</p>
Join OpenGenus for better oportunity (Hover here)
When the user hovers over the paragraph, a small popup containing the tooltip text will appear.2. Text and Formatting
Tooltips can contain plain text or even formatted content. HTML tags can be used within the tooltip text to apply formatting, such as bold or italic styles. For instance:
<p title="<strong>Important Tip:</strong> Hover over me to see the tooltip!">...</p>
📤 Output
...
This would display the tooltip with the "Important Tip" text in bold.
3. Customization
While the default appearance of tooltips may vary across browsers, their styling can be customized to align with the design of your web page. CSS (Cascading Style Sheets) can be employed to alter various properties such as background color, text color, font size, and more.
<style>
p[title] {
background-color: #F5F5F5;
color: #333;
font-size: 14px;
}
</style>
This CSS snippet targets all paragraphs with a "title" attribute, applying a specific background color, text color, and font size to their tooltips.
II. Practical Examples and Use Cases
1. Image Descriptions
Tooltips can be used to provide descriptive text for images, ensuring accessibility for users with visual impairments. By adding the "title" attribute to the "img" tag, you can display alternative text or additional details when hovering over the image.
<img src="example.jpg" alt="A beautiful landscape" title="Explore the serene beauty of nature in this breathtaking landscape." />
Hover on the image below
2. Hyperlink Explanations
Tooltips can enhance the understanding of hyperlinks by providing brief explanations. For instance, when hovering over a link to a product, you can display a tooltip with details about the item.
<a href="product.html" title="Discover our latest product, designed to revolutionize your daily routine.">Product Details (Hover)</a>
📤 Output
Product Details (hover here)
This tooltip provides a glimpse into the product's features without the need to navigate away from the current page.
3. Form Field Assistance
Tooltips can guide users when filling out forms by offering clarifications or examples for specific fields. For example, a tooltip can provide instructions on how to format a phone number or specify the expected input.
<input type="text" title="Please enter a valid phone number (e.g., +1 123-456-7890)" />
📤 Output
This tooltip assists users in entering the correct format for the phone number, minimizing errors.
III. Best Practices for Implementing Tooltips
1. Concise and Relevant
To ensure tooltips are helpful and don't overwhelm users, keep the content concise and relevant. Focus on providing essential information or clarifying the context of the element.
Here's an example that demonstrates the principle of keeping tooltips concise and relevant:
<!DOCTYPE html>
<head>
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltiptext {
visibility: hidden;
width: 200px;
background-color: #333;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 8px;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
transform: translateX(-50%);
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltiptext {
visibility: visible;
opacity: 1;
}
</style>
</head>
<body>
<div class="tooltip">
Hover over me
<span class="tooltiptext">Click to learn more about our product</span>
</div>
</body>
</html>
**📤 Output**
By keeping the tooltip content concise and directly relevant to the element it is associated with, users can quickly grasp the purpose or context without being overwhelmed by excessive information. In this case, the tooltip succinctly informs the user that clicking will provide further details about the product, encouraging engagement without overwhelming them with unnecessary details.
2. Accessibility Considerations
Ensure that tooltips are accessible to all users, including those who rely on assistive technologies. Test tooltips with screen readers to ensure the information is conveyed effectively.
<!DOCTYPE html>
<html>
<head>
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltiptext {
visibility: hidden;
width: 200px;
background-color: #333;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 8px;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
transform: translateX(-50%);
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltiptext {
visibility: visible;
opacity: 1;
}
</style>
</head>
<body style="border: 1px black solid;">
<div class="tooltip">
Hover over me
<span class="tooltiptext">Click to learn more about our product</span>
</div>
<script>
// Ensure tooltips are accessible to screen readers
const tooltips = document.querySelectorAll('.tooltip');
tooltips.forEach((tooltip) => {
tooltip.addEventListener('focus', () => {
tooltip.classList.add('active');
});
tooltip.addEventListener('blur', () => {
tooltip.classList.remove('active');
});
});
</script>
</body>
</html>
📤 Output
To ensure accessibility, we add event listeners to the tooltip element. When the tooltip receives focus (e.g., through keyboard navigation), it adds the "active" class, indicating to screen readers that the tooltip is active and should be announced. When the tooltip loses focus, it removes the "active" class.
By testing tooltips with screen readers, developers can ensure that the information conveyed by the tooltip is effectively communicated to users who rely on assistive technologies. This consideration helps make tooltips inclusive and accessible to a broader range of users, ensuring a positive user experience for everyone.
3. Avoid Overusing:
While tooltips can be beneficial, avoid excessive use, as they can become distracting or irritating. Reserve tooltips for situations where additional information is genuinely needed.
<!DOCTYPE html>
<html>
<head>
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltiptext {
visibility: hidden;
width: 200px;
background-color: #333;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 8px;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
transform: translateX(-50%);
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltiptext {
visibility: visible;
opacity: 1;
}
</style>
</head>
<body>
<p>
<span class="tooltip">
Hover over me
<span class="tooltiptext">This is a tooltip.</span>
</span>
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis euismod varius diam, ac dapibus urna interdum vel.
<span class="tooltip">
Hover over me
<span class="tooltiptext">This is another tooltip.</span>
</span>
Vestibulum in ex at tortor maximus blandit ut ut turpis.
</p>
</body>
</html>
📤 Output
<!DOCTYPE html>
Hover over me This is a tooltip. Lorem ipsum dolor sit amet, consectetur adipiscing elit. Duis euismod varius diam, ac dapibus urna interdum vel. Hover over me This is another tooltip. Vestibulum in ex at tortor maximus blandit ut ut turpis.
In this example, we have two tooltips associated with different sections of a paragraph. The tooltips appear when the user hovers over the corresponding "Hover over me" text.
By using tooltips sparingly and only in situations where additional information is genuinely needed, we avoid overwhelming the user with excessive tooltips. Each tooltip serves a specific purpose, providing relevant information when hovering over specific elements within the paragraph.
This approach ensures that tooltips remain useful and do not become distracting or irritating for users. By using tooltips judiciously, developers can strike a balance between providing valuable supplementary information and maintaining a clean and uncluttered user interface.
4. Responsive Design:
Consider the responsiveness of tooltips, particularly for mobile devices. As hover interactions are not available on touchscreens, provide alternative ways to access the tooltip content, such as a tap or long-press gesture.
<!DOCTYPE html>
<head>
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltiptext {
visibility: hidden;
width: 200px;
background-color: #333;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 8px;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
transform: translateX(-50%);
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltiptext,
.tooltip.show-tooltip .tooltiptext {
visibility: visible;
opacity: 1;
}
</style>
</head>
<body>
<div class="tooltip" ontouchstart="">
Tap or hover over me
<span class="tooltiptext">This is a tooltip.</span>
</div>
<script>
const tooltips = document.querySelectorAll('.tooltip');
tooltips.forEach((tooltip) => {
tooltip.addEventListener('click', (event) => {
event.preventDefault();
tooltip.classList.toggle('show-tooltip');
});
tooltip.addEventListener('touchstart', (event) => {
event.preventDefault();
tooltip.classList.toggle('show-tooltip');
});
});
</script>
</body>
</html>
📤 Output
In this example, we have a tooltip associated with a
To ensure responsiveness, we add event listeners for both click and touch events. When the user clicks or taps on the element, the tooltip's visibility toggles. By using the show-tooltip class, we ensure that the tooltip remains visible even when the user taps outside of it to read the content.
This approach provides an alternative way to access tooltip content on touchscreens, accommodating mobile users who cannot rely on hover interactions. By considering the responsiveness of tooltips and providing alternative access methods, we create a seamless and user-friendly experience across different devices and interaction modes.
IV. Advanced Tooltip Libraries
While basic tooltips can be implemented using HTML alone, numerous JavaScript libraries offer advanced features and customization options. Some popular libraries include:
- Tippy.js: A highly customizable tooltip library with a wide range of features. lets discuss Tippy in detail with examples.
Tippy.js stands out as a highly versatile and customizable tooltip library, offering an extensive range of features that go beyond the capabilities of basic HTML tooltips. With Tippy.js, developers can effortlessly create tooltips with advanced functionality, such as interactive elements, animations, and various positioning options. Its comprehensive customization options allow developers to fine-tune every aspect of the tooltip's appearance and behavior, making it an ideal choice for projects that demand precise control and attention to detail. Whether you need tooltips that adapt to different screen sizes or tooltips with complex interactions, Tippy.js empowers developers to create exceptional tooltip experiences tailored to their specific needs.
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="tippy.css">
</head>
<body>
<button id="myButton">Hover over me</button>
<script src="tippy.js"></script>
<script>
// Initialize Tippy.js tooltip
tippy('#myButton', {
content: 'Click me to perform an action!',
placement: 'right',
arrow: true,
animation: 'scale',
duration: 300,
});
</script>
</body>
</html>
📤 Output
In this example, we have a button with the ID "myButton." By including the Tippy.js library and initializing it with the tippy() function, we can enhance the button with a customized tooltip. The content option specifies the tooltip text, which states "Click me to perform an action!" The placement option determines where the tooltip will appear relative to the button (in this case, it is set to "right"). The arrow option adds an arrow to the tooltip, indicating its association with the button. We've also added an animation effect using the animation option, which scales the tooltip when it appears. Lastly, the duration option sets the duration of the animation to 300 milliseconds.
This is just a basic example, and Tippy.js provides numerous other options and configurations to create tooltips with even more advanced functionality and styling.
- Tooltipster: Provides flexible and visually appealing tooltips with support for various interactions.
- Popper.js: A powerful library for creating tooltips, popovers, and dropdown menus with robust positioning capabilities.
Conclusion
Tooltips in HTML are a simple yet effective way to enhance user experience, provide additional information, and improve accessibility. By leveraging the "title" attribute and CSS styling, developers can create tooltips that enrich the content and design of web pages. Understanding the best practices and use cases for tooltips empowers developers to optimize their implementation and create engaging user interfaces. So, the next time you embark on a web development journey, remember the power of tooltips and make your designs shine!