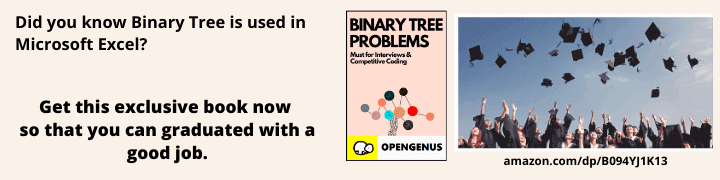
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Infinite scrolling is a popular UI pattern used on many websites and apps. It allows content to be loaded continuously as the user scrolls down the page, eliminating the need for pagination or clicking "next" buttons. Infinite scrolling can be implemented using CSS and HTML, with the help of JavaScript to handle the scrolling events and fetch new data.
In this article, we'll explore how to implement infinite scrolling in CSS and HTML, and provide some code examples.
How Infinite Scrolling Works
Infinite scrolling works by loading more content as the user scrolls down the page. This can be achieved by detecting when the user reaches the bottom of the page and then fetching new data from the server. The new data is then added to the existing content, creating the illusion of infinite scrolling.
To implement infinite scrolling in CSS and HTML, we'll use the overflow property to create a scrollable container for our content. We'll also use JavaScript to detect when the user reaches the bottom of the container and fetch new data.
Creating the Scrollable Container
The first step in implementing infinite scrolling is to create a scrollable container for our content. We'll use the overflow property to make the container scrollable, and set a fixed height for the container to limit the amount of content that's displayed at once.
Here's an example of how to create a scrollable container in CSS:
#container {
height: 500px;
overflow: auto;
}
In this example, we've created a container with an id of "container" and set a fixed height of 500 pixels. We've also set the overflow property to "auto" to create a scrollable container. This means that if the content within the container exceeds the height of the container, the container will display a scrollbar to allow the user to scroll down and see the rest of the content.
Adding Content to the Container
Once we've created the scrollable container, we can start adding content to it. In this example, we'll use an unordered list to display a list of items.
<div id="container">
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li>Item 5</li>
<li>Item 6</li>
<li>Item 7</li>
<li>Item 8</li>
<li>Item 9</li>
<li>Item 10</li>
</ul>
</div>
In this example, we've added an unordered list to the container, with ten list items. This will serve as our initial content that's displayed when the page loads.
Detecting When the User Reaches the Bottom
The next step in implementing infinite scrolling is to detect when the user reaches the bottom of the container. We'll use JavaScript to detect when the user scrolls to the bottom of the container, and then fetch new data from the server.
Here's an example of how to detect when the user reaches the bottom of the container in JavaScript:
const container = document.getElementById('container');
container.addEventListener('scroll', () => {
if (container.scrollTop + container.clientHeight >= container.scrollHeight) {
// Fetch new data and add it to the container
}
});
In this example, we're attaching a scroll event listener to the container element using the addEventListener() method. When the user scrolls within the container, the event listener checks if the sum of the container's scrollTop and clientHeight properties is greater than or equal to the container's scrollHeight property. If it is, this means that the user has scrolled to the bottom of the container, and we can fetch new data from the server and add it to the container.
Fetching New Data and Adding it to the Container
Now that we've detected when the user reaches the bottom of the container, we can fetch new data from the server and add it to the container. This can be done using JavaScript's fetch() method to make an AJAX request to the server, and then appending the new data to the existing content in the container.
Here's an example of how to fetch new data and add it to the container:
const container = document.getElementById('container');
container.addEventListener('scroll', () => {
if (container.scrollTop + container.clientHeight >= container.scrollHeight) {
fetch('/api/data')
.then(response => response.json())
.then(data => {
// Add new data to the container
const list = document.querySelector('#container ul');
data.forEach(item => {
const li = document.createElement('li');
li.textContent = item;
list.appendChild(li);
});
});
}
});
In this example, we're using the fetch() method to make a request to an API endpoint (/api/data) to fetch new data. We're then using the json() method to parse the response as JSON.
Once we have the new data, we're adding it to the existing content in the container by creating new list items for each item in the data and appending them to the unordered list in the container.
Limiting the Number of Items to Fetch
Infinite scrolling can be resource-intensive if we fetch and display an unlimited number of items. To prevent this, we can limit the number of items that are fetched and displayed at once.
One way to do this is to add a limit parameter to the API endpoint and specify the number of items to fetch. We can also keep track of the number of items that have been fetched and stop fetching new items once we reach a certain limit.
Here's an example of how to limit the number of items that are fetched and displayed:
const container = document.getElementById('container');
let limit = 10;
let offset = 0;
container.addEventListener('scroll', () => {
if (container.scrollTop + container.clientHeight >= container.scrollHeight) {
fetch(`/api/data?limit=${limit}&offset=${offset}`)
.then(response => response.json())
.then(data => {
// Add new data to the container
const list = document.querySelector('#container ul');
data.forEach(item => {
const li = document.createElement('li');
li.textContent = item;
list.appendChild(li);
});
// Update the offset and check if we've reached the limit
offset += data.length;
if (data.length < limit) {
container.removeEventListener('scroll');
}
});
}
});
In this example, we're adding a limit parameter to the API endpoint and specifying the number of items to fetch. We're also keeping track of the offset (the number of items that have already been fetched) and updating it each time we fetch new data.
We're also checking if the number of items in the new data is less than the limit. If it is, this means that we've reached the end of the data, so we're removing the scroll event listener to stop fetching new data.
Conclusion
Infinite scrolling is a popular UI pattern that can be implemented using CSS and HTML, with the help of JavaScript to handle the scrolling events and fetch new data. By creating a scrollable container, detecting when the user reaches the bottom of the container, and fetching new data and adding it to the container, we can create a seamless browsing experience for our users.
It's important to note that infinite scrolling should be used carefully and in the appropriate context. In some cases, it may not be the best option for displaying content, especially if the content is complex or requires a lot of processing power. It's also important to consider the impact on performance and user experience, as infinite scrolling can be resource-intensive if not implemented properly.
In conclusion, infinite scrolling is a powerful and useful technique that can be used to enhance the user experience on websites and web applications. With the right implementation, it can provide a seamless browsing experience that keeps users engaged and encourages them to explore more content.