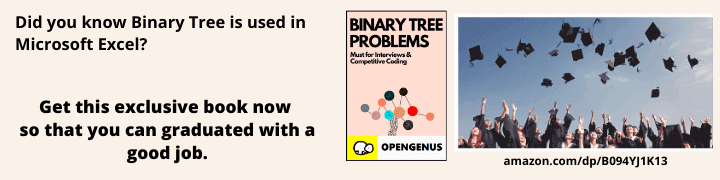
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of contents:
- What are CSS Preprocessors
- How to compile the code
- Different Syntaxes
- Why use a Preprocessor
- Features of a Preprocessor
What are CSS Preprocessors?
CSS Preprocessors are tools that add more functionality to normal CSS by using its own unique syntax. The browser can't read preprocessors so the code has to be compiled into vanila CSS which the browser can read.
Most CSS preprocessors have features that don't exist in normal CSS such as variables, loops, if/else statements etc. There are many CSS preprocessors but one of the most popular ones used is SASS which is the one I will talking about through this article and giving code examples to.
How to compile the code?
There are many ways to compile the code such as you can use node.js to run a compiler that will monitor files and compile them automatically for us. Another way is by using Gulp which is an open source JavaScript toolkit. Essentiallys Gulp allows you to automate processes and run repetitive tasks. It only needs to read a file once and then process it through multiple tasks and finally write the output file.
Other than these methods one of the most effective ways is using an extension in vscode called Live SASS Compiler which will help you to compile your SASS files to CSS files in real time. In order to install this you need to go in the vscode enxtensions tab and type Live SASS Compiler and click install.
Different Syntaxes
SCSS
There are two syntaxes which are supported by SASS, the most commonly known is SCSS. This uses the .SCSS file and is basically a superset of CSS, which essentially means that all valid CSS is valid SCSS as well and because of this similarity it's the easiest syntax to get used to and the most popular.
Indented Syntax
The other supported syntax is the Indented Syntax and this uses the file .SASS and this was the original syntax for SASS. Indented Syntax supports all of the same features as SCSS but it uses indentation instead of curly braces and semicolons and a few other minor differences.
Why use a Preprocessor?
The DRY Principle
One of the reasons why you should use a preprocessor is because it implements the principles of DRY. DRY stands for Don't Repeat Yourself and stops as much repetition of code as possible and uses better developer practices in the software development lifecycle. A programmer should always try to avoid rewriting code within their application. This is done by taking a piece of code that has been repeated in the app and turn it into a reusable helper function or variable. CSS Preprocessors allows you to keep your code DRY because they give you access to things like variables, loops and if/else statements which allow you to replace repeated css rules with something reusable.
Using a preprocessor in your software development workflow can significantly improve your code's maintainability and readability. DRY principles ensure that your codebase is concise and efficient by reducing repetition and increasing reuse. By implementing DRY principles in your CSS with preprocessors, you can create variables for frequently used values such as color schemes or font sizes and reuse them throughout your code. This eliminates the need to write the same code repeatedly which saves time and improves the overall quality of your code.
In addition, preprocessors provide features like loops which allow you to generate complex CSS styles with ease. For example you can use loops to create a grid layout or generate multiple variations of a single style. This can help you reduce the amount of CSS code you need to write and make it more manageable. Overall by using DRY principles and leveraging the features of preprocessors, you can make your software development process more efficient and productive.
Complex Logic
Preprocessors gives you access to some logical syntax you would find in a normal programming language and allows you to create dynamic styling which responds to the output of the logic. This includes items such as loops, If/Else statements, and visual nesting of CSS rules.
Another advantage of preprocessors is that they include built in functions and mixins that can streamline the process of writing CSS. For example a preprocessor may provide a mixin for creating a responsive grid layout or a function for calculating values based on user input or other dynamic factors. These tools can save time and effort for developers allowing them to focus on more important aspects of their projects.
Overall, preprocessors can be a powerful tool for developers looking to create dynamic stylesheets. By providing access to logical syntax, advanced features, and built in functions, preprocessors can make it easier to write complex CSS code and achieve sophisticated styling effects. Whether you're a beginner to web development or just starting out, incorporating a preprocessor into your workflow can help you work more effectively and achieve better results.
Features of a Preprocessor:
Variables
One of the most commonly used things in any programming language is variables which CSS doesn't have. By having variables you can define a value once and reuse it throughout the entire program. Here is an example of this in SASS:
$yourcolor: #000056
.yourDiv {
color: $yourcolor;
}
By declaring the SASS yourcolor variable once, it is now possible to reuse this exact same color throughout the entire document without having to retype the definition which is effectively saving time.
Loops
Another important concept in a languages are loops which is another thing that CSS lacks. Loops are used to repeat the same instruction multiple times without having to be typed multiples of times. Here is an example with SASS:
@for $vfoo 35px to 85px {
.margin-#{vfoo} {
margin: $vfoo 10px;
}
}
Here in the example our loop saves us time from having to write the same code over again to change the margin size.
If/Else Statements
Another feature which CSS doesn't have is If/Else statements. This is something which will only make a program run when a set of instructions by a given condition are true. Here is an example in SASS:
@if width(body) > 500px {
background color: blue;
} else {
background color: white;
}
In our example the background colour will change the colour depending on the width of the page’s body.
With this article at OpenGenus, you must have the complete introductory idea of CSS Preprocessors.