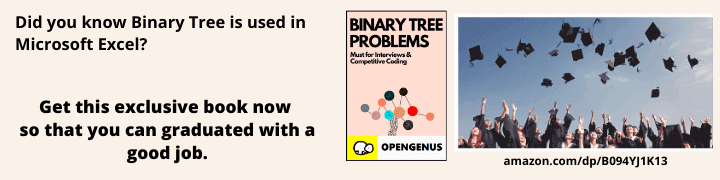
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the world of web development, a new revolutionary force is uprising and reshaping the way we build and execute web applications. Enter WebAssembly (WASM for short), a binary instruction format poised to elevate the performance and versatility of web applications. In this guide at OpenGenus, you'll learn how to use WASM, get started with it, use it for building your next application, and explore the power of WASM!
So let's just dive into this new awesome thing, i.e., WebAssembly, shall we?
What the heck is WebAssembly?
Before starting out with the basics, let's just understand what exactly WebAssembly is and whether you should even care.
So, as per the official site of WebAssembly:
WebAssembly (abbreviated Wasm) is a binary instruction format for a stack-based virtual machine. Wasm is designed as a portable compilation target for programming languages, enabling deployment on the web for client and server applications.
So what this means essentially is that it allows JavaScript to execute a piece of compiled code, opening new dimensions for high-performance web applications.
JavaScript is no longer the only language that can be run natively in browsers, and web apps will become much faster than ever before!
You might be thinking, "Will I be freed and escape from the depths of the tutorial hell and skip Javascript? "
Answer: No, JavaScipt still remains one of the most popular languages for application development, and with its big community support and the larger popularity of its runtime environment, Node.js, and the package manager NPM, there are years to come for any technology to replace it.
Basics of WebAssembly
Here's an awesome video on Wasm in 100 seconds by fireship.
Interpreted vs. Compiled:
To grasp the essence of WebAssembly, we must first differentiate between interpreted and compiled languages.
Interpreted | Compiled |
---|---|
Executed line by line | Directly Translated into machine code before runtime |
Source code is converted to bytecode and executed at runtime | Faster execution and better performance. |
Languages: Javascript, Python, PHP, Ruby etc | languages: C, C++, Rust, Go etc |
Do I need to learn another programming language?
Not really!
Because WebAssembly isn't really some other programming language, you won't actually write this code directly, but instead you can use it as a compilation target after writing programs in other languages like C, C++, Rust and compile it into WebAssembly where it can directly run on a browser, giving it a near-native feel. Sounds awesome, right?
It's an intruction format that works hand in hand with JavaScript. And the best way to start with Wasm is with AssemblyScript (a strict variant of TypeScript).
Security Considerations: WebAssembly adoption is heavily reliant on security, in addition to performance benefits.
JavaScript code is exposed to all users, which could be dangerous from a security standpoint and can be reverse engineered easily using browser developer tools.
Compilated code, on the other hand, presents additional challenges for reverse engineering, making it difficult to gain access to programmes.
So, where can I use it?
The Use of Web Assembly in Web Development: Consider Web Assembly as a dynamic tool in the toolbox of a web developer. It easily connects with online apps, enabling developers to take advantage of its performance advantages for particular modules while keeping the application's general JavaScript structure.
JavaScript Integration: Think of Web Assembly as a cooperative JavaScript collaborator. Developers are able to take advantage of each language's advantages since they function well together. High-level application logic is managed by JavaScript, and jobs requiring high performance are handled by Web Assembly.
High-performance Computing within the Web Browser: Visualise your web browser as a computational powerhouse. Complex computations that were previously limited to server-side processing can now be done directly in the browser, thanks to Web Assembly. This creates new opportunities for dynamic and interactive online apps.
Plus you can also build some awesome side projects like this one to improve your developer skills. As of now, unfortunately, WebAssembly hasn't yet seen the widespread adoption that it deserves.
Writing your first "Hello World!" Program in WebAssembly
As a developer, writing the "Hello, World!" program is a mandate, right? So let's build a simple Hello World! app using AssemblyScript and compile it to WebAssembly using Emscripten.
AssemblyScript is a language similar to TypeScript but designed for WebAssembly.
Setting Up the Environment
Installing Toolchains for Web Assembly:
Install the necessary toolchains to set up your development environment. this is just preparing your workplace with the equipment you'll need for the trip ahead.
Assembling to Web Assembly
using Emscripten: (Emscripten is a complete compiler toolchain to WebAssembly)
Picture Emscripten as an expert in converting high-level code to bytecode that is compatible with Wasm. It allows them to communicate in the common language of Web Assembly, bridging the gap between languages.
Step 1: Install the Required Tools
-
Install Node.js and npm:
Make sure you have Node.js and npm installed. You can download them from here.
-
Install and Initialize the AssemblyScript project:
Follow this to set up assemblyscript in your local repo.
npm init
npm install --save-dev assemblyscript
npx asinit .
- Install Emscripten:
Follow the instructions on the official Emscripten installation guide.
Step 2: Create the AssemblyScript Hello World Program
-
Create a new folder for your project by quickly firing up these commands on your terminal
mkdir hello-world cd hello-world
-
Edit the 'assembly/index.ts' file:
Replace its content with a simple "Hello, World!" program:export function helloWorld(): void { // Print to console // Note: This will be captured by // Emscripten's console.log // when running in a web browser. trace("Hello, World!\n"); }
Step 3: Compile AssemblyScript to WebAssembly using Emscripten
- Compile AssemblyScript to WebAssembly using Emscripten:
npx asc assembly/index.ts -b build/assembly.wasm --target wasm32
Step 4: Use Emscripten to Create JavaScript Bindings
- Generate JavaScript bindings using Emscripten:
emcc build/assembly.wasm -o build/assembly.js
Step 5: Create an HTML File to Use WebAssembly
- Create an 'index.html' file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello, WebAssembly!</title> </head> <body> <script src="build/assembly.js"></script> <script> // Call the exported function from AssemblyScript Module.helloWorld(); </script> </body> </html>
Step 6: Run Locally
Run a local server:
-
You can use a simple server like http-server or live-server. Install it via npm if you haven't:
npm install -g http-server
-
Run the server:
http-server
-
Open your browser and go to http://localhost:8080 (or the port specified by the server).
NOTE: The choice of port number is arbitrary, and you can use any available port number that is not already in use by other services on your system.
Now the console in your browser should display "Hello, World!" This example demonstrates the basic structure of an AssemblyScript program compiled to WebAssembly using Emscripten.
Key Takeaways (WebAssembly: A Beginner's Guide)
- WebAssembly Overview: WebAssembly (WASM) is a binary instruction format enhancing web app performance by allowing JavaScript to execute compiled code.
- Basics and Integration: Differentiating between interpreted and compiled languages, WebAssembly collaborates with JavaScript, offering advantages in specific modules without replacing it.
- Use Cases: WebAssembly acts as a dynamic tool in web development, connecting with online apps for performance advantages and enabling high-performance computing within browsers.
- Getting Started: The blog provides a practical guide to writing a "Hello, World!" program in WebAssembly using AssemblyScript, Emscripten, and a local server setup, demonstrating the basics and initial steps for developers.