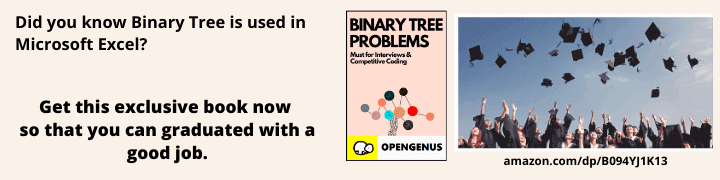
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Introduction
The landscape of programming languages is vast and diverse, each with its own set of strengths and weaknesses. Developers often face the challenge of selecting the most suitable language for their projects, considering factors like performance, ease of use, ecosystem, and platform compatibility. In this comprehensive analysis at OpenGenus, we delve into the speed aspect of programming languages, examining the results of a performance test conducted on nine popular languages. Additionally, we explore the key factors influencing language speed and provide recommendations tailored to specific use cases.
Understanding Language Speed
Compilation vs. Interpretation
One of the fundamental distinctions in language implementation is between compiled and interpreted languages. Compiled languages, such as C and C++, translate source code into machine-executable binaries before execution. This process allows for direct interaction with hardware, resulting in fast performance. In contrast, interpreted languages, like Python and JavaScript, rely on an interpreter to execute code line by line at runtime. While interpretation offers flexibility and portability, it often incurs a performance overhead due to the additional processing required during execution.
Cross-Platform Support
Languages designed for cross-platform compatibility often sacrifice raw execution speed for the sake of portability. Cross-platform languages, such as Java and C#, require an additional layer of abstraction to manage platform-specific differences. This abstraction introduces overhead in terms of runtime interpretation or dynamic code generation, impacting performance compared to languages optimized for a specific platform.
Execution Model
The execution model of a language plays a significant role in determining its performance characteristics. Low-level languages, like C and assembly, provide direct access to hardware resources, enabling efficient memory management and optimized code execution. On the other hand, high-level languages, such as Python and Ruby, abstract hardware details to simplify development but may incur performance penalties due to overhead associated with runtime interpretation and memory management.
Static vs. Dynamic Typing
Type systems in programming languages can be classified as static or dynamic, based on when variable types are determined. In statically typed languages like C and Java, variable types are resolved at compile time, allowing for early error detection and optimized code generation. In contrast, dynamically typed languages such as Python and JavaScript determine variable types at runtime, providing flexibility but potentially introducing performance overhead due to type checks and runtime type resolution.
Performance Test
To evaluate the performance of programming languages, we conducted a standardized test involving a loop iterating one billion times, calculating the sum of integers within the loop. The test serves as a representative benchmark for assessing the raw computational speed of each language.
Test Procedure
The test involved implementing a loop in each language to iterate over one billion integers and calculate their sum. The execution time of each implementation was measured using a high-precision timer to accurately capture the computational overhead of each language.
import time
start = time.time()
total_sum = 0
for i in range(1000000000):
total_sum += i
end = time.time()
print("Execution time:", end - start, "seconds")
Test Results
The following are the execution times observed for each language during the performance test:
- GO: 0.381 seconds
- Java: 0.78 seconds
- C: 0.998 seconds
- C#: 1.237 seconds
- C++: 1.453 seconds
- JavaScript: 1.178 seconds
- Rust: 8.952 seconds
- Swift: 17.37 seconds
- Python: 120.0 seconds
The results highlight significant variations in execution speed among the tested languages, underscoring the importance of language selection in achieving optimal performance for different applications.
Key Insights and Analysis
Python's Performance:
Python's relatively slow performance in the test, with an execution time of 120 seconds, can be attributed to several factors inherent to the language's design. As a high-level interpreted language, Python incurs overhead in dynamic type checking, memory management, and runtime interpretation, leading to slower execution compared to compiled languages like C and Go.
GO's Efficiency:
GO's exceptional performance, with an execution time of 0.381 seconds, can be attributed to its efficient compilation model, built-in concurrency support, and optimized standard library. As a compiled language designed for concurrent execution, GO leverages lightweight threads (goroutines) and efficient memory management to deliver high-performance applications, particularly in web servers and cloud-native environments.
Rust's Performance Trade-offs:
Rust, despite being lauded for its safety and concurrency features, exhibited relatively slower performance in the test, with an execution time of 8.952 seconds. This performance trade-off can be attributed to Rust's focus on memory safety and strict compile-time checks, which introduce overhead during execution. Additionally, the need for explicit memory management and type annotations may impact Rust's performance compared to more dynamically typed languages.
Java's Just-In-Time (JIT) Compilation:
Java's competitive performance, with an execution time of 0.78 seconds, can be attributed to its Just-In-Time (JIT) compilation model and efficient runtime environment. By compiling Java bytecode to native machine code at runtime, the JVM (Java Virtual Machine) achieves near-native performance while providing platform independence and runtime optimizations. Java's extensive standard library and robust ecosystem further contribute to its efficiency in a wide range of applications, from enterprise software to mobile app development.
Conclusion:
In conclusion, the choice of programming language significantly impacts the performance and efficiency of software applications. While some languages prioritize raw execution speed, others emphasize developer productivity, portability, or safety. By understanding the factors influencing language speed and conducting performance tests tailored to specific use cases, developers can make informed decisions when selecting the most appropriate language for their projects.
Recommendations:
Speed-Optimized Languages:
- GO: Ideal for web servers, cloud-native applications, and network programming due to its efficiency and concurrency support.
- Java: Well-suited for enterprise software, backend systems, and Android app development, leveraging JIT compilation and robust standard libraries.
- C, C++, and Rust: Recommended for system programming, operating systems, and performance-critical applications requiring low-level control, memory safety, and efficiency. Rust, in particular, offers a modern take on systems programming with a focus on safety, concurrency, and performance, making it increasingly popular for projects where memory safety and performance are paramount.
Balance of Performance and Productivity:
- Python: Despite slower execution, Python offers versatility and ease of use, making it suitable for rapid development, data analysis, and prototyping.
- JavaScript: Widely used for front-end and back-end web development, JavaScript balances performance with a vast ecosystem of frameworks and libraries.
Platform-Specific Development:
- Swift: Tailored for building high-performance applications on Apple platforms, including iOS, macOS, watchOS, and tvOS, leveraging modern language features and safety.
In summary, while speed is a critical consideration in language selection, developers should also weigh factors such as project requirements, developer productivity, ecosystem maturity, and platform compatibility to make informed decisions. By aligning language choice with project goals and constraints, developers can optimize both performance and development efficiency in their software endeavors.
Additional Considerations:
Beyond speed, other factors such as memory usage, scalability, security, and community support play vital roles in language selection. Developers should evaluate these factors holistically to ensure that the chosen language aligns with the broader objectives and constraints of their projects.
Final Thoughts:
Programming language selection is a multifaceted decision that requires careful consideration of various technical and practical factors. While speed is an essential aspect of language performance, it should be balanced with considerations such as developer productivity, project requirements, ecosystem support, and long-term maintainability. By taking a holistic approach to language selection and considering the specific needs of their projects, developers can maximize the success and effectiveness of their software endeavors.