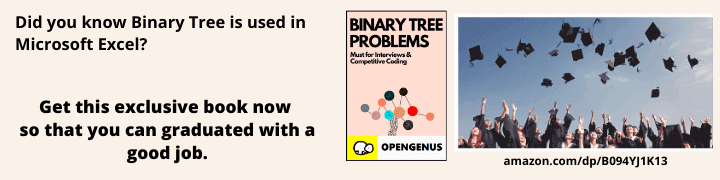
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have developed a Python Script to Like Recent Tweets with a Particular Hashtag. We have developed 2 approaches: one using tweepy library and other using requests library to make direct API calls to Twitter API.
Table of Contents
- Introduction
- Code
- Complexity
- Applications
- Questions
- Conclusion
Introduction
-
Twitter is one of the most popular social media platforms in the world. It has over 330 million active users who send over 500 million tweets per day. With so much content being generated every day, it can be difficult to keep up with everything thatâs happening.
-
One way to stay up-to-date is by using hashtags. Hashtags are a way to categorize tweets and make them easier to find.
-
In this article, we will use Python to interact with the Twitter API and like tweets with a particular hashtag.
-
To get started, we will need to create a Twitter Developer account and obtain our API credentials. We will use Tweepy, a Python library for accessing the Twitter API, to interact with Twitter.
Code
Hereâs a Python script that uses the Twitter API to like recent tweets with a particular hashtag.
An approach to make you do API calls in addition to using a Twitter API library
-
To make API calls in addition to using a Twitter API library directly, you can use a library like Requests1 which is a Python library that allows you to send HTTP requests using Python. You can use it to send GET, POST, PUT, DELETE, and other types of HTTP requests(Code given below).
-
You can also use Tweepy which is a Python library for accessing the Twitter API. It provides an easy-to-use interface for accessing Twitterâs RESTful API and Streaming API (Code given below).
-
Hereâs a code for making API calls in addition to using a Twitter API library directly:
Steps involved -->
- Import the necessary libraries
- Set up your Twitter API credentials
- Make an API call using the Twitter API library
- Make an API call using Requests
Approach 1
- Hereâs an example of how you can use Requests to make an API call:
import requests
from requests_oauthlib import OAuth1
# Replace these values with your own consumer key, consumer secret, access token and access token secret
auth = OAuth1('YOUR_CONSUMER_KEY', 'YOUR_CONSUMER_SECRET', 'YOUR_ACCESS_TOKEN', 'YOUR_ACCESS_TOKEN_SECRET')
# Define the hashtag you want to like tweets for
hashtag = "#example"
# Define the number of tweets you want to like
num_of_tweets = 10
# Search for tweets containing the hashtag
url = f'https://api.twitter.com/1.1/search/tweets.json?q={hashtag}'
response = requests.get(url, auth=auth)
tweets = response.json()
# Like the tweets
for tweet in tweets['statuses'][:num_of_tweets]:
tweet_id = tweet['id']
url = f'https://api.twitter.com/1.1/favorites/create.json?id={tweet_id}'
response = requests.post(url, auth=auth)
if response.status_code == 200:
print("Liked tweet")
else:
print(f"Error: {response.text}")
This script uses the requests and requests_oauthlib libraries to authenticate with Twitterâs API and make HTTP requests to the GET search/tweets and POST favorites/create endpoints. These direct API calls allow the script to search for tweets containing a specific hashtag and like them.
- Hereâs a line-by-line explanation of the code:
import requests
This line imports the requests library, which allows you to send HTTP requests using Python.
from requests_oauthlib import OAuth1
This line imports the OAuth1 class from the requests_oauthlib library. This class is used to handle OAuth 1 authentication with the Twitter API.
auth = OAuth1('YOUR_CONSUMER_KEY', 'YOUR_CONSUMER_SECRET', 'YOUR_ACCESS_TOKEN', 'YOUR_ACCESS_TOKEN_SECRET')
This line creates an instance of the OAuth1 class and passes in your Twitter API credentials. You will need to replace âYOUR_CONSUMER_KEYâ, âYOUR_CONSUMER_SECRETâ, âYOUR_ACCESS_TOKENâ, and âYOUR_ACCESS_TOKEN_SECRETâ with your own credentials.
hashtag = "#example"
This line defines the hashtag that you want to search for on Twitter.
num_of_tweets = 10
This line defines the number of tweets that you want to like.
url = f'https://api.twitter.com/1.1/search/tweets.json?q={hashtag}'
response = requests.get(url, auth=auth)
tweets = response.json()
These lines create a URL for the Twitter API search endpoint and include the hashtag as a query parameter. The requests.get() method is then used to send a GET request to this URL and retrieve tweets containing the specified hashtag. The response.json() method is used to parse the JSON response and store it in a variable called tweets.
for tweet in tweets['statuses'][:num_of_tweets]:
tweet_id = tweet['id']
url = f'https://api.twitter.com/1.1/favorites/create.json?id={tweet_id}'
response = requests.post(url, auth=auth)
if response.status_code == 200:
print("Liked tweet")
else:
print(f"Error: {response.text}")
These lines iterate over the first num_of_tweets tweets in the tweets['statuses'] list. For each tweet, the script extracts its ID and constructs a URL for the Twitter API favorites/create endpoint. The requests.post() method is then used to send a POST request to this URL and like the tweet. If the request is successful (i.e., if response.status_code is 200), then a message is printed to indicate that the tweet was liked. Otherwise, an error message is printed.
Approach 2
- Hereâs an example of how you can fetch recent tweets with a hashtag and retweet it using direct API calls & tweepy library:
This script uses the Tweepy library to interact with the Twitter API(Code given below).
Some theory points on the Tweepy library:
-
Tweepy is a Python library that provides an easy-to-use interface for accessing Twitterâs API.
-
With Tweepy, you can perform various tasks such as searching for tweets, accessing user information, and posting tweets.
-
To use Tweepy, youâll need to install it using pip. You can do this by running pip install tweepy in your terminal.
-
Once youâve installed Tweepy, youâll need to authenticate with Twitterâs API using your API keys and access tokens.
-
You can then create an API object using the authenticated OAuth1UserHandler object and use it to perform various tasks.
import tweepy
# Authenticate to Twitter
auth = tweepy.OAuthHandler("API key", "API secret key")
auth.set_access_token("Access token", "Access token secret")
# Create API object
api = tweepy.API(auth)
# Define the hashtag you want to like tweets for
hashtag = "#example"
# Define the number of tweets you want to like
num_of_tweets = 10
# Like the tweets
for tweet in tweepy.Cursor(api.search_tweets, hashtag).items(num_of_tweets):
try:
tweet.favorite()
print("Liked tweet")
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
This script authenticates with your Twitter account using your API key, API secret key, access token, and access token secret. It then searches for tweets with the specified hashtag and likes them. You can adjust the number of tweets you want to like by changing the num_of_tweets variable.
- Here is a line-by-line explanation of the code:
import tweepy
This line imports the Tweepy library, which we will use to interact with the Twitter API.
auth = tweepy.OAuthHandler("API key", "API secret key")
auth.set_access_token("Access token", "Access token secret")
These two lines authenticate our Twitter Developer account and obtain our API credentials. You will need to replace âAPI keyâ, âAPI secret keyâ, âAccess tokenâ, and âAccess token secretâ with your own credentials.
api = tweepy.API(auth)
This line creates an API object using Tweepy. We will use this object to interact with Twitter.
hashtag = "#example"
This line defines the hashtag we want to like tweets for. Replace â#exampleâ with your own hashtag.
num_of_tweets = 10
This line defines the number of tweets we want to like. Replace â10â with the number of tweets you want to like.
for tweet in tweepy.Cursor(api.search_tweets, hashtag).items(num_of_tweets):
This line uses a for loop to iterate through the tweets that match our hashtag. The Cursor object is used to search for tweets that match our hashtag. The items() method specifies the number of tweets we want to like.
try:
tweet.favorite()
print("Liked tweet")
except tweepy.TweepError as e:
print(e.reason)
except StopIteration:
break
We then use a try-except block to handle errors that may occur while liking tweets. If an error occurs while liking a tweet, the error message is printed. If there are no more tweets to like, the loop is broken.
Complexity
-
The code above is relatively simple and easy to understand. We first authenticate to Twitter using our API credentials.
-
We then create an API object using Tweepy.
-
We define the hashtag we want to like tweets for and the number of tweets we want to like.Finally, we use a for loop to iterate through the tweets and like them.
Applications
-
This script can be used for various applications such as social media marketing, data analysis, and more.
-
For example, if you are running a social media campaign for your business and want to increase engagement on Twitter, you can use this script to like tweets with your campaign hashtag.
Questions
- How do I authenticate with Twitter API?
- How do I search for tweets with a particular hashtag?
- How do I like tweets using Python?
Conclusion
-
In this article at OpenGenus, we learned how to write a Python script that can like recent tweets with a particular hashtag. We used Tweepy, a Python library for accessing the Twitter API, to interact with Twitter.
-
This script can be used for various applications such as social media marketing and data analysis.