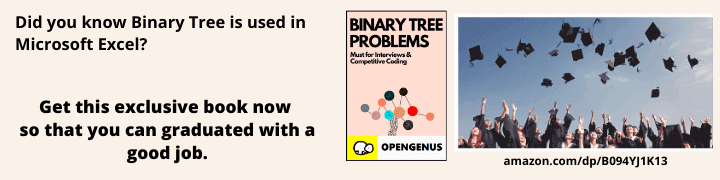
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Are you stepping into the world of Python and databases? If so, you're likely to encounter various libraries that facilitate the interaction between your Python scripts and databases. One such powerful tool is mysql-connector-python. In this guide at OpenGenus, we'll introduce you to the basics of this library, exploring its features and demonstrating its usage through a sample Python script.
Table of contents:
- What is mysql-connector-python?
- Installation
- Explanation
- A Simple Query Example
- Conclusion
What is mysql-connector-python?
mysql-connector-python is a MySQL driver for Python, enabling developers to connect Python applications to MySQL databases seamlessly. This library provides an interface for executing SQL queries, managing database connections, and handling data retrieval and manipulation.
Installation
Before diving into the world of MySQL connectivity in Python, let's ensure you have the library installed. You can install it using pip
python package installer:
$ pip install mysql-connector-python
Connecting to a MySQL Database
The heart of any database interaction lies in establishing a connection. The script snippet below demonstrates how to connect to a MySQL database using mysql-connector-python:
import mysql.connector as m
try:
# Replace the placeholders with your database credentials
connection = m.connect(host="localhost", user="root", password="your_password", database="your_database")
wire = connection.cursor()
# Your database operations go here
wire.close()
connection.close()
except Exception as e:
print(e)
Ensure to replace "your_password" and "your_database" with your actual MySQL password and database name.
Explanation:
-
import mysql.connector as m:
This line imports the mysql.connector module and aliases it as m. It provides Python with the necessary tools to connect to and interact with MySQL databases. -
try:
This begins a try-except block, a structure used for exception handling. The code inside the try block is executed, and if an exception occurs, it is caught and handled in the except block. -
connection = m.connect(host="localhost", user="root", password="your_password", database="your_database"):
This establishes a connection to a MySQL database. The connect method is called with parameters such as the host, user, password, and database to connect to a specific MySQL instance. -
wire = connection.cursor():
This line creates a cursor object (wire). A cursor is like a pointer that facilitates interaction with the database. You can execute SQL queries and retrieve results using this cursor. -
#Your database operations go here:
This is a comment indicating the placeholder where you would perform your specific database operations. You can execute SQL queries using wire.execute() and retrieve results with wire.fetchall(). -
wire.close():
This closes the cursor (wire). It's good practice to close the cursor when you're done with your database operations. -
connection.close():
This closes the database connection (connection). It's essential to close the connection to release resources and ensure a clean exit. -
except Exception as e:
If an exception occurs within the try block, it is caught, and the code inside the except block is executed. -
print(e):
This line prints the exception message. It helps in identifying and debugging any issues that might have occurred during the database connection or operations.
A Simple Query Example
Now, let's consider a straightforward example where we have a table named "employees" with columns for names and ages. We want to fetch and print the names and ages of all employees. Replace the table and column names with your actual database schema.
Sample Table: employees
ID | Name | Age |
---|---|---|
1 | Alice | 28 |
2 | Bob | 35 |
3 | Charlie | 22 |
4 | David | 29 |
5 | Eva | 32 |
import mysql.connector as m
try:
# Establishing connection to the database
connection = m.connect(host="localhost", user="root", password="your_password", database="your_database")
wire = connection.cursor()
# Executing a simple query
query = "SELECT name, age FROM employees"
wire.execute(query)
records = wire.fetchall()
# Printing the fetched data
for record in records:
print(f"Name: {record[0]}, Age: {record[1]}")
# Closing the cursor and connection
wire.close()
connection.close()
except Exception as e:
print(e)
Output
Name: Alice, Age: 28
Name: Bob, Age: 35
Name: Charlie, Age: 22
Name: David, Age: 29
Name: Eva, Age: 32
In this example, we execute a SELECT query to fetch names and ages from the "employees" table and then print the results.
Conclusion
Congratulations! You've embarked on your journey with mysql-connector-python, a valuable companion for database interactions in Python. As you explore further, delve into the library's documentation for advanced features and functionalities.
Remember, connecting Python to a MySQL database is a fundamental skill for developers. With mysql-connector-python, not only is this task achievable, but it can also be an enjoyable aspect of your coding endeavors. Happy coding!