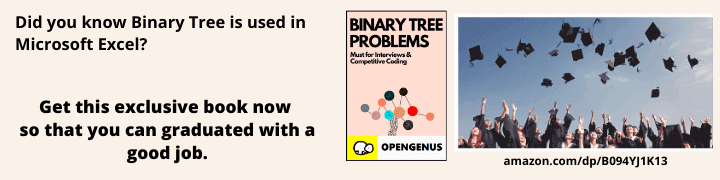
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will make a program that tracks the active windows in your laptop that you click on and prints their names on the terminal. This is program is just for education purposes.
Table of contents
- Introduction to the problem statement
- Prerequisites
- Solving the problem
- Implementing the solution
- Complexity
- Takeaways
- References
Introduction to the problem statement
Before we begin, this is the definition of an active window from easytechjunkie, “An active window is the currently selected window, which has primary focus within a computer application.”
For this problem you need to be able to know the what windows are open. The position of the mouse is the second component. Thirdly, you need to know when the mouse clicks on an active window. Fourthly, we need the findings to be printed on the terminal. The last step is to end the program.
Prerequisites
The modules you need to import are to make this program are:
- Pynput
- Pygetwindow
- time
I am using VS Code as my IDE of choice and Python 3.12.
Solving the problem
Any program can be broken down into subproblems. This are the subproblems of the click logger.
- Make a click function that detects when the mouse is used and changes the global variable that checks if a mouse is clicked. E.i.: clicked.
- A function that detects when a key is pressed. It needs to ignore all the other keys except the one that is used to end stop the program. If the special button is pressed then it should end change the global variable that is in charge of checking if the key is pressed. E.i.: running.
- Make a listener that for the mouse and the keyboard modules each.
- Print the window title every time the mouse is clicked and they end program button, e.i.: Esc button, is not pressed.
- End the program when the special key on the keyboard is pressed.
Implementing the solution
from pynput import keyboard, mouse
import pygetwindow as gw
import time
running = True
clicked = False
def on_click(x, y, button, pressed):
if pressed:
global clicked
clicked = True
if not pressed:
clicked = False
def on_press(key):
if key == keyboard.Key.esc:
global running
running = False
with keyboard.Listener(on_press=on_press) as listener, mouse.Listener(on_click=on_click) :
while (running):
for window in gw.getAllWindows():
if clicked:
if window.isActive:
print(window.title)
time.sleep(0.5) # to prevent excessive CPU usage
listener.join()
Here are some comments on the code:
- The running variable is used to control the while group. The variable clicked is used to check if a click has happened
- The on_click function has 4 parameters: the x and y coordinates, button is a value from the Button class and pressed determines whether a click has been made. For the program the parameter that matters is pressed. The function returns true or false depending on whether a click has been be done. A click is defined as a press and release of the mouse pad or a physical press and release of the mouse. Using hotkeys will not be counted clicks even do they can be used to move from one window to the other.
- The on_press function. Takes in a any key that is pressed. When the Esc button is pressed it will change end the execution of the program though the running variable
- The mouse.Listener is used to collect the events from the mousepad/touchpad and the same for the keyboard and the keyboard. Listener
- The function gw.getAllWindows() is used to get all the windows. Check in the for loop for the window that is active using window.isActive. If the listener works and the loop is still running then you print the title of the active window using window.title()
Complexity
Importing Libraries: The time complexity of importing libraries is frequently thought to be constant, therefore, it can be classified as O(1).
Setting Variables: The time complexity of setting variables is also O(1).
While Loop: The time complexity of the while loop is founded on the conditions in the while loop and the statements inside it. In this case, the loop runs until running is False. The time complexity of the loop itself can be considered as O(n) as a result of the for loop in it.
Mouse Listener: The time complexity of setting up the mouse listener using mouse.Listener is constant because it initialises the listener object. Therefore, it can be considered as O(1) – the same can be said with the keyboard listener
Worst-case vs. Best-case of Time Analysis:
The worst-case and best-case time complexity of the program is O(n) because of the number of windows open.
Space Complexity Analysis:
It is O(n) for most operations, due to the for loop.
Key Takeaways
- Using libraries to aid in the making of programs greatly simplifies their creation.
- This code can be used to test antivirus software.
- A way to stop a program while the terminal is running.