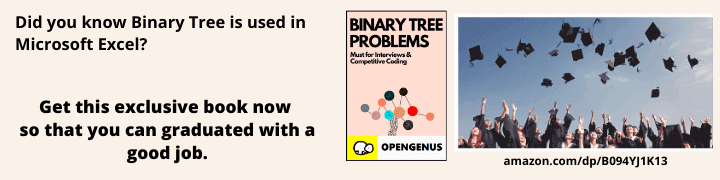
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of content
- Basics of Hangman game
- Play Strategies
- Approach to Implement
- Final Implementation
- Sample Output
- Time and Space Complexity
Basics of Hangman game
Hangman is a fun game for two or more players. In this game, one player picks a word, phrase, or sentence, and the others try to guess it by suggesting letters or numbers. But there's a catch: they can only guess a certain number of times. The traditional way to play is using pen and paper, but now you can also play it on electronics.
This classic word game has brought joy for years. Here's how it goes: The computer picks a random secret word, and it's up to you to guess it by suggesting letters. But remember, you only have a certain number of tries. Every time you guess a wrong letter, a part of the "hangman" figure is drawn on the screen. The game happens on the console, with you guessing and the computer showing the hangman as you go along.
Play Strategies
Hangman is about using what you know to guess the word correctly before running out of guesses. The secret word is shown as empty spaces on a grid. If the guesser suggests a wrong letter, a part of a stick figure is drawn. The goal is to guess the word before the stick figure is completely drawn.
Here some tricks to win the game:
- Start with Common Letters: Begin by guessing letters that are used a lot, like "E," "A," "R," and "T."
- Avoid Uncommon Letters: Don't guess letters that are rare, like "Z," unless you're sure they're in the word.
- Guess Consonants First: Try guessing consonants before vowels, as words often have more consonants.
- Look at Word Length: The length of the word gives you a hint. Short words are easier to guess than long ones.
- Find Patterns: When you know a few letters, look for patterns in words you know that fit the revealed letters.
- Use Context: If you know the word category, like "Animals," guess words related to that category.
- Keep Track: Remember the letters you've guessed to avoid repeating them.
- Educated Guesses: When you know part of the word, try guessing words that fit the pattern.
- Don't Waste Guesses: Stick to the pattern and only guess when you're fairly certain.
- Practice: Like any game, practice helps you get better at finding words and patterns.
Approach to Implement
Let's simplify the implementation of this game by breaking it down into four distinct parts, following the principle of 'Divide and Conquer':
- Step 1:
- Separate File: Make a new ".py" file, like "my_words.py".
- Create Dictionary: In that file, create a dictionary with categories as keys and lists of words as values.
- Import in Main: In your main file, write from my_words import word_dict at the top.
- Use Categories: Now you can use word_dict["category_name"] to access lists of words by their categories.
Here below, I created a separate python file to form a dictionay named Words
Words =
{'COUNTRY': ['India', 'USA', 'Italy', 'New Zealand', 'Russia', 'Germany', 'Mexico', 'China', 'Canada', 'Japan'],
'DAIRY': ['Milk', 'Butter', 'Cream', 'Cheese', 'Yoghurt', 'Ice-Cream', 'Ghee', 'Custard', 'Whipped Cream', 'Buttermilk'],
'FRUIT': ['Apple', 'Banana', 'Papaya', 'Peach', 'Pomegranate', 'Pineapple', 'Raspberries', 'Strawberries', 'Watermelon', 'Mango'],
'ANIMAL': ['Dog', 'Birds', 'Lion', 'Cat', 'Horse', 'Chicken', 'Bears', 'Monkey', 'Tiger', 'Giraffe'],
'VEGETABLE': ['Carrots', 'Potato', 'Tomato', 'Onions', 'Broccoli', 'Mushroom', 'Lettuce', 'Capsicum', 'Pumpkin', 'Zucchini'],
'PROFESSION': ['Teacher', 'Dentist', 'Physician', 'Architect', 'Lawyer', 'Software Developer', 'Artist', 'Police', 'Scientist', 'chef'],
'PLANET': ['Earth', 'Jupiter', 'Saturn', 'Venus', 'Mars', 'Mercury', 'Neptune', 'Uranus'],
'BRAND': ['Apple', 'Nike', 'Adidas', 'Google', 'Microsoft', 'Amazon', 'BMW', 'zara', 'Starbucks', 'Disney'],
'Vehicle': ['Van', 'car', 'Bus', 'Ambulance', 'Bicycle', 'Scooter', 'Motorcycle', 'Helicopter', 'Airplane', 'Boat', 'Train'],
'Feeling': ['Anger', 'Sadness', 'Anxiety', 'Fear', 'Happiness', 'Guilt', 'Disgust', 'Disappointment', 'Love'],
'Electronics': ['Computer', 'Refrigerator', 'Washing machine', 'Transistor', 'Microwave', 'Speaker', 'Printer', 'Juicer', 'Iron', 'Charger'],
'Social media': ['Instagram', 'Twitter', 'Facebook', 'Pinterest', 'LinkedIn', 'TikTok','YouTube', 'Reddit', 'Discord', 'Snapchat', 'Telegram']}
You can also make a dictionary in your main file if you prefer. However, creating a separate file for it has its benefits. It helps keep your code organized and easy to read. Plus, when you need to add new words, you can do so without any confusion.
- Step 2: Create a function that picks a word at random according to its category. Curious about how we do it? No worries – I'll guide you through the simple process of setting up this function, conveniently called "randoms".
# Define a function called Randoms that takes a dictionary 'Words' as input.
def Randoms(Words):
# Randomly select a key-value pair from the 'Words' dictionary and store it in 'res'.
res = key, val = random.choice(list(Words.items()))
# Print the chosen key as a hint for the player regarding the hidden word's category.
print(res[0])
# Extract the list of characters (val) from the selected value and store it in 'choose'.
choose = list(res[1])
# Randomly choose a character (take) from the 'choose' list.
take = random.choice(choose)
# Calculate the length of the selected character.
l = len(take)
# Create a string 's' containing an underscore followed by two spaces.
s = '_ '
# Create a string 'blank' by repeating the 's' string 'l' times.
blank = l * s
# Print the 'blank' string to represent a blank space for the chosen character.
print(blank, "\n")
# Will explain as next step about this below called guess function
guess(take)
Import random above of your code to use its function
- Step 3: Implementing the Guessing Process through guess function : Stay calm, let's break it down. In this step, we'll use a loop to repeatedly ask the player to guess a letter or word. If they guess wrong, we'll add 1 to a counter and use a function called Hangman to draw part of a stick figure. The number of incorrect guesses will determine how much of the stick figure gets drawn. This only happens when they guess a wrong word. The player has 8 chances to guess wrong. If they reach 8 wrong guesses, they lose and the loop stops. However, if they guess a correct letter, we'll remember their guess and ask for another letter. If they manage to guess all the letters correctly, the game will say "You win." If they guess the entire word correctly, they also win, and the loop stops.
Please refer to the comments in the code below for better understanding.
# Define a function named "guess" that takes one argument called "take".
def guess(take):
# Initialize a string variable "s" with a single underscore character.
s = '_'
# Create a new string "b" by repeating the underscore character as many times as the length of "take".
b = s * len(take)
# Convert the string "b" into a list of characters.
b = list(b)
# Initialize a variable "count" to keep track of incorrect guesses. Set it to 0.
count = 0
# Start a loop that continues until "count" reaches 8 (8 incorrect guesses).
while(count != 8):
# Ask the player to input their guess (a letter or the whole word) and convert it to lowercase.
choose = input("Guess letter or whole word: ").lower()
# Convert the "take" word to lowercase and store it in the variable "low".
low = take.lower()
# Convert the "low" string into a list of characters and store it in the variable "k".
k = list(low)
# Find the index of the guessed letter ("choose") within the "low" word.
i = low.find(choose)
# Check if the guess is the correct word or if the guessed letters match the original word.
if(choose == low or b == k):
# Print a winning message and break out of the loop.
print("\nYOU WIN!!!\n" + choose + " is the correct word")
break
# Check if the guessed letter was found in the word.
if(i != -1):
# Initialize a variable "j" to 0.
j = 0
# While "j" is not equal to the length of the guessed word ("choose"):
while(j != len(choose)):
# Replace the underscore in the "b" list with the guessed letter.
b[i] = choose[j]
# Move to the next character in the guessed word and the next position in the original word.
j += 1
i += 1
# Check if the entire word has been guessed correctly.
if(b == k):
# Print a winning message and break out of the loop.
print("\nYOU WIN!!!\n" + low + " is the correct word")
break
# Print the current state of the guessed word (with underscores and correctly guessed letters).
print(' '.join(map(str, b)))
else:
# Increment the "count" variable to track incorrect guesses.
count += 1
# Check if the player still has remaining attempts (less than 9).
if(count < 9):
# Call a function "Hangman" which print hangman's body in each wrong letter or word
Hangman(count, take)
Step 4: Lets make Hangman function: Every time player guess a wrong letter, a new part of the hangman is drawn. Once count value reach to 8, player will see full body part of hangman with message "you lose". It's a fun way to keep the game exciting and a bit more challenging!
def Hangman(count, take):
if(count == 1):
print('___________________\n|\n|\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 2):
print('___________________\n| |\n|\n|\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 3):
print('___________________\n| |\n| .;;;.\n| (*_*)\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 4):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 5):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\n| / |\n|\n|')
print("\nTry Again")
elif(count == 6):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n|\n|')
print("\nTry Again")
elif(count == 7):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n| /\n| /')
elif(count == 8):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n| / \ \n| / \ ')
print("\nYOU LOSE :(\n The correct word was " + take)
- Step 5: Call random function
Randoms(Words)
Final Implementation
Let's now bring together all the above mentioned steps to implement the Hangman game:
import random
from Dictionary import Words
def Hangman(count, take):
if(count == 1):
print('___________________\n|\n|\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 2):
print('___________________\n| |\n|\n|\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 3):
print('___________________\n| |\n| .;;;.\n| (*_*)\n|\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 4):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n|\n|\n|\n|')
print("\nTry Again")
elif(count == 5):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\n| / |\n|\n|')
print("\nTry Again")
elif(count == 6):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n|\n|')
print("\nTry Again")
elif(count == 7):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n| /\n| /')
elif(count == 8):
print('___________________\n| |\n| .;;;.\n| (*_*)\n| |\n| /|\ \n| / | \ \n| / \ \n| / \ ')
print("\nYOU LOSE :(\n The correct word was " + take)
def guess(take):
s = '_'
b = s * len(take);
b = list(b)
count = 0
while(count != 8):
choose = input("Guess letter or whole word: ").lower()
low = take.lower();
k = list(low)
i = low.find(choose)
if(choose == low or b == k):
print("\nYOU WIN!!!\n" + choose + " is a right word")
break
if(i != -1):
j = 0
while(j != len(choose)):
b[i] = choose[j]
j += 1
i += 1
if(b == k):
print("\nYOU WIN!!!\n" + low + " is a right word")
break
print(' '.join(map(str, b)))
else:
count += 1
if(count < 9):
Hangman(count, take)
def Randoms(Words):
res = key, val = random.choice(list(Words.items()))
print(res[0])
choose = list(res[1])
take = random.choice(choose);
l = len(take)
s = '_ '
blank = l * s;
print(blank, "\n")
guess(take)
print('\nLets Play H.A.N.G.M.A.N!!!\n')
Randoms(Words)
Words = {'COUNTRY': ['India', 'USA', 'Italy', 'New Zealand', 'Russia', 'Germany', 'Mexico', 'China', 'Canada', 'Japan'],
'DAIRY': ['Milk', 'Butter', 'Cream', 'Cheese', 'Yoghurt', 'Ice-Cream', 'Ghee', 'Custard', 'Whipped Cream', 'Buttermilk'],
'FRUIT': ['Apple', 'Banana', 'Papaya', 'Peach', 'Pomegranate', 'Pineapple', 'Raspberries', 'Strawberries', 'Watermelon', 'Mango'],
'ANIMAL': ['Dog', 'Birds', 'Lion', 'Cat', 'Horse', 'Chicken', 'Bears', 'Monkey', 'Tiger', 'Giraffe'],
'VEGETABLE': ['Carrots', 'Potato', 'Tomato', 'Onions', 'Broccoli', 'Mushroom', 'Lettuce', 'Capsicum', 'Pumpkin', 'Zucchini'],
'PROFESSION': ['Teacher', 'Dentist', 'Physician', 'Architect', 'Lawyer', 'Software Developer', 'Artist', 'Police', 'Scientist', 'chef'],
'PLANET': ['Earth', 'Jupiter', 'Saturn', 'Venus', 'Mars', 'Mercury', 'Neptune', 'Uranus'],
'BRAND': ['Apple', 'Nike', 'Adidas', 'Google', 'Microsoft', 'Amazon', 'BMW', 'zara', 'Starbucks', 'Disney'],
'Vehicle': ['Van', 'car', 'Bus', 'Ambulance', 'Bicycle', 'Scooter', 'Motorcycle', 'Helicopter', 'Airplane', 'Boat', 'Train'],
'Feeling': ['Anger', 'Sadness', 'Anxiety', 'Fear', 'Happiness', 'Guilt', 'Disgust', 'Disappointment', 'Love'],
'Electronics': ['Computer', 'Refrigerator', 'Washing machine', 'Transistor', 'Microwave', 'Speaker', 'Printer', 'Juicer', 'Iron', 'Charger'],
'Social media': ['Instagram', 'Twitter', 'Facebook', 'Pinterest', 'LinkedIn', 'TikTok','YouTube', 'Reddit', 'Discord', 'Snapchat', 'Telegram']}
Sample Output
-
Test Case 1:
-
Test Case 2:
Time and Space Complexity
Time Complexity:
- Most parts of the code have constant time complexity O(1) due to fixed operations.
- The loop inside the
guess
function contributes to a linear time complexity of O(n), where n is the length of thetake
word.
Space Complexity:
- Functions like
Hangman
,guess
, andRandoms
use a constant amount of space, resulting in O(1) space complexity. - The
Words
dictionary takes space proportional to the number of keys and values. - The
take
variable occupies space proportional to the length of the word, resulting in O(n) space complexity.
In summary, the main time complexity comes from the linear loop inside the guess
function, leading to O(n), while the space complexity remains mostly constant except for the input word and the pre-defined dictionary, which contribute to O(n) space complexity.
Take a look at my repository to better understand how Hangman is implemented. You're welcome to join and make the game even better visually. Grab the code from GitHub here: Hangman GitHub Repository.