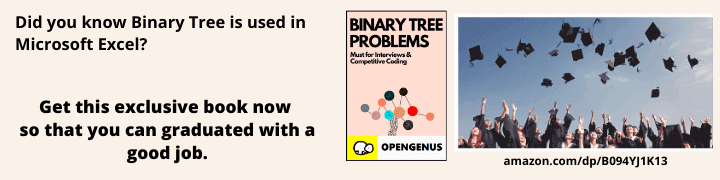
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The time module in python provides us with functionality to work with the time format, accessing current system time, changing representation format of time etc.
Some important terminologies and conventions used in this article at OpenGenus:
- epoch:It is the point where the time starts, and is platform dependent. For Unix, the epoch is January 1, 1970, 00:00:00 (UTC).
- UTC:It is Coordinated Universal Time (formerly known as Greenwich Mean Time, or GMT).
- DST:Daylight Saving Time(DST), an adjustment of the timezone by (usually) one hour during part of the year.Daylight saving time is the practice of setting the clock forward by one hour during the warmer part of the year, so that evenings have more daylight and mornings have less.
time.time():
This function is used to get the time since the epoch in seconds.It returns the time in seconds as a floating point number.
Syntax: time.time()
Parameter: None.
Return type: Returns a floating point number representing time since epoch in seconds.
time.time_ns():
This function is used to get the time since epoch in nanoseconds.It returns the time in nanoseconds as an integer number.
Syntax: time.time_ns()
Parameter: None.
Return type: Returns a integer number representing time since epoch in nanoseconds.
time.ctime():
This function takes the time in seconds as an input and converts it into a string format representation(Sun Jun 10 14:28:05 1999).If no input is provided to the function then it assumes the current time as an input and returns the string equivalent representation of the current time.
Syntax: time.ctime(secs)
Parameter: It takes a floating point number as a parameter.
Return type: It returns a string format representation(Sun Jun 10 14:28:05 1999).
Example:
import time
t=time.time()
#printing time in seconds.
print("Current time in seconds:",t)
#printing time in string format.
print("Current time in string format:",time.ctime(t))
#printing ouput of ctime() if no input is provided.
print("If no input is provided to ctime() output is:",ctime())
Output:
Current time in seconds: 1586441139.834961
Current time in string format: Thu Apr 9 19:35:39 2020
If no input is provided to ctime() output is: Thu Apr 9 19:35:39 2020
time.struct_time:
It is a type of object with a named tuple interface returned by the following functions:gmtime(), localtime(), and strptime().The values can be accessed by using the attribute name or the index.
The values that the object contains are:
Index Attribute Values
0 tm_year (for example, 1993)
1 tm_mon range [1, 12]
2 tm_mday range [1, 31]
3 tm_hour range [0, 23]
4 tm_min range [0, 59]
5 tm_sec range [0, 61]
6 tm_wday range [0, 6], Monday is 0
7 tm_yday range [1, 366]
8 tm_isdst 0, 1 or -1
N/A tm_zone abbreviation of timezone name
N/A tm_gmtoff offset east of UTC in seconds
time.gmtime():
This function takes the time in seconds a s an input and returns a struct_time in UTC. This function returns the struct_time with the dst flag set as 0.If no input is provided then the present time is assumed as the input.
Syntax: time.gmtime(secs)
Parameter: It takes a floating point number as a parameter.
Return type: Returns a struct_time object in UTC.
time.localtime():
This function is similar to gmtime() but convert the input to the local time.If no input is provided then the present time is assumed as the input.The dst flag is set to 1 when DST applies to the given time.
Syntax: time.localtime(secs).
Parameter: It takes a floating point number as a parameter.
Return type: Returns a struct_time object in local time.
time.mktime():
This function is the inverse of the localtime() function.It takes struct_time which express the local time as an input and returns the time as a floating point number.
Syntax: time.mktime(t)
Parameter: It takes a struct_time object expressed in local time as a parameter.
Return type: Returns a floating point number representing the time since epoch in seconds.
time.asctime():
This function takes a tuple or struct_time as an input and converts it into a string format representation(Sun Jun 10 14:28:05 1999).If no input is provided to the function then it assumes the current time as an input and returns the string equivalent representation of the current time.
Syntax: time.asctime(t)
Parameter: It takes a tuple or struct_tijme object as parameter.
Return type: Returns a string format representation(Sun Jun 10 14:28:05 1999).
Example:
import time
t=time.time()
st=time.gmtime(t)
print("Current time in struct_time using gmtime():\n",st)
lt=time.localtime(t)
print("\n\nCurrent time in struct_time using localtime():\n",lt)
print("\n\nCurrent time in seconds using mktime():",time.mktime(lt))
print("\n\nCurrent time in string format using asctime():",time.asctime(st))
Output:
Current time in struct_time using gmtime():
time.struct_time(tm_year=2020, tm_mon=4, tm_mday=9, tm_hour=17, tm_min=25, tm_sec=59, tm_wday=3, tm_yday=100, tm_isdst=0)
Current time in struct_time using localtime():
time.struct_time(tm_year=2020, tm_mon=4, tm_mday=9, tm_hour=22, tm_min=55, tm_sec=59, tm_wday=3, tm_yday=100, tm_isdst=0)
Current time in seconds using mktime(): 1586453159.0
Current time in string format using asctime(): Thu Apr 9 17:25:59 2020
time.strftime():
This function takes a tuple or struct_time representing a time as returned by gmtime() or localtime() to a string as specified by the format argument.If time argument in not provided to the function then it assumes the local time as the input for time argument.
There are many directives which can be used in the format string argument.The directives are given below:
%a:Locale’s abbreviated weekday name.
%A:Locale’s full weekday name.
%b:Locale’s abbreviated month name.
%B:Locale’s full month name.
%c:Locale’s appropriate date and time representation.
%d:Day of the month as a decimal number [01,31].
%H:Hour (24-hour clock) as a decimal number [00,23].
%I:Hour (12-hour clock) as a decimal number [01,12].
%j:Day of the year as a decimal number [001,366].
%m:Month as a decimal number [01,12].
%M:Minute as a decimal number [00,59].
%p:Locale’s equivalent of either AM or PM.
%S:Second as a decimal number [00,61].
%U:Week number of the year (Sunday as the first day of the week) as a decimal number [00,53]. All days in a new year preceding the first Sunday are considered to be in week 0.
%w:Weekday as a decimal number [0(Sunday),6].
%W:Week number of the year (Monday as the first day of the week) as a decimal number [00,53]. All days in a new year preceding the first Monday are considered to be in week 0.
%x:Locale’s appropriate date representation.
%X:Locale’s appropriate time representation.
%y:Year without century as a decimal number [00,99].
%Y:Year with century as a decimal number.
%z:Time zone offset indicating a positive or negative time difference from UTC/GMT of the form +HHMM or -HHMM, where H represents decimal hour digits and M represents decimal minute digits [-23:59, +23:59].
%Z:Time zone name (no characters if no time zone exists).
%%:A literal '%' character.
Syntax: time.strftime(format,t)
Parameter:
- format: A string of combination of the derivatives shown above.
- t: A tuple or struct_tijme object.
Return type: A string as specified by the format parameter.
time.strptime():
This function is the inverse of the strftime() function,it takes time in string format and a string of directives for format as input and return the struct_time as an output.It uses the same directives as the strftime() function. The default directive string is "%a %b %d %H:%M:%S %Y".
Syntax: time.strptime(string,format)
Parameter:
- string: A string representing time as per the format parameter.
- format: A string of combination of the derivatives shown above.
Return type: A struct_tijme object.
Example:
import time
lt=time.localtime()
print("Local time as struct_time tuple:\n",lt)
strt=time.strftime('%a %b %d %H:%M:%S %Y',lt)
print("\nConverting struct_time to string format:",strt)
tupt=time.strptime(strt)
print("\nConverting time in string format into tuple(struct_time) format:",tupt)
Output:
Local time as struct_time tuple:
time.struct_time(tm_year=2020, tm_mon=4, tm_mday=10, tm_hour=11, tm_min=12, tm_sec=26, tm_wday=4, tm_yday=101, tm_isdst=0)
Converting struct_time to string format: Fri Apr 10 11:12:26 2020
Converting time in string format into tuple(struct_time) format: time.struct_time(tm_year=2020, tm_mon=4, tm_mday=10, tm_hour=11, tm_min=12, tm_sec=26, tm_wday=4, tm_yday=101, tm_isdst=-1)