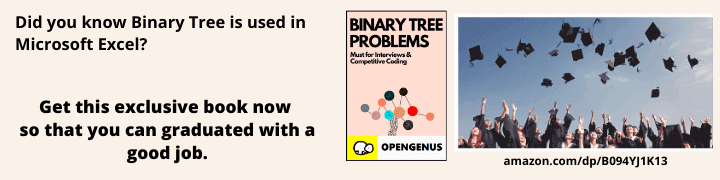
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of contents:
- Introduction
- Understanding Linear Search
- OOP Concepts
- Implementing Linear Search using OOP
- Example Usage
- Conclusion
Introduction
Linear search is a basic searching algorithm that sequentially examines each element in a list to find the target value. While not the most efficient search algorithm for large datasets, it serves as a fundamental concept in computer science and is essential to understand. In this article at OpenGenus, we will explore how to implement the linear search algorithm in Python using Object-Oriented Programming (OOP) concepts. By leveraging the power of OOP, we can create a reusable and modular linear search class that can be easily integrated into different projects.
Understanding Linear Search
Linear search, also known as sequential search, works by traversing a list of elements one by one until the desired target is found or the end of the list is reached. It has a time complexity of O(n), where 'n' is the number of elements in the list. This means the search time grows linearly with the size of the list.
OOP Concepts
-
Class and Object:
In Python, a class is a blueprint for creating objects. Objects are instances of a class that encapsulate data and methods. We will use a class to define the linear search functionality, and objects of this class will perform the actual searching on different lists. -
Constructor (
__init__
):
The constructor is a special method that initializes the object when it is created. We'll use it to initialize the list to be searched and the target element. -
Method:
Methods are functions defined inside a class and are used to perform actions on the object's data. We'll create a method to implement the linear search algorithm.
Implementing Linear Search using OOP
Let's start by creating our LinearSearch class with the necessary methods:
class LinearSearch:
def __init__(self, target_list, target_element):
self.target_list = target_list
self.target_element = target_element
self.found_index = -1
def search(self):
for index, element in enumerate(self.target_list):
if element == self.target_element:
self.found_index = index
break
Explanation:
- We define the
LinearSearch
class with a constructor (__init__
), which takes two arguments:target_list
(the list to be searched) andtarget_element
(the element we want to find in the list). - The constructor initializes three instance variables:
target_list
,target_element
, andfound_index
.found_index
will store the index of the target element if found, or remain -1 if not found. - We create a
search
method that performs the linear search. It uses afor
loop to iterate over the elements intarget_list
using theenumerate()
function, which gives both the index and element at that index. - Inside the loop, we compare each element with the
target_element
. If a match is found, we update thefound_index
with the current index and break out of the loop.
Example Usage
Now, let's use our LinearSearch
class to search for an element in a list:
# Sample list for demonstration
my_list = [12, 45, 78, 34, 56, 90, 23]
# Create an instance of LinearSearch
search_instance = LinearSearch(my_list, 56)
# Perform the search
search_instance.search()
# Check if the element is found and display the result
if search_instance.found_index != -1:
print(f"Element found at index: {search_instance.found_index}")
else:
print("Element not found in the list.")
Explanation:
- We create a sample list
my_list
with some elements. - Next, we create an instance of
LinearSearch
calledsearch_instance
and passmy_list
and the target element56
to the constructor. - We call the
search()
method onsearch_instance
to perform the linear search. - Finally, we check the value of
found_index
. If it is not equal to -1, it means the element is found, and we display its index. Otherwise, we print a message indicating that the element is not present in the list.
Conclusion
In this article at OpenGenus, we have explored how to implement the linear search algorithm in Python using Object-Oriented Programming (OOP) concepts. By creating a LinearSearch
class, we encapsulated the search functionality into a reusable and modular unit. Linear search, though not the most efficient, is an important concept to understand, as it forms the basis for more complex searching algorithms.
Remember that while OOP is a powerful paradigm for organizing code and promoting reusability, for large datasets or frequently performed searches, more efficient search algorithms like binary search or hash-based methods should be considered.