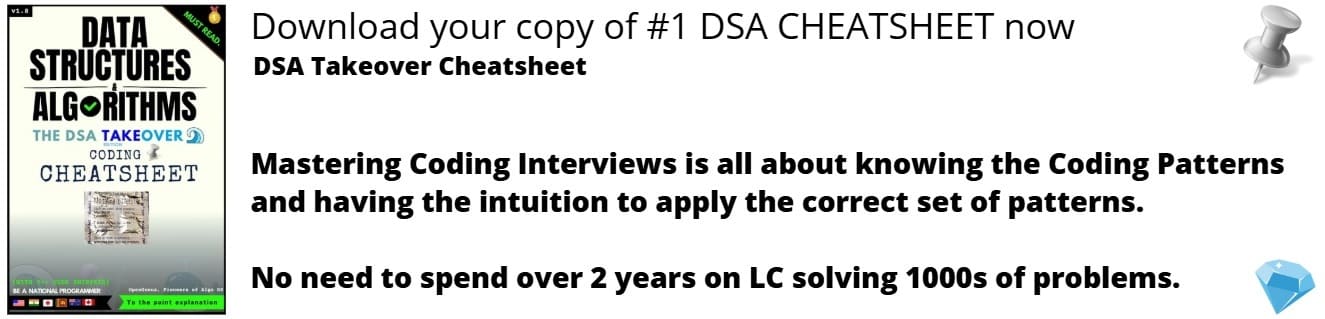
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Getting Started
- Key Takeaways
- Introduction
- Definition of Friend Classes
- Syntax and Examples
- Use cases
- Inheritance and Friendship
- Encapsulation
Key Takeaways
Key Takeaways (Friend Classes in C++)
- Friend classes enable access to private and protected members, promoting controlled communication between classes.
- Usage scenarios include maintaining privacy while allowing functionality sharing and facilitating unit testing.
- Friendship, distinct from inheritance, enhances encapsulation by enabling inter-class communication.
Introduction
In this article at OpenGenus, we will be going over friend classes in C++, what they're used for, usage examples, difference between friendship and inheritance, and alternatives.
Definition of Friend Classes
Friend is a keyword in C++. Normally we wouldn't be able to access private or protected members of classes within other classes. However we can use Friend keyword just for that. For class B to access class A's private and protected members, we would define class B as friend in the class A. See the examples below.
Syntax and Examples
#include <iostream>
#include <string>
class A {
private:
std::string privateValue;
protected:
std::string protectedValue;
public:
std::string publicValue;
A()
{
privateValue = "Private Area";
protectedValue = "Protected Area";
publicValue = "Public Area";
}
friend class B;
};
class B {
public:
void display(A& obj)
{
std::cout
<< obj.privateValue << std::endl
<< obj.protectedValue << std::endl
<< obj.publicValue << std::endl;
}
};
int main(void)
{
A foo;
B bar;
bar.display(foo);
return 0;
}
Use Cases
Friend classes can be used in many different ways. The main reason we use friend classes is when you have a class that has members that shouldn't be public, but another class would use its functionalities. Therefore we can provide the privacy for that class while still being able to use its members.
Another reason is to unit test. You can use friend classes to write and test classes' private or protected members.
Although we will not be talking much about friend functions, you can use friend keyword to overload operators like << and >>. These are used to determine the class objects behaviour when streamed (Input and Output).
Inheritance and Friendship
Inheritance and friendship are different in C++. With inheritance, the child class inherits all functionalities of the base class and makes its own. For example consider the following example.
#include <iostream>
class Base
{
public:
Base() {}
};
class Derived : public Base
{
public:
Derived() {}
};
void bar(Base* ptr)
{
std::cout << "The address of the pointer is " << &ptr << std::endl;
}
int main(void)
{
Derived* foo = new Derived();
bar(foo);
delete foo;
return 0;
}
In this example you can see that we are able to pass Derived class pointer as a Base class pointer to the function "bar".
Friendship only uses the said members of the base class instead of making them its own.
Encapsulation
Since friend keyword allows you to access normally inaccessible members of a class, most think this violates encapsulation. However friendship in fact enhances the encapsulation if used properly. For example you could have two classes inter-communicate with each other while preserving the privacy of their private and protected members for other classes.
#include <iostream>
#include <string>
class A {
private:
std::string privateValueA;
public:
A()
{
privateValueA = "Private Value for A";
}
friend class B;
};
class B {
private:
std::string privateValueB;
public:
B ()
{
privateValueB = "Private Value for B";
}
void displayB(A& obj)
{
std::cout << obj.privateValueA << std::endl; // Works
}
friend class A;
};
class Foo{
public:
Foo(A& obj1, B& obj2)
{
// Will get errors depending on the compiler
// while trying to compile the lines below
// as they are declared as private for their
// respective classes.
std::cout << obj1.privateValueA << std::endl;
std::cout << obj2.privateValueB << std::endl;
}
};
int main(void)
{
A aObject;
B bObject;
Foo bar(aObject, bObject);
return 0;
}
With this, you must have the complete idea of Friend Class in C++.