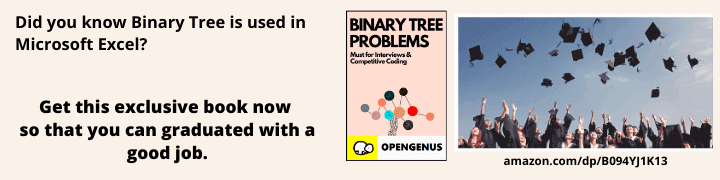
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus.org, we will discuss emplace method of Queue container in C++ STL.
Questions regarding emplace that will be answered in this article will be:
- What does the emplace function in the Queue class do?
- What are the benefits of using the emplace function?
- What is the time complexity of the emplace function?
- Are there situtations when using emplace has no benefit?
- What are the practical applications of the emplace function?
1. What does the emplace function in the Queue class do?
Queue::emplace works similarly to queue::push. When you use the emplace function, you will add an element to the queue's end. This is an example of how to use emplace:
#include <iostream>
#include <queue>
int main() {
std::queue<int> myQueue;
// Using emplace to add elements to the queue
myQueue.emplace(1);
myQueue.emplace(2);
myQueue.emplace(3);
// Displaying the elements in the queue
while (!myQueue.empty()) {
std::cout << myQueue.front() << " ";
myQueue.pop();
}
return 0;
}
2. What are the benefits of using the emplace function?
The emplace function constructs an element in place without the need for a temporary object. This is not the same as queue::push since the latter requires the creation and copying/moving of an object into the queue. This requires more time than emplace, as it might require unnecessary copies or copies/moves. In some cases, this makes emplace more efficient than push.
In certain cases, using emplace could improve readability of the code.
Here is an implementation of emplace with a queue of objects:
#include <iostream>
#include <queue>
// Simple class with a constructor
class Person {
public:
// Constructor with two parameters
Person(const std::string& n, int a) : name(n), age(a) {}
// Member function to display information
void display() const {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
private:
std::string name;
int age;
};
int main() {
std::queue<Person> peopleQueue;
// Using emplace to construct objects in place
peopleQueue.emplace("Alice", 25);
peopleQueue.emplace("Bob", 30);
peopleQueue.emplace("Charlie", 22);
// Displaying information
while (!peopleQueue.empty()) {
Person person = peopleQueue.front();
person.display();
peopleQueue.pop();
}
return 0;
}
If you wanted to use push instead emplace, this is how the code would have looked like:
#include <iostream>
#include <queue>
// Simple class with a constructor
class Person {
public:
// Constructor with two parameters
Person(const std::string& n, int a) : name(n), age(a) {}
// Member function to display information
void display() const {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
private:
std::string name;
int age;
};
int main() {
std::queue<Person> peopleQueue;
// Using push to add objects to the queue
//peopleQueue.push("Alice", 25); ERROR: No matching member function for call to 'push'
peopleQueue.push(Person("Alice", 25));
peopleQueue.push(Person("Bob", 30));
peopleQueue.push(Person("Charlie", 22));
// Displaying information
while (!peopleQueue.empty()) {
Person person = peopleQueue.front();
person.display();
peopleQueue.pop();
}
return 0;
}
As you can see, the implementation that uses push requires an object to be created and moved into queue. The emplace implementation, on the other hand, created the objects in place, eliminating the need to move them into a queue. This is a situation where emplace is slightly more efficient than push. This efficiency can be greater in objects of larger size.
3. What is the time complexity of the emplace function?
The time complexity of the emplace function is O(1). This is because it takes a constant amount of time to add something to end of the queue as it is a memory location that is defined by pointers.
4. Are there situtations when using emplace has no benefit?
Queue::emplace shines when a queue requires large objects to be created and added to said queue. However, there is no benefit in using emplace instead of push when it comes to primitive data types with one parameter.
Example:
// Using emplace for a simple type
myQueue.emplace(42);
// Equivalent using push
myQueue.push(42);
Furthermore, emplace has no benefit over push when it comes to adding existing objects. Emplace is much more useful when it comes to creating new objects.
Example:
// Adding an existing object to the queue
MyObject obj;
myQueue.emplace(obj); // Not as clear as using push or insert
5. What are the practical applications of the emplace function?
The emplace function is useful in situations where you need to efficiently construct and insert elements into a queue. Real-world applications include real-time systems and large data containers.
Key-takeaways
Emplace is a function that has advantages and disadvantages when compared to the standard push function in the queue class.
Pros:
- Emplace can be more efficient than push when there is a need to create new objects.
- Emplace can avoid the creation of temporary objects which is required for the push function.
- Emplace can improve readibility in certain situations
Cons:
- When the queue contains primitive types or an object construction is not required, emplace has no advantage over push. As a result, using emplace instead of push may be confusing to the reader.
- Emplace can be difficult to implement if the object requires a complex initialization. In this case, it may be preferable to create the object separately and then add it to the queue.
- This could also make the code less readable.
Ultimately, the distinction between queue::emplace and queue::push is small. While there are times when using emplace can result in significant performance gains, most of the time, the more readable option is the better choice.
With this article at OpenGenus.org, you must have a strong idea of Queue::emplace in C++ STL.