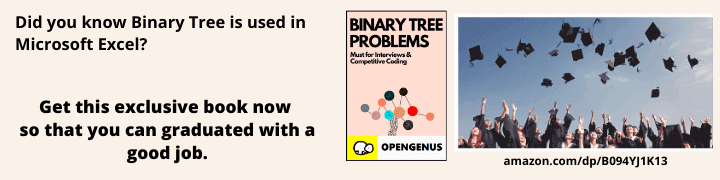
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In the broad world of software development, our primary goal is to create applications that work well, are easy to maintain, and can grow as needed. One important principle that helps us achieve this is called Command Query Separation (CQS). In this article at OpenGenus, we will explore CQS, its practical benefits, and how it can help us build software systems that are not only effective but also strong and adaptable.
Table of contents:
- Unpacking Command Query Separation (CQS)
- Commands: Making Things Happen
- Queries: Getting Information
- Avoiding Ambiguity: Not Mixing Commands and Queries
- Following CQS: Keeping Things Separate
- Enhancing Clarity, Maintenance, and More
- In Summary: How CQS Helps in Software Design
- Question
Unpacking Command Query Separation (CQS)
At its core, Command Query Separation (CQS) is a design concept that emphasizes categorizing methods or functions into two clear groups: actions that do something (commands) and those that provide information (queries).
The main idea behind CQS is to separate actions that change the system's state from those that only gather information without making any changes.
Commands: Making Things Happen
Commands are actions that can modify the system's state. These actions make changes, updates, or transformations in data, resources, or other parts of an application. To understand this better, think of a social media app where a user posts something new. This act of posting is a command because it changes the state of the system by adding a new post to the user's profile.
class SocialMediaService {
createPost(user, content) {
// Logic to create a new post
// ...
}
}
In this JavaScript example, the createPost
method is a command because it alters the system's state by adding a new post.
Queries: Getting Information
On the other hand, queries are actions that only retrieve information from the system, without making any changes. Imagine an online shopping app where customer check if a specific product is available. This action, which gathers information without affecting the system's state, is query.
class ProductService {
getProductAvailability(productID) {
// Logic to check product availability
// ...
}
}
In this JavaScript representation, the getProductAvailability
method is a query because its purpose is to retrieve information without making any changes.
Avoiding Ambiguity: Not Mixing Commands and Queries
Sometimes, things can get accomplished when commands and queries are combined in a single action, which goes against the CQS principle.
Consider a financial app where users withdraw more money and at the same time request their updated account balance.
class AccountService {
processWithdrawal(user, amount) {
// Logic to update account balance
// Logic to return the updated balance
// ...
}
}
The processWithdrawal
method blends both command(updating the account balance) and query(returning the balance) actions, which doesn't follow CQS.
Following CQS: Keeping Things Separate
To stick to CQS, it's important to clearly separate command and query actions. Let's improve the previous example by splitting these actions.
class AccountService {
withdrawFunds(user, amount) {
// Logic to update account balance
// ...
}
getAccountBalance(user) {
// Logic to retrieve account balance
// ...
}
}
Here, the withdrawFunds
method handles the command of updating the account balance, while the getAccountBalance
method deals with the query of retrieving the balance.
This separation ensures that we follow CQS and keeps our code clear.
Enhancing Clarity, Maintenance, and More
By embracing Command Query Separation(CQS), developers can enjoy several benefits:
- Clarity and Easier Maintenance: Separating commands from queries leads to code that is easy to understand and maintain. This separation reduces confusion and helps identify and resolve issues quickly.
- Reduced Side Effects: CQS reduces the chance of unintended problems that can happen when mixing data changes with data retreival. This separation encourages good coding practices and prevent bugs.
- Effective Testing: Since commands often involve changing things, testing them can be complex. By separating commands and queries, developers can focus their testing on expected state changes. Queries, which don't change anything, are usually easier to test.
- Opportunities for Optimization: Queries, which don't alter the system's state, can be optimized more flexibly. This allows for targeted performance improvements without worrying about unintended consequences on data.
- Scalability Made Easier: CQS supports scalability by letting developers concentrate on the specific needs of commands and queries individually. This separation enables targeted optimization efforts, resulting in responsive and efficient applications.
In Summary: How CQS Helps in Software Design
Think of Command Query Separation (CQS) as a helpful tool in software design. It makes software easier to understand and work with. By separating actions that change things from those that gather information, developers can build software that's reliable and can adapt to changes easily.
When you embrace CQS in your software development journey, you're setting yourself up for success in the ever-changing world of software design.
Question
What is the primary purpose of Command Query Separation (CQS) in software design?- Commands, which are responsible for changing the system's state.
- Queries, which are responsible for retrieving information from the system without causing any changes.