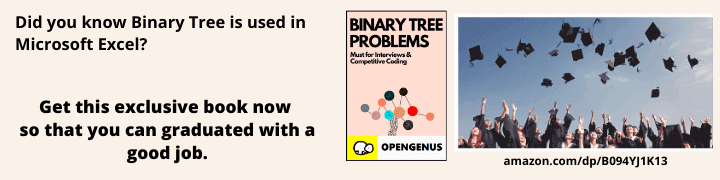
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of Contents
- Introduction
- Basic SQL Queries
- Advanced SQL Queries
- Aggregation Functions
- Modifying Data with SQL
- Working with Dates and Time
- Performance Optimization
- Combining SQL with Programming Languages
- Best Practices for Writing SQL Queries
- Conclusion
Introduction
In this article at OpenGenus, we have covered a brief overview of SQL and its importance in database management along with explanation of SQL queries and their role in retrieving data from databases.
Basic SQL Queries
SELECT statement: Retrieve data from a single table.
SELECT column1, column2, ...
FROM table_name;
Explanation: This query selects specific columns (column1, column2, etc.) from the table named "table_name" and retrieves all rows from that table.
WHERE clause:
Filter data based on conditions.
SELECT column1, column2, ...
FROM table_name
WHERE condition;
Explanation: The WHERE clause is used to filter rows based on specific conditions. Only the rows that satisfy the given condition will be included in the query result.
ORDER BY clause:
Sort query results.
SELECT column1, column2, ...
FROM table_name
ORDER BY column1 ASC/DESC;
Explanation: The ORDER BY clause is used to sort the query result based on the values in "column1." ASC stands for ascending (default), and DESC stands for descending order.
LIMIT clause:
Control the number of returned rows.
SELECT column1, column2, ...
FROM table_name
LIMIT number_of_rows;
Explanation: The LIMIT clause is used to restrict the number of rows returned in the query result to the specified "number_of_rows."
Advanced SQL Queries
JOINs:
Combine data from multiple tables.
SELECT column1, column2, ...
FROM table1
JOIN table2 ON table1.column = table2.column;
Explanation: JOINs are used to combine data from different tables based on a common column. This query uses an INNER JOIN, which returns only the matching rows from both tables.
GROUP BY clause:
Group data for aggregation.
SELECT column1, COUNT(column2) AS count_column2
FROM table_name
GROUP BY column1;
Explanation: The GROUP BY clause groups rows based on the values in "column1." The COUNT function is used to calculate the number of occurrences of "column2" for each group.
HAVING clause:
Filter grouped data.
SELECT column1, COUNT(column2) AS count_column2
FROM table_name
GROUP BY column1
HAVING COUNT(column2) > 10;
Explanation: The HAVING clause filters the groups generated by the GROUP BY clause. In this query, it includes only the groups where the count of "column2" is greater than 10.
Subqueries:
Use queries within queries.
SELECT column1, column2, ...
FROM table_name
WHERE column1 IN (SELECT column1 FROM table2 WHERE condition);
Explanation: This query uses a subquery inside the WHERE clause to filter rows in "table_name" where the value of "column1" matches any of the values returned by the subquery.
Aggregation Functions
COUNT, SUM, AVG, MIN, MAX: Understanding and using these functions.
SELECT COUNT(*), SUM(column1), AVG(column2), MIN(column3), MAX(column4)
FROM table_name;
Explanation: The SELECT statement uses various aggregation functions to retrieve information about the data in "table_name." COUNT(*) counts the total number of rows, SUM calculates the sum of "column1," AVG calculates the average of "column2," MIN finds the minimum value of "column3," and MAX identifies the maximum value of "column4."
Modifying Data with SQL
INSERT: Add new records to a table.
INSERT INTO table_name (column1, column2, ...)
VALUES (value1, value2, ...);
Explanation: The INSERT statement adds a new row to "table_name" with the specified values for "column1," "column2," and so on.
UPDATE:
Modify existing records.
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
Explanation: The UPDATE statement modifies existing rows in "table_name" by setting the values of "column1," "column2," and so on to the specified values, based on the given condition.
DELETE:
Remove records from a table.
DELETE FROM table_name
WHERE condition;
Explanation: The DELETE statement removes rows from "table_name" that satisfy the given condition.
Working with Dates and Time
Date functions: Manipulate date and time values.
SELECT column1, DATE_ADD(date_column, INTERVAL 1 MONTH) AS next_month
FROM table_name;
Explanation: The DATE_ADD function is used to add an interval (in this case, 1 month) to the date values in "date_column." The result is displayed as "next_month" in the query result.
Extracting information from date/time fields.
Copy code
SELECT column1, YEAR(date_column) AS year, MONTH(date_column) AS month, DAY(date_column) AS day
FROM table_name;
Explanation: The YEAR, MONTH, and DAY functions are used to extract the year, month, and day components from the date values in "date_column." The results are displayed as separate columns in the query result.
Performance Optimization
Indexes: Improving query performance with indexing.
CREATE INDEX index_name ON table_name (column1, column2, ...);
Explanation: The CREATE INDEX statement creates an index on one or more columns in "table_name," improving the performance of queries that involve those columns.
Avoiding costly queries and improving efficiency.
Explanation: In this section, we discuss general best practices to avoid inefficient queries, such as avoiding using "SELECT *," using appropriate data types, and optimizing complex queries.
Combining SQL with Programming Languages
Incorporating SQL queries into applications (e.g., PHP, Python).
import sqlite3
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
query = "SELECT column1, column2 FROM table_name WHERE condition;"
cursor.execute(query)
result = cursor.fetchall()
conn.close()
Explanation: This Python code demonstrates how to connect to a SQLite database, execute an SQL query, and retrieve the result using the sqlite3 module.
Parameterized queries to prevent SQL injection.
import sqlite3
conn = sqlite3.connect('database.db')
cursor = conn.cursor()
query = "SELECT column1, column2 FROM table_name WHERE column3 = ?;"
value = ('some_value',)
cursor.execute(query, value)
result = cursor.fetchall()
conn.close()
Explanation: This Python code shows how to use parameterized queries with the sqlite3 module to prevent SQL injection attacks. The value to be inserted in place of the "?" is passed as a separate parameter to the execute() method.
Best Practices for Writing SQL Queries
Proper formatting and indentation.
Explanation: In this section, we emphasize the importance of clean and organized SQL code, which enhances readability and maintainability.
Naming conventions for tables and columns.
Explanation: We discuss the significance of consistent naming conventions to make SQL code more understandable and standardized.
Avoiding common mistakes.
Explanation: Common mistakes in SQL queries, such as missing WHERE clauses in UPDATE and DELETE statements, are discussed, along with how to avoid them.
Conclusion
Importance of SQL in data management and its applications in various domains.
Explanation:
SQL queries are fundamental to database management, enabling us to interact with data efficiently and effectively. In this guide, we covered the basics of SQL queries, including retrieval, filtering, and sorting of data. We explored advanced concepts like JOINs, grouping, and subqueries, which empower us to handle complex data scenarios. Additionally, we delved into aggregation functions, data modification, and working with dates and time. To ensure optimal performance and security, we discussed performance optimization techniques and the integration of SQL with programming languages. By adhering to best practices, developers can write maintainable and secure SQL queries to harness the full potential of their databases.