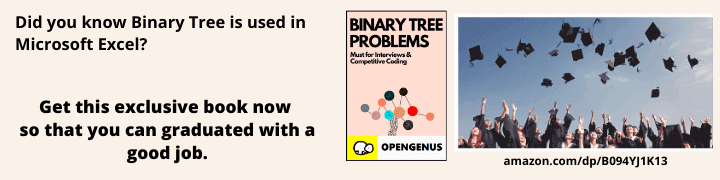
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the concept of Compound Interest and implemented a Java program to compute Compound Interest.
Table of contents:
- Calculating Compound Interest in Java
- Implementation in Java
Calculating Compound Interest in Java
Compound interest is a financial concept that describes the process of earning interest on the principal amount as well as the accumulated interest. It is a powerful tool that can help individuals and businesses grow their wealth over time. In this article, we will explore how to calculate compound interest in Java.
Formula for Calculating Compound Interest
The formula for calculating compound interest is:
A = P (1 + r/n)^(nt)
Where:
- A = the final amount
- P = the principal amount
- r = the annual interest rate (as a decimal)
- n = the number of times the interest is compounded per year
- t = the time (in years)
Implementation in Java
To implement this formula in Java, we can create a function that takes in the principal amount, annual interest rate, number of times compounded per year, and time in years as inputs. The function can then return the final amount after compounding the interest.
Hereβs an example implementation of a compound interest calculator in Java:
public class CompoundInterestCalculator {
public static double calculate(double principal, double rate, int compoundingsPerYear, double timeInYears) {
double amount = principal * Math.pow(1 + (rate / compoundingsPerYear), compoundingsPerYear * timeInYears);
return amount;
}
public static void main(String args) {
double principal = 1000;
double rate = 0.05;
int compoundingsPerYear = 12;
double timeInYears = 5;
double finalAmount = calculate(principal, rate, compoundingsPerYear, timeInYears);
System.out.println("Principal: $" + principal);
System.out.println("Annual Interest Rate: " + rate);
System.out.println("Compounding Period: " + compoundingsPerYear + " times per year");
System.out.println("Time: " + timeInYears + " years");
System.out.println("Final Amount: $" + finalAmount);
}
}
In the above code, we define a calculate function that takes in the principal amount, annual interest rate, number of times compounded per year, and time in years as inputs. It then returns the final amount after compounding the interest using the formula we discussed earlier.
In the main function, we define the values for the inputs and call the calculate function. We then print out the results to the console.
When we run this program, we get the following output:
Principal: $1000.0
Annual Interest Rate: 0.05
Compounding Period: 12 times per year
Time: 5.0 years
Final Amount: $1283.3595809000004
As we can see from the output, the final amount after 5 years of compounding interest is $1283.36.
Differences between Compound and Simple Interest
Simple interest is a type of interest that is calculated only on the principal amount. In other words, it does not take into account the accumulated interest. On the other hand, compound interest takes into account the accumulated interest as well as the principal amount, resulting in a higher overall return.
Calculation
Simple interest is calculated as: I = P * r * t, where I is the interest, P is the principal, r is the interest rate, and t is the time.
Compound interest is calculated as: A = P (1 + r/n)^(nt), where A is the final amount, P is the principal, r is the interest rate, n is the number of n is the number of times the interest is compounded per year, and t is the time.
With this article at OpenGenus, you must have the complete idea of Compound Interest and how to compute it in Java.