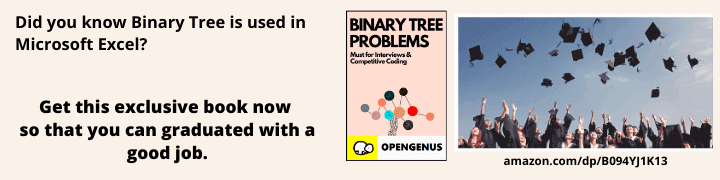
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Car rental systems have become increasingly popular over the years, providing a convenient and cost-effective mode of transportation for individuals and businesses alike. In this article, we will take a look at the key features a car rental system needs to offer, its high-level, low-level design, database design, and some of the already existing car rental software.
Table of contents:
- Introduction
- Key Features and System Requirements
- Functional Requirements
- Non-Functional Requirements
- System Scale and Requirements
- Database Design in Car Rental System
- Leading Car Rental Systems
Key Features and System Requirements
Car rental systems have gained significant popularity over the years, offering a convenient and cost-effective mode of transportation for individuals and businesses alike. A car rental system is an essential tool that helps users rent a car, manage their bookings, and make payments securely. In this article, we will discuss the key features that a car rental system needs to offer, its high-level and low-level design, database design, and some of the existing car rental software.
The key requirements that need to be offered by the Car Rental System can be classified into functional and non-functional requirements.
Functional Requirements
- Allow users to search for available cars by location, date, and time, and view car details such as make, model, year, and features.
- . Users can book a car, select pick-up and drop-off locations, and make payments using a secure payment gateway.
- The system should send a confirmation email or SMS to the user with the booking details and rental agreement.
- Allow users to cancel or modify their bookings, and refund the appropriate amount based on the cancellation policy.
- The system should notify the user and the rental agency about any changes to the booking, such as delays, cancellations, or extensions.
- Provide an admin dashboard for the rental agency to add and manage cars, update rental rates, and view sales reports.
- The system should ensure data privacy and security, and protect against fraud and unauthorized access.
Non-Functional Requirements
- The system should be highly available and scalable to handle peak loads during weekends and holidays.
- The system should be user-friendly, responsive, and accessible on different devices and platforms.
- The system should have a fast and reliable search and booking engine, and provide real-time updates on car availability and pricing.
- The system should have a robust and reliable payment gateway, and ensure secure and seamless transactions.
- The system should comply with industry standards and regulations, and protect the user's personal and financial data.
In this diagram, there are two primary actors: User and Admin. The User can search for available cars, view car details, book cars, modify bookings, and cancel bookings. The Admin can manage cars and rental rates.
The use cases are represented as ovals, and the actors are represented as stick figures. The arrows show the flow of information between the actors and use cases.
System Scale and Requirements
The scale of the car rental system depends on the number and distribution of its users. For instance, a local car rental system would have a limited number of users, and the system requirements would be relatively straightforward. However, a national car rental system would have a large number of users, and the system requirements would be complex. In such a case, the system should be able to handle a large number of concurrent users, ensure high availability, and have the necessary infrastructure to support the system's requirements.
Based on the system scale, the system requirements would vary. A local car rental system would have simple software requirements, while a national car rental system would have more complex software requirements. Some of the software requirements for a car rental system include:
- A server running Windows Server/Linux OS
- A multi-threading capable backend language like Python, Java or Node.js
- Front-end frameworks like React/Vue for the client
- Relational DBMS like MySQL, PostgreSQL, etc
- Containers and orchestration services like Kubernetes (for a large setting like a national car rental company).
- The database design of the car rental system should be designed to accommodate the storage and retrieval of relevant data. The design should also ensure that the data is consistent, accurate, and secure. The database should store information about users, cars, rental rates, and bookings.
In conclusion, a car rental system is an essential tool for individuals and businesses that need reliable and cost-effective transportation. The system should offer a range of key features, including real-time updates, secure payment processing, and user-friendly interfaces. With the right software requirements and database design, a car rental system can provide a seamless and hassle-free experience for both users and rental agencies.
//Person class as a base class for User and Admin classes
class Person {
constructor(firstName, lastName, email, phoneNo, address) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.phoneNo = phoneNo;
this.address = address;
}
}
//User class, which extends the Person class
class User extends Person {
constructor(firstName, lastName, email, phoneNo, address, password) {
// Call the parent class constructor with super()
super(firstName, lastName, email, phoneNo, address);
// Initialize the password and rentals array properties
this.password = password;
this.rentals = [];
}
//Method to search for available vehicles based on location and rental dates
searchVehicles(location, startDate, endDate) {
// search vehicles logic here
}
//Method to rent a vehicle and add a new rental to the rentals array
rentVehicle(vehicle, startDate, endDate) {
// rent vehicle logic here
const rental = new Rental(this, vehicle, startDate, endDate, totalCost);
this.rentals.push(rental);
return rental;
}
//Method to view the user's rental history
viewRentals() {
// view rentals logic here
}
//Method to modify an existing rental by rental ID
modifyRental(rentalId, newStartDate, newEndDate) {
// modify rental logic here
const rental = this.rentals.find((r) => r.id === rentalId);
if (rental) {
rental.modifyRental(newStartDate, newEndDate);
}
}
//Method to cancel an existing rental by rental ID
cancelRental(rentalId) {
// cancel rental logic here
const rentalIndex = this.rentals.findIndex((r) => r.id === rentalId);
if (rentalIndex !== -1) {
this.rentals.splice(rentalIndex, 1);
}
}
//Method to make a payment for a rental or other expense
makePayment(paymentInfo) {
// make payment logic here
}
//Method to submit the return date of a rental by rental ID
submitVehicleReturnDate(rentalId, returnDate) {
const rental = this.rentals.find((r) => r.id === rentalId);
if (rental) {
rental.submitVehicleReturnDate(returnDate);
}
}
}
//Admin class, which also extends the Person class
class Admin extends Person {
constructor(firstName, lastName, email, phoneNo, address, password) {
// Call the parent class constructor with super()
super(firstName, lastName, email, phoneNo, address);
// Initialize the password property
this.password = password;
}
//Method to manage vehicles (e.g. add, delete, update)
manageVehicles() {
// manage vehicles logic here
}
//Method to manage rentals (e.g. approve, deny, modify)
manageRentals() {
// manage rentals logic here
}
//Method to view all rentals (for all users)
viewRentals() {
// view rentals logic here
}
//Method to cancel an existing rental by rental ID
cancelRental(rentalId) {
// cancel rental logic here
}
}
class Vehicle {
constructor(make, model, year, type, capacity, pricePerDay, location, color, brand) {
this.make = make;
this.model = model;
this.year = year;
this.type = type;
this.capacity = capacity;
this.pricePerDay = pricePerDay;
this.location = location;
this.color = colorl
this.brand = brand;
}
}
class Rental {
constructor(user, vehicle, startDate, endDate, totalCost) {
this.user = user;
this.vehicle = vehicle;
this.startDate = startDate;
this.endDate = endDate;
this.totalCost = totalCost;
this.status = "Booked";
}
modifyRental(newStartDate, newEndDate) {
// modify rental logic here
}
cancelRental() {
// cancel rental logic here
}
submitVehicleReturnDate(returnDate) {
this.returnDate = returnDate;
if (this.returnDate > this.endDate) {
// raise alert if the car is returned late
console.log("Late return alert!");
}
}
}
class Payment {
constructor(cardholderName, cardNumber, expiryDate, cvv) {
this.cardholderName = cardholderName;
this.cardNumber = cardNumber;
this.expiryDate = expiryDate;
this.cvv = cvv;
}
makePayment(amount) {
// make payment logic here
}
}
Database Design in Car Rental System
The above database desgin has five tables: User, Rental, Admin, Payment, and Vehicle. These tables are used to manage a rental car company's database.
The User table has columns for id, first_name, last_name, gender, address, and phone_no, all of which are not nullable. The id column is set as the primary key for this table. The Rental table has columns for id, user_id, vehicle_id, start_date, end_date, total_cost, and payment_id, all of which are not nullable. The id column is set as the primary key for this table. The user_id, payment_id, and vehicle_id columns have foreign key constraints that reference the User, Payment, and Vehicle tables, respectively.
The Admin table has columns for id, first_name, last_name, email, and address, all of which are not nullable. The id column is set as the primary key for this table. The Payment table has columns for id, cardholder_name, card_number, expiry_date, and cvv, all of which are not nullable. The id column is set as the primary key for this table.
The Vehicle table has columns for id, model, year, type, capacity, price_per_day, location, color, and brand, all of which are not nullable. The id column is set as the primary key for this table. The Rental table also has a foreign key constraint that references the Vehicle table.
Overall, these tables will store data related to users, rentals, payments, vehicles, and administrators. By creating these tables, the rental car company can more efficiently manage their data and access information about their customers, vehicles, rentals, and payments.
Leading Car Rental Systems
There are several leading rental companies that offer services worldwide. Some of the top car rental companies are:
- Enterprise Rent-A-Car - It is one of the largest car rental companies in the world, with over 7,600 locations in more than 85 countries. It offers a wide range of vehicles, including luxury cars, SUVs, and trucks.
- Hertz Rent A Car - It is another major car rental company with over 10,000 locations in more than 150 countries. It offers a variety of vehicles, including electric cars, luxury cars, and SUVs.
- Avis Car Rental - Avis is a popular car rental company with more than 5,500 locations in over 165 countries. It offers a range of vehicles, including compact cars, SUVs, and luxury vehicles.
- Budget Rent A Car - Budget is a global car rental company with more than 3,000 locations in over 120 countries. It offers a range of vehicles, including economy cars, SUVs, and luxury vehicles.
These leading car rental companies provide users with a hassle-free car rental experience with a variety of features and options to choose from. They offer various types of vehicles to suit different needs, and some of them also offer additional services such as GPS, roadside assistance, and insurance options. Users can easily book a car online or through a mobile app, and these companies also provide customer support to assist users with any queries or issues.