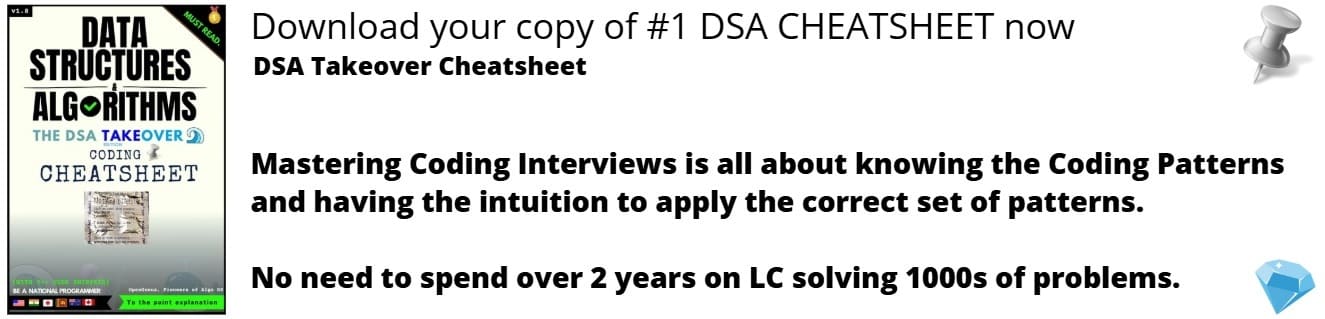
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
JavaScript, the ubiquitous programming language for web development, has traditionally been single-threaded, meaning it can only execute one task at a time. However, with the introduction of web workers and recent advancements in browser technology, developers can now leverage multithreading capabilities to execute tasks in parallel, significantly enhancing the performance of their JavaScript applications.
Summary:
- Introduction
- Understanding Multithreading
- Benefits of Multithreading in JavaScript
- Web Workers: Enabling Multithreading
- Example: Multithreading in JavaScript using Web Workers
- Conclusion
Introduction:
Multithreading is the ability to execute multiple threads concurrently within a single process. In the context of JavaScript, it allows executing multiple tasks simultaneously, effectively utilizing available system resources and improving the responsiveness and performance of web applications. By breaking down complex tasks into smaller units and executing them concurrently, multithreading enables faster execution times and a smoother user experience.
The benefits of multithreading in JavaScript are numerous. Firstly, it enhances performance by allowing computationally intensive tasks to run in the background without blocking the main thread. This ensures that the user interface remains responsive, even during heavy computations or data processing. Additionally, multithreading enables parallel processing, leveraging the power of modern multicore processors to perform calculations, data manipulation, and other operations faster. By distributing work across multiple threads, developers can fully utilize the available hardware resources and achieve optimal performance.
Overall, multithreading in JavaScript is a game-changer for web developers, providing them with the tools to optimize their applications and deliver exceptional performance. In the following sections, we will delve deeper into the concept of multithreading, explore the benefits it brings to JavaScript development, and provide examples of how to implement multithreading using web workers. So let's dive in and unlock the full potential of parallelism in JavaScript!
Understanding Multithreading
Multithreading is the ability to execute multiple threads concurrently within a single process. In the context of JavaScript, it allows executing multiple tasks simultaneously, effectively utilizing available system resources and improving the responsiveness and performance of web applications.
Benefits of Multithreading in JavaScript:
- Enhanced Performance: By utilizing multiple threads, computationally intensive tasks can be executed in the background without blocking the main thread, resulting in a more responsive user interface.
- Parallel Processing: Multithreading enables simultaneous execution of independent tasks, leveraging the power of modern multicore processors to perform calculations, data processing, and other operations faster.
- Improved User Experience: Multithreading ensures that time-consuming operations, such as data fetching or complex calculations, do not cause the UI to freeze, leading to a smoother and more enjoyable user experience.
Web Workers: Enabling Multithreading:
Web Workers are a built-in feature of modern browsers that allow executing JavaScript code in background threads, separate from the main thread. They enable true multithreading in JavaScript by providing a mechanism to offload computationally intensive tasks to separate worker threads.
Example: Multithreading in JavaScript using Web Workers:
// main.js
const worker = new Worker('worker.js'); // Create a new web worker by providing the URL of the worker script, 'worker.js'.
worker.onmessage = function(event) { // Set up an event listener to capture messages sent by the worker.
console.log('Result:', event.data); // Log the result received from the worker to the console.
};
worker.postMessage(42); // Send the value 42 to the worker using the postMessage() method.
// worker.js
self.onmessage = function(event) { // Set up an event listener to receive messages from the main script.
const result = event.data * 2; // Perform a computation by multiplying the received data by 2.
self.postMessage(result); // Send the computed result back to the main script using the postMessage() method.
};
Lets see Multithreading more in the following example:
// main.js
const worker1 = new Worker('worker1.js');
const worker2 = new Worker('worker2.js');
// When worker1 finishes its task, log the result
worker1.onmessage = function(event) {
console.log('Result from worker 1:', event.data);
};
// When worker2 finishes its task, log the result
worker2.onmessage = function(event) {
console.log('Result from worker 2:', event.data);
};
// Send data to worker1
worker1.postMessage(10);
// Send data to worker2
worker2.postMessage(20);
// worker1.js
self.onmessage = function(event) {
const data = event.data;
const result = data * 2;
self.postMessage(result);
};
// worker2.js
self.onmessage = function(event) {
const data = event.data;
const result = data + 5;
self.postMessage(result);
};
In this example, we have two web workers, worker1 and worker2, each performing a different task. Here's a breakdown of the code:
- We create two instances of Worker, worker1 and worker2, by providing the URLs of their respective worker scripts, worker1.js and worker2.js.
- We set up onmessage event listeners for both workers to capture the messages they send back.
- We send data to worker1 using the postMessage() method. In this case, we send the value 10.
- We send data to worker2 using the postMessage() method. Here, we send the value
- In worker1.js, we define the onmessage event listener to handle messages received from the main script. We extract the data sent from the main script using event.data.
- We perform a simple computation by doubling the data received, resulting in result.
- Finally, we send the result back to the main script using self.postMessage().
- Similarly, in worker2.js, we define the onmessage event listener, extract the data, add 5 to it, and send the result back to the main script.
When the workers finish their tasks, they post messages back to the main script. The event listeners defined in the main script capture these messages and log the results to the console.
This example showcases how multiple web workers can work independently, processing data and returning results to the main script asynchronously. By splitting the workload across different threads, we maximize the usage of available system resources and achieve better performance in JavaScript applications.
In the example above, the main script creates a new web worker by providing the URL of the worker script. It then sets up an onmessage event listener to receive messages from the worker. The main script sends a message to the worker using the postMessage() method, and the worker responds by posting a message back with the result. The main script logs the result to the console.
Example: Finding the Number of Prime Numbers Less Than N Using Web Workers
How to efficiently find the number of prime numbers less than a given value N using web workers. Web workers allow us to perform heavy computations in the background without blocking the main thread, resulting in a more responsive user experience.
Step 1: Creating the Web Worker File
The first step is to create a JavaScript file called primeWorker.js, which will contain the code for our web worker. This file will be responsible for calculating prime numbers in a separate thread.
// primeWorker.js
// Function to check if a number is prime
function isPrime(num) {
if (num <= 1) {
return false;
}
for (let i = 2; i * i <= num; i++) {
if (num % i === 0) {
return false;
}
}
return true;
}
// Listener for receiving messages from the main thread
self.onmessage = function (event) {
const { N } = event.data;
let count = 0;
// Count the number of primes less than N
for (let i = 2; i < N; i++) {
if (isPrime(i)) {
count++;
}
}
// Send the result back to the main thread
self.postMessage(count);
};
Step 2: Main JavaScript File
In your main JavaScript file, let's call it main.js, you can create a web worker, send a message to it with the desired value of N, and handle the result when it's received.
// main.js
// Function to handle the web worker's response
function handleWorkerResult(event) {
const count = event.data;
console.log(`Number of primes less than N: ${count}`);
// Further processing or displaying the result
}
// Create a new web worker from the primeWorker.js file
const worker = new Worker('primeWorker.js');
// Handle messages received from the web worker
worker.onmessage = handleWorkerResult;
// Start the calculation by sending a message to the web worker
const N = 100; // Replace with your desired value of N
worker.postMessage({ N });
Step 3: Implementing the Solution
Include the main.js file in an HTML file, and when you open this HTML file in a web browser, it will initiate the web worker and calculate the number of prime numbers less than N. The result will be logged in the browser's console.
In conclusion By leveraging the power of web workers, we can perform computationally intensive tasks, such as finding prime numbers, without impacting the performance of the main thread. This approach allows for a smoother user experience in web applications that require complex calculations. Remember to consider browser compatibility and fallback options if web workers are not supported in your target environment.
Web workers are a valuable tool in a developer's arsenal for creating responsive and efficient web applications. I hope this tutorial has helped you understand how to utilize web workers to find the number of prime numbers less than a given value N.
Questions
- What is multithreading in JavaScript, and how does it differ from single-threaded execution?
- What are the benefits of implementing multithreading in JavaScript applications?
- How can web workers enable multithreading in JavaScript? Provide an overview of their usage.
- What is the purpose of the onmessage event listener in the web worker script?
- In the provided example code, what computations are performed by worker1.js and worker2.js respectively?
- How can multithreading improve the performance of computationally intensive tasks in JavaScript applications?
- What precautions should developers take when utilizing multithreading in JavaScript to avoid potential issues?
- Describe a real-world scenario where multithreading in JavaScript can significantly enhance application performance.
Conclusion:
Multithreading in JavaScript through the use of web workers opens up exciting possibilities for developers to optimize their applications, improve performance, and deliver a smoother user experience. By leveraging parallelism, we can harness the full potential of modern hardware and unlock new opportunities for building high-performance web applications. Start exploring multithreading in your JavaScript projects and take your applications to the next level!
Remember to use multithreading judiciously, as excessive parallelism can introduce complexities and potential synchronization issues. Always profile and benchmark your code to ensure you're achieving the desired performance improvements.
Happy multithreading in JavaScript!