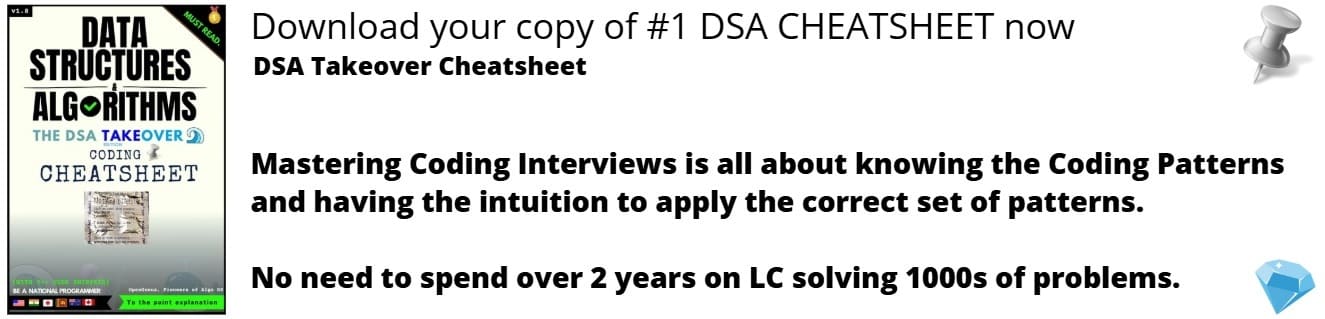
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore some common functions of Scanner Class in Java. It will introduce the Scanner Class and some common methods that developer may need to call when they apply the Scanner Class and some points that the developer needs to be careful about.
Since this article may not cover every methods of Scanner Class, if this class seems interesting and useful, developers can explore more methods in this class here
-
Table of Contents:
- What is Scanner Class in Java
- Import Scanner Class
- Create Instance in Scanner Class
- Read different types of data
- Handle user's input
- Read file input line by line
- Close the Scanner
- Conclusion
-
What is Scanner Class in Java
The Scanner class allows the developer to read input from different input streams, including the console and files. It also provides some methods to parse and extract different types of input which include Integers, Strings, and more.
-
Import Scanner Class
To use this class, the developer needs to import it into their Java program. The example is provided below:
import java.util.Scanner;
-
Create an instance of the Scanner class
After importing the Scanner Class, the developer can create an instance of it through invoking its constructor. The examples are provided below:
- Read input from the console:
Scanner sc = new Scanner(System.in);
- Read input from a file:
Scanner sc = new Scanner(new File ("file1.txt"));
- Read input from a String:
String str = "Hello World";
Scanner sc = new Scanner (str);
-
Read different types of data
The Scanner Class offers different methods to read various types of data from the input. The followings are some commonly used examples. More methods can be looked up in the Standard Java Library.
- Read integers:
int num = sc.nextInt();
- Read floating-point numbers:
double val = sc.nextDouble();
- Read Strings:
String str = sc.nextLine();
-
Handle User Input
When the developer tries to read the user input through Scanner class, it is important to pay attention to the potential excpetions that may occur. The developer may catch the IOException. The example is provided below:
I/O Exception
This exception occurs when using the Scanner Class, especially when read input from a file. The reasons for the exception may be: files to be used as sources may not exist, the file may not be readable by the user program, etc. Developers can use the try-catch block to catch the exception. Before showing the example, let's briefly talk about Java try-catch. Statements in the try block may throw an exception. The catch block will state the exception which it deals with, and it will be executed if and only if the code in the try block throws the exception. If the I/O expection occrs, with the try-catch block, it will report about the error message in the catch block and exit the program. System.err is often used to print the error messages.
try{
Scanner sc = new Scanner(new File ("file1.txt"));
} catch (IOException e){
System.err.println("File not found");
}
-
Read File Input Line by Line
Another useful way of applying the Scanner Class is to read a file line by line. But the demonstration of how to read the file input line by line with code sample, this article will first discuss a set of boolean methods start with "hasNext" in the Scanner Class. Some commonly used methods will be introduced here
- hasNext():
This method will return true if the scanner still have another token in its input. The following is one way of using it.Scanner sc = new Scanner(new File ("file1.txt")); boolean result = sc.hasNext();
- hasNextLine():
This method will return true if there is another line in the input. The following is an example of using it. This method will also be used later in the demonstration of reading file line by line.Scanner sc = new Scanner(new File ("file1.txt")); boolean result = sc.hasNextLine();
- hasNextInt():
This method will return true if the next token in the input stream can be interpreted as an int value using the nextInt() method.Scanner sc = new Scanner(new File ("file1.txt")); boolean result = sc.hasNextInt();
- hasNextDouble():
This method will return true if the next token in the input stream can be interpreted as an double value using nextDouble() method.Scanner sc = new Scanner(new File ("file1.txt")); boolean result = sc.hasNextDouble();
With the knowledge of the set of "hasNext" methods in the Scanner class and the IOException, the developer can read a input file line by line like the following code sample:
try{
Scanner sc = new Scanner(new File ("file1.txt"));
while (sc.hasNextLine()){
System.out.println(sc.nextLine());
}
sc.close();
} catch (IOException e) {
System.err.println ("Error occur");
}
It is also worth mentioning that there is not just one way of reading the file line by line. Some developers also use BufferedReader Class to achieve this function. The main difference for these two classes would be that Scanner Class parses the input data while BufferedReader Class just reads the characters. However, this article will not go furthur in explaining the BufferedReader Class.
-
Close the Scanner
After finished reading the input, it is essential to remember to close the Scanner to release any associated system resources. After close the Scanner, the developer can prevent any resource leaks and ensure optimal memory management. The following is the example of close the Scanner.
sc.close();
-
Conclusion
The Scanner Class in Java simplifies the process of reading and parsing user input. It also offers a lot of methods to handle different types of data and input sources. It can be helpful for developing more interative and user friendly Java application. The basic steps of using the Scanner Class in Java would be first import the Scanner Class. Second, create an instance to invoke the constructor. Then, the developer may call different methods in the Scanner Class for different purposes.