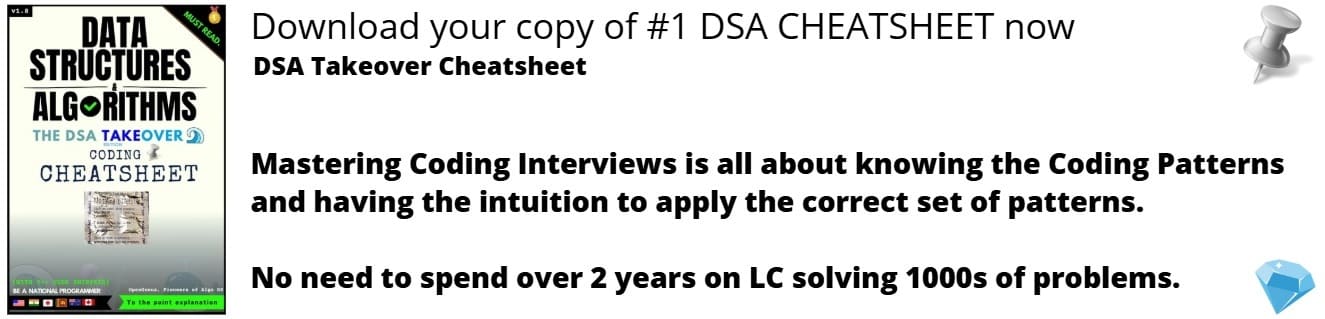
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article at OpenGenus, we will explore the notion of Concatenation operation in CNN in detail.
- Table of Contents:
- Introduction
- Mathematical model
- code for concatenation operation in CNN
- Advantages and disadvantages
- Applications
- Application of concatenation operation in various model architectures
- Conclusion
Introduction
Convolutional Neural Networks (CNNs) are a class of deep learning models that have achieved great success in various computer vision tasks such as image classification, object detection, and semantic segmentation. One of the key components of CNNs is the convolutional layer, which applies a set of filters to the input image to extract features. However, in many cases, the features extracted by a single filter may not be sufficient to accurately classify an image. In such cases, concatenation operation in CNN can be used to combine features from multiple filters to improve the classification accuracy.
The concatenation operation can be used in various ways in CNNs. One common approach is to concatenate feature maps from multiple convolutional layers to create a more expressive feature representation. For example, in the popular ResNet architecture, the residual blocks use concatenation to combine the output of a convolutional layer with the input to the block. This allows the network to learn more complex representations by preserving the lower-level features.
Another way to use concatenation is in multi-scale feature extraction. In this approach, multiple convolutional layers with different kernel sizes are applied to the input image to extract features at different scales. The feature maps from each layer are then concatenated to create a more comprehensive feature representation that captures information at multiple scales. This approach is commonly used in object detection and semantic segmentation tasks.
Convolutional neural networks (CNNs) are a powerful type of deep learning model that has revolutionized many fields, including computer vision, natural language processing, and speech recognition. CNNs are characterized by their ability to learn hierarchical representations of input data, which allows them to achieve state-of-the-art performance on a wide range of tasks.
In this article, we will explore the concatenation operation in detail, including its mathematical formulation, advantages, disadvantages, and applications in deep learning. We will also provide sample code and programs to help readers better understand this important operation.
Mathematical Model
The concatenation operation in a convolutional neural network (CNN) can be represented mathematically as follows:
Let's assume that we have two feature maps with dimensions H x W x C1 and H x W x C2 respectively, where H and W are the height and width of the feature maps, and C1 and C2 are the number of channels in the feature maps.
To concatenate these two feature maps, we first need to ensure that they have the same height and width. This can be achieved by using a 1x1 convolutional layer with stride 1 on the feature map with a smaller number of channels, to increase its number of channels to match the larger feature map. Let's denote the resulting feature map as F1 with dimensions H x W x C2.
We can then concatenate the two feature maps along the channel dimension to obtain a new feature map F2 with dimensions H x W x (C1 + C2). Mathematically, this can be represented as:
F1 = 1x1_Conv(F1, C2)
F2 = Concatenate(F1, F2)
where 1x1_Conv() is the 1x1 convolutional layer and Concatenate() is the concatenation operation.
Note that this operation can be repeated for multiple feature maps, by concatenating them along the channel dimension to obtain a final feature map with dimensions H x W x C, where C is the total number of channels of all the concatenated feature maps.
Code for Concatenation operation in CNN
Here's an example program that demonstrates the use of concatenation operation in a simple CNN model using Keras library in Python:
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Concatenate
# Define the input shape of the images
input_shape = (256, 256, 3)
# Create a sequential model
model = Sequential()
# Add a convolutional layer with 32 filters and kernel size of 3x3
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=input_shape))
# Add a max pooling layer with pool size of 2x2
model.add(MaxPooling2D(pool_size=(2, 2)))
# Add another convolutional layer with 64 filters and kernel size of 3x3
model.add(Conv2D(64, (3, 3), activation='relu'))
# Add another max pooling layer with pool size of 2x2
model.add(MaxPooling2D(pool_size=(2, 2)))
# Flatten the output of the last convolutional layer
model.add(Flatten())
# Define two fully connected layers with 128 units each
fc1 = Dense(128, activation='relu')
fc2 = Dense(128, activation='relu')
# Define two input tensors for the fully connected layers
input1 = model.output
input2 = model.output
# Concatenate the output of the last convolutional layer twice to create two input tensors
concat = Concatenate()([input1, input2])
# Connect the concatenated input tensors to the fully connected layers
fc1_output = fc1(concat)
fc2_output = fc2(fc1_output)
# Add the fully connected layers to the model
model.add(fc1)
model.add(fc2)
# Add a final output layer with one unit for binary classification
model.add(Dense(1, activation='sigmoid'))
# Compile the model with binary crossentropy loss and Adam optimizer
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Print the summary of the model
model.summary()
In this example, we define a simple CNN model with two convolutional layers and two fully connected layers. We use the 'Concatenate()' layer in Keras to concatenate the output of the last convolutional layer twice to create two input tensors for the fully connected layers. We then connect the concatenated input tensors to the fully connected layers and add them to the model. Finally, we compile the model with binary crossentropy loss and Adam optimizer and print the summary of the model.
Advantages and Disadvantages
- Advantages:
-
Enables information flow from one layer to another: Concatenation operation allows features learned by one layer to be directly passed on to another layer, which can improve the overall performance of the model by enabling the flow of useful information between different layers.
-
Increases the expressive power of the model: By concatenating feature maps from multiple layers, the model can learn more complex features than would be possible by using a single layer alone, leading to higher accuracy and better generalization.
-
Provides flexibility in designing network architectures: The ability to concatenate feature maps from multiple layers gives designers more flexibility in building deep learning architectures that can perform more complex tasks.
- Disadvantages:
-
Increases model complexity and computational cost: Concatenation operation increases the number of parameters in the model, which can lead to increased computational cost and longer training times.
-
May introduce noise into the model: Concatenating feature maps from multiple layers may also introduce noise into the model, which can reduce the accuracy of the model and make it harder to train.
-
May lead to overfitting: When concatenation is used excessively, it can lead to overfitting, where the model performs well on the training data but poorly on new data. To avoid overfitting, proper regularization techniques must be employed.
Applications of Concatenation Operation in CNN
Concatenation operation is a powerful tool in CNNs that is used in a wide range of applications. Here are some of the applications of concatenation operation in CNN:
-
Object detection: In object detection tasks, concatenation operation is often used to combine features from different layers of a CNN to improve object recognition accuracy.
-
Semantic segmentation: In semantic segmentation tasks, concatenation operation is used to combine feature maps from different layers of a CNN to enable more accurate segmentation of different regions in an image.
-
Image synthesis: In image synthesis tasks, concatenation operation is used to combine features from different layers of a CNN to generate new images that are similar to the training data.
-
Natural Language Processing: In Natural Language Processing (NLP) tasks, concatenation operation can be used to combine the output from different layers of a CNN that process different types of input, such as text and images.
-
Feature extraction: In general, concatenation operation is used to extract features from different layers of a CNN, which can then be used for a wide range of applications, such as image classification, object recognition, and more.
Application of concatenation operation in various model architectures
There are several model architectures in which concatenation operation is commonly used in CNNs. Here are a few examples:
-
U-Net: U-Net is a popular architecture used for image segmentation tasks. It consists of an encoder path that gradually reduces the spatial resolution of the input image and an accompanying decoder path that upsamples the feature maps to obtain a segmentation map. In U-Net, concatenation operation is employed to fuse feature maps from the encoder path with corresponding feature maps in the decoder path. This enables the model to combine high-level and low-level features, resulting in improved segmentation accuracy.
-
ResNet (Residual Network): ResNet is a widely used architecture known for its effectiveness in training very deep networks. ResNet introduces the concept of residual blocks, which use skip connections to pass the output of one layer directly to a deeper layer. Concatenation is often used in these skip connections to concatenate the feature maps from the previous layer with the output of the deeper layer. By concatenating the feature maps, ResNet allows the model to learn residual mappings, facilitating the training of deeper networks.
-
DenseNet: DenseNet is another architecture that encourages feature reuse and strengthens information flow across layers. In DenseNet, each layer is connected to every other layer in a feed-forward manner, resulting in a dense connectivity pattern. Concatenation operation is used to concatenate the feature maps from all preceding layers, creating a dense feature map that is passed on to subsequent layers. This dense connectivity facilitates the gradient flow and encourages feature reuse, leading to improved accuracy and parameter efficiency.
-
InceptionNet: InceptionNet, also known as GoogLeNet, is designed to capture multi-scale features by using parallel convolutional pathways with different kernel sizes. In these pathways, concatenation operation is used to concatenate feature maps from different kernel sizes before passing them on to subsequent layers. This allows the model to capture information at different scales and helps in detecting features of varying sizes.
These are just a few examples of model architectures that leverage the concatenation operation in CNNs. The use of concatenation allows these models to exploit diverse information from different layers or pathways, leading to improved performance in various computer vision tasks.
Conclusion
In summary, concatenation operation in CNN is a simple yet powerful technique that can be used to combine feature maps from multiple layers or scales to improve the expressive power of the feature representation. By using concatenation, CNNs can learn more complex representations and achieve higher accuracy in various computer vision tasks. Overall, concatenation operation is a versatile tool that can be used in a wide range of applications in deep learning, and its flexibility makes it an essential component of many state-of-the-art deep learning architectures. However, like all techniques in deep learning, it must be used judiciously to avoid the pitfalls of overfitting and increased computational complexity.