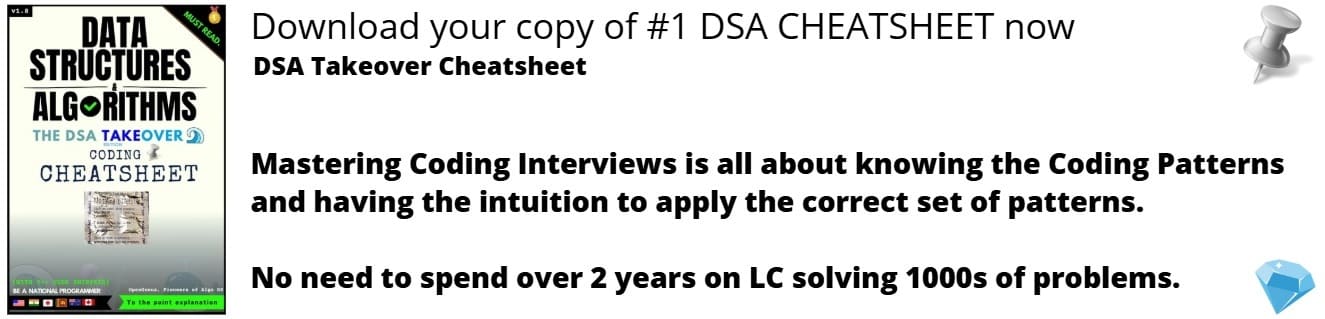
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will explore the topic of Kinetic Heap Data Structures, including its implementation and practical applications.
What we'll discuss:
- Kinetic Data Structure
- Introduction to Kinetic Heap
- Uses of Kinetic Heap
- How can we implement Kinetic Heap?
- Implementation using Java
- How is it different from regular heap?
- Conclusion
Kinetic Data Structure
A kinetic data structure is a type of computer program that is able to store and manage information that changes over time. It automatically adapts to the changes to maintain a certain desired property, such as sorting or organizing the information in a specific way. These types of data structures are often used in real-time or dynamic systems, where the information being stored is constantly changing and traditional data structures would not work well.
Examples of kinetic data structures include Kinetic Heaps and Kinetic B-trees, which are used to efficiently store and manage information in a way that keeps it organized and easy to access.
Introduction to Kinetic Heap
A Kinetic Heap is a type of data structure that combines the features of a binary heap and a kinetic data structure. It is used to efficiently store and retrieve elements that have a specific priority or "energy" level.
The basic idea behind a Kinetic Heap is to use a binary heap to store the elements and use the kinetic structure to automatically adjust the elements' positions in the heap as their priorities change over time. This allows for fast insertion and deletion of elements, as well as quick retrieval of the highest-priority element. Kinetic Heaps are particularly useful in real-time systems where elements have a dynamic priority that changes over time.
An example of this can be a video game where the priority of an object is based on its distance from the camera, and as the camera moves, the Kinetic Heap will automatically reorder the objects to maintain the correct order.
Uses of Kinetic Heap
Here are some of the use cases of Kinetic heap:
-
Real-time systems: Kinetic heap can be used to efficiently store and manage elements in real-time systems where the elements have a dynamic priority that changes over time. For example, a video game may use a Kinetic heap to store and manage objects on the screen, where the priority of an object is based on its distance from the camera.
-
Scheduling algorithms: Kinetic heap can be used in scheduling algorithms to manage the priority of tasks, adjusting the priority as the tasks' deadlines change.
-
Network routing: Kinetic heap can be used in network routing algorithms to manage the priority of packets, adjusting the priority as the packets' paths change.
-
Graph algorithms: Kinetic heap can be used in graph algorithms like Dijkstra's shortest path algorithm, where the priority of a node is based on its distance from the source node and changes as the shortest path is found.
-
Prioritizing data: Kinetic heap can be used to prioritize data based on certain criteria. For example, in a news feed application, articles can be sorted based on the date they were published, the number of views, or the number of likes.
-
Other fields: Kinetic heap can be used in other fields where elements have a dynamic priority that changes over time, such as in robotics, logistics, and transportation.
How can we implement Kinetic Heap?
There are several ways to implement a kinetic heap data structure using the Java programming language.
-
One common approach is to use a binary heap, which is a simple and efficient implementation of a heap. A binary heap can be implemented using an array, where the elements are stored in a complete binary tree. The parent of a node at index i is at index (i-1)/2, and its left and right children are at indices 2_i+1 and 2_i+2, respectively. The heap property can be maintained by using the built-in compareTo method of the Comparable interface or by providing a custom comparator.
-
Another approach is to use a Fibonacci heap, which is a more advanced implementation of a heap that supports faster insertion and deletion operations. A Fibonacci heap can be implemented using a collection of circular doubly-linked lists, where each list represents a tree with a certain number of nodes. The heap property is maintained by merging trees of the same degree and by promoting nodes with a higher key to the root of the tree. The Fibonacci heap can be implemented using a custom comparator.
Yet another approach is to use a pairing heap, which is a simpler variant of the Fibonacci heap that uses a binary tree instead of a collection of circular doubly-linked lists. A pairing heap can also be implemented using a custom comparator.
The choice of implementation will depend on the specific requirements of the application and the desired trade-offs between performance and complexity.
Implementation using Java
Here, it is a simple implementaion of Kinetic Heap using Java language.
import java.util.ArrayList;
import java.util.List;
public class KineticHeap<T extends Comparable<T>> {
// Declare a List of TreeNode to store the elements of the heap
private List<TreeNode<T>> heap;
// Declare a variable to store the current size of the heap
private int size;
public KineticHeap() {
// Initialize the heap with an empty ArrayList
heap = new ArrayList<TreeNode<T>>();
// Initialize the size to 0
size = 0;
}
public void insert(T element) {
// Create a new TreeNode with the element
TreeNode<T> newNode = new TreeNode<T>(element);
// Add the new node to the heap
heap.add(newNode);
// Increment the size of the heap
size++;
// Move the new node up to its correct position in the heap
moveUp(size - 1);
}
public T remove() {
// Check if the heap is empty
if (size == 0) {
throw new IllegalStateException("Cannot remove from an empty heap");
}
// Get the value of the root node (the top of the heap)
T removed = heap.get(0).getValue();
// Replace the root node with the last element in the heap
heap.set(0, heap.get(size - 1));
// Remove the last element from the heap
heap.remove(size - 1);
// Decrement the size of the heap
size--;
// Move the new root node down to its correct position in the heap
moveDown(0);
// Return the value of the removed node
return removed;
}
//Helper method to move a node down the heap to maintain the heap property
private void moveUp(int index) {
// Get the index of the parent node
int parent = (index - 1) / 2;
// While the parent node exists and the value of the current node is greater than the value of its parent
while (parent >= 0 && heap.get(parent).getValue().compareTo(heap.get(index).getValue()) < 0) {
// Swap the current node and its parent
TreeNode<T> temp = heap.get(parent);
heap.set(parent, heap.get(index));
heap.set(index, temp);
// Update the current index to the index of the parent node
index = parent;
// Get the new parent index
parent = (index - 1) / 2;
}
}
// Helper method to move a node down the heap to maintain the heap property
private void moveDown(int index) {
// Calculate the indices of the left and right child nodes
int left = 2 * index + 1;
int right = 2 * index + 2;
// Assume the current node is the largest
int largest = index;
// Check if the left child is within the heap size and has a greater value than the current node
if (left < size && heap.get(left).getValue().compareTo(heap.get(largest).getValue()) > 0) {
largest = left; // update the largest value
}
// Check if the right child is within the heap size and has a greater value than the current node
if (right < size && heap.get(right).getValue().compareTo(heap.get(largest).getValue()) > 0) {
largest = right; // update the largest value
}
// If the largest value is not the current node, swap the current node with its largest child
if (largest != index) {
TreeNode<T> temp = heap.get(largest);
heap.set(largest, heap.get(index));
heap.set(index, temp);
// Recursively call moveDown method on the largest child node to maintain the heap property
moveDown(largest);
}
}
// Private nested class to hold the value of each node in the heap
private static class TreeNode<T> {
private T value;
// Constructor for TreeNode class
public TreeNode(T value) {
this.value = value;
}
// Getter method for the value of the node
public T getValue() {
return value;
}
}
}
This code is an implementation of a Kinetic Heap data structure in Java. A kinetic heap is a data structure that is used to maintain a set of elements with associated priorities, where the priorities can change over time.
The KineticHeap class is a generic class that can take any type of element as long as it implements the Comparable interface. The class uses an ArrayList to store the elements of the heap and an int variable, size, to keep track of the number of elements in the heap.
The class has two main methods, insert(T element)
and remove()
, which are used to insert and remove elements from the heap, respectively. The insert()
method creates a new TreeNode
for the given element and adds it to the end of the ArrayList. It also increases the size of the heap by 1 and calls the moveUp(int index)
method to maintain the heap property. The remove()
method removes the root element of the heap and replaces it with the last element in the ArrayList. It then calls the moveDown(int index)
method to maintain the heap property.
The moveUp(int index)
and moveDown(int index)
methods are helper methods that are used to maintain the heap property. The moveUp()
method compares the element at the current index to its parent and swaps them if necessary. The moveDown()
method compares the element at the current index to its children and swaps them with the largest child if necessary.
The class also includes a private inner class, TreeNode, which is used to store the elements of the heap. The TreeNode class has a single instance variable, value, which stores the value of the element, and a single constructor that takes a value and sets it as the value of the TreeNode.
The time complexity of the insert()
method is O(log n)
and the time complexity of the remove()
method is O(log n)
. This is because in the worst case scenario both of these methods need to traverse the height of the heap.
The overall approach of this code is to maintain the heap property by comparing the element at the current index to its parent and children and swapping them if necessary.
How is it different from regular heap?
One of the advantages of a kinetic heap over a regular heap is its ability to handle a dynamic set of elements. In a regular heap, inserting and removing elements can be a time-consuming operation, because the heap property needs to be maintained after each insertion or removal. However, in a kinetic heap, these operations can be performed in O(log n)
time, which is faster than the O(n)
time required for a regular heap.
Another advantage of a kinetic heap is that it can be used in real-time applications, where elements need to be inserted or removed at any time. Kinetic heap can also be used in applications that require maintaining a set of elements that are constantly changing, such as in some algorithms related to dynamic connectivity, dynamic graph algorithms, etc.
One of the disadvantages of a kinetic heap is that it can be more complex to implement than a regular heap. Additionally, kinetic heap are less common, therefore, it might be harder to find libraries or pre-existing code to use.
Conclusion
In conclusion, this article at OpenGenus discussed Kinetic Heap, a type of data structure that combines the features of a binary heap and a kinetic data structure. It is used to efficiently store and retrieve elements that have a specific priority or βenergyβ level in real-time systems. It also discussed the implementation of Kinetic heap in Java programming language and how it differs from regular heap. Kinetic heap is a specialized data structure that is designed to handle a dynamic set of elements efficiently and can be used in real-time applications and situations where elements are constantly changing.