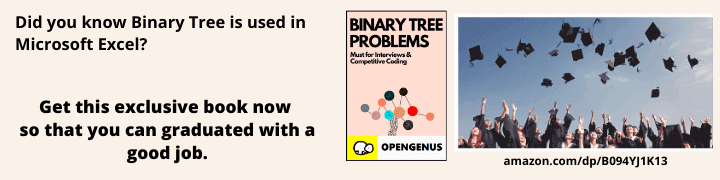
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In Python, "lambda" is a keyword that is used to create small, anonymous unnamed functions. These functions are also called lambda functions. They are defined using the "lambda" keyword followed by one or more arguments, a colon, and a single expression that is evaluated when the function is called. The expression is the return value of the function.
Some other things to know about lambda functions in Python are:
1)Lambda functions can take any number of arguments, but they can only have one expression. This means that they are limited in terms of functionality compared to regular (named) functions.
2)The expression in a lambda function can be any valid Python expression, including function calls and mathematical operations.
Lambda functions do not have a return statement; the value of the expression is automatically returned.
3)Lambda functions do not have a name, so they cannot be referred to after they are defined. They are typically used as arguments to other functions.
1) What is a lambda function in Python and what is it used for?
Answer: A lambda function is a small anonymous function in Python. It is defined using the "lambda" keyword and does not have a name. It is typically used for short, throwaway functions, such as for sorting or for applying a function to a list of elements.
2)How do you define a lambda function in Python?
Answer: A lambda function is defined using the "lambda" keyword, followed by one or more arguments, a colon, and the function body.
For example:lambda x: x + 1
3)What is the difference between a lambda function and a regular Python function?
Answer: A lambda function is a small, anonymous function that does not have a name, whereas a regular Python function is defined using the "def" keyword and has a name. Lambda functions are also limited in functionality compared to regular functions, as they can only contain a single expression.
4)What is the syntax of a lambda function in Python?
Answer: The syntax of a lambda function in Python is as follows:
lambda x: x*2
5)How do you use a lambda function in Python?
Answer: Lambda functions can be used in a variety of ways, including as arguments to other functions (such as the built-in "map" or "filter" functions), or to create short, throwaway functions for one-time use.
6)What are some examples of when you would use a lambda function in Python?
Answer: Some examples of when you might use a lambda function in Python include:
Sorting a list of elements by a specific attribute
Applying a function to a list of elements
Creating a short, throwaway function for one-time use
7)What is the difference between a lambda function and a closure in Python?
Answer: A lambda function is a small, anonymous function that does not have a name, whereas a closure is a function object that remembers values in the enclosing scope even if they are not present in memory.
8)How does a lambda function differ from a list comprehension in Python?
Answer: A lambda function is a small, anonymous function that does not have a name, whereas a list comprehension is a more concise way to create a list in Python. While lambda functions can be used to perform operations on a list, list comprehensions are a more concise and readable way to perform those same operations.
9)Can a lambda function be used in place of a regular function in all cases in Python?
Answer: No, lambda functions are limited in functionality compared to regular functions and are typically used for short, throwaway functions, so they can not be used in place of a regular function in all cases.
10)Are lambda functions slower than regular functions in Python?
Answer: The performance of lambda functions is similar to that of regular functions in Python, so there should not be a noticeable difference in speed. However, as lambda functions are limited in functionality compared to regular functions, they may be slower in cases where they are used to perform more complex operations.
11)Can a lambda function have a return statement?
Answer: Yes, if it is a single expression function
12)What is the use of lambda function with filter() in Python?
Answer: To filter out elements of a list that do not satisfy a certain condition.
13)When is it appropriate to use a lambda function in Python?
Answer: When a function is going to be used only once.
14)Can a lambda function be defined inside a regular function in Python?
Answer: Yes, lambda functions can be defined inside regular functions in Python.
15)What is the difference between a lambda function and a generator expression in Python?
Answer: A lambda function is a small anonymous function while a generator expression creates an iterator.
16)What keyword is used to define a lambda function in Python?
a) def
b) lambda
c) func
d) anonymous
Answer: b) lambda
17)How many expressions can a lambda function contain in Python?
a) 1
b) 2
c) 3
d) Unlimited
Answer: a) 1
Explaination: A lambda function in Python can contain only one expression.
In lambda functions, the expression is automatically returned by the function, there is no need for a return statement.
18)Which of the following is a use case for a lambda function in Python?
a) Sorting a list of elements
b) Creating a user interface
c) Defining a class
d) Opening a file
Answer: a) Sorting a list of elements
19)What type of function is a lambda function in Python?
a) Anonymous
b) Named
c) Both
d) None of the above
Answer: a) Anonymous
Explaination: A lambda function in Python is an anonymous function, meaning it is a function without a name. It is defined using the "lambda" keyword, followed by one or more arguments and an expression. Lambda functions are typically used as arguments to other functions, such as the built-in "map()" and "filter()" functions, and can be used to create small, one-time-use functions.
20)What built-in function in Python can take a lambda function as an argument?
a) print()
b) map()
c) open()
d) sorted()
Answer: b) map()
Explaination: The built-in function "map()" in Python can take a lambda function as an argument. The "map()" function applies a given function to each item of an iterable (such as a list, tuple, or string) and returns an iterator that yields the results.
Code snippet:
numbers = [1, 2, 3, 4, 5]
squared_numbers = map(lambda x: x**2, numbers)
print(list(squared_numbers))
21)What is the difference between a lambda function and a closure in Python?
a) A lambda function is a small anonymous function while a closure is a function object that remembers values in the enclosing scope
b) A closure is a small anonymous function while a lambda function is a function object that remembers values in the enclosing scope
c) Both are the same
d) None of the above
Answer: a) A lambda function is a small anonymous function while a closure is a function object that remembers values in the enclosing scope
Explaination: A lambda function in Python is a small, anonymous function defined using the "lambda" keyword. It can have one or more arguments and an expression and is often used as an argument to other functions such as the built-in "map()" and "filter()" functions.
A closure, on the other hand, is a function object that remembers values in the enclosing scope, even if they are not present in memory. A closure function is a nested function which has access to a free variable from its containing function that has finished its execution. Closures are used in cases where a function has to remember some state, such as in decorators or implementing a state machine.
Code Snippet:
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
closure = outer_function(10)
print(closure(5))
22)How does a lambda function differ from a list comprehension in Python?
a) A lambda function is a small anonymous function while a list comprehension is a more concise way to create a list
b) A list comprehension is a small anonymous function while a lambda function is a more concise way to create a list
c) Both are the same
d) None of the above
Answer: a) A lambda function is a small anonymous function while a list comprehension is a more concise way to create a list
23)Can a lambda function have a return statement?
a) Yes
b) No
c) Only if it's a single expression
d) It depends
Answer: c) Only if it's a single expression
Explaination: A lambda function in Python can have a return statement, but only if it is a single expression. This means that the lambda function can only consist of one expression, and not multiple statements.
The expression of the lambda function is automatically returned by the function, thus the return statement is not necessary.
24)How to pass multiple arguments to a lambda function in Python?
a) Using commas
b) Using semicolons
c) Using colons
d) Using parentheses
Answer: a) Using commas
Explaination: Multiple arguments can be passed to a lambda function in Python by using commas to separate them.
The arguments are listed after the lambda keyword, and the syntax is similar to that of a regular function definition.
Code Snippet :
lambda lst, key, value: [dict(i, **{key: value}) for i in lst]
25)When is it appropriate to use a lambda function in Python?
a) When a function is going to be used only once
b) When a function is going to be used multiple times
c) When a function is complex
d) When a function is simple
Answer: a) When a function is going to be used only once
Explaination: It is appropriate to use a lambda function in Python when the function is going to be used only once. This is because lambda functions are anonymous, meaning they do not have a name and cannot be reused. They are typically used as arguments to other functions, such as the built-in "map()" and "filter()" functions, or as a quick way to define a small function for a specific task.
Code Snippet:
numbers = [1, 2, 3, 4, 5]
even_numbers = filter(lambda x: x % 2 == 0, numbers)
print(list(even_numbers))
26)What is the use of lambda function with map() in Python?
a) To apply a certain function to all elements of a list
b) To filter out elements of a list that do not satisfy a certain condition
c) To sort a list of elements
d) To perform mathematical operations on a list
Answer: a) To apply a certain function to all elements of a list
27)How can you use a lambda function to filter a list of integers?
Answer: Use the filter() function and pass the lambda function as the first argument, followed by the list of integers. The lambda function should return a Boolean value indicating whether the element should be included in the filtered list.
28)How can you use a lambda function to modify the values of a dictionary?
Answer: Use the map() function and pass the lambda function as the first argument, followed by the dictionary. The lambda function should take a key-value pair and return a modified key-value pair.
29)How can you use a lambda function to sort a list of strings alphabetically?
Answer: Use the sort() function and pass the lambda function as the key argument. The lambda function should take a string as an argument and return the string in lowercase.
30)How can you use a lambda function to calculate the sum of the elements in a list of integers?
Answer: Use the reduce() function from the functools module and pass the lambda function as the first argument, followed by the list of integers. The lambda function should take two arguments, the current total and the next element in the list, and return the updated total.
31)How can you use a lambda function to find the minimum value in a list of integers?
Answer: Use the min() function and pass the lambda function as the key argument, followed by the list of integers. The lambda function should take an integer as an argument and return the integer.
32)What are the limitations of lambda function in Python?
Answer: 1) Limited Expressiveness: Lambda functions are limited to a single expression and cannot contain statements or multiple expressions. This makes them less expressive and less powerful than traditional functions.
2)No Default Arguments: Lambda functions do not support default arguments, which can make them less convenient to use in certain situations.
3)Limited Use Cases: Lambda functions are typically used for simple operations and are not well-suited for complex or large-scale tasks.
33)Write a program to find lambda function to multiply all elements in a list by a given number.
numbers = [1, 2, 3, 4, 5]
x = 2
result = list(map(lambda a: a*x, numbers))
print(result)
Explanation:
1)The map() function is used to apply a specified function (in this case a lambda function) to each element of an iterable (in this case the list of numbers).
2)The lambda function takes a single argument a, which represents each element of the list, and multiplies it by x.
3)Then the list() function is used to convert the map object to a list and the result variable contains the final list of numbers after multiplying each element with the given number.
34)How do we use the Lambda function in apply()?
Answer : The apply() function in Pandas allows you to apply a function to a specific axis of a DataFrame. The function can be a built-in function, a user-defined function, or a lambda function.
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df['A'] = df.apply(lambda x: x['A'] ** 2, axis=1)
print(df)
Explanation:
Here, we are using the apply() function to apply the lambda function on each row of the DataFrame (axis=1). The lambda function takes a single argument x, which represents each row of the DataFrame, and squares the value of the 'A' column (x['A'] ** 2).
The result is a new DataFrame with the squared values of the 'A' column.
35)When is it advised not to use a Lambda function?
Answer: 1)When the logic is too complex: Lambda functions are limited to a single expression, so if the logic is too complex or requires multiple expressions, it is best to use a regular function definition instead.
2)When the function needs to be reused: Since lambda functions are anonymous, they cannot be reused, so if the function needs to be reused in multiple places, it is best to use a regular function definition instead.
3)When the function requires default arguments: Lambda functions do not support default arguments, so if the function requires default arguments, it is best to use a regular function definition instead.
36)How is lambda assigned to a variable?
Answer: In Python, a lambda function can be assigned to a variable just like any other value. This allows you to use the lambda function in multiple places, and also makes the code more readable.
squared = lambda x: x**2
print(squared(5)) # Output: 25
print(squared(10)) # Output: 100
We are assigning the lambda function to a variable squared. Then we can use the variable squared as a function just like any other function. You can also pass the lambda function as an argument to other functions that accept a function as an argument, like map, filter, and reduce.
37)Is multithreading possible in lambda?
Answer :In Python, it is possible to use multithreading with lambda functions, although it is not a very common use case. Multithreading allows you to run multiple threads concurrently, which can be useful for improving the performance of certain types of tasks.
When using multithreading with lambda functions, you should take into account the Global Interpreter Lock (GIL) of python, which is a mechanism used by the interpreter to synchronize access to Python objects.
38)What happens if a lambda function in python fails?
Answer :If a lambda function fails in Python, it will raise an exception just like any other function. The exception will be propagated to the calling code, and if it is not handled, it will cause the program to crash.
lambda_function = lambda: 1/0
try:
lambda_function()
except ZeroDivisionError as e:
print("An error occurred: ", e)
It's important to note that since lambda functions are anonymous, it's harder to trace the origin of the error, thus it's recommended to add a comment or a meaningful name when defining the lambda function, to make it easier to debug.
39)Is Lambda function in python synchronous or asynchronous?
Answer: In Python, a lambda function is synchronous, meaning that it runs in the same thread as the calling code and blocks the execution of the calling code until it completes.
A synchronous function runs in a linear fashion, it starts, runs to completion, and returns the result. While the calling code waits for the lambda function to complete, it cannot do any other work.
If you call a lambda function that performs a long-running task, such as a network call or a complex calculation, the calling code will be blocked until the lambda function completes.
40)How do you handle errors in lambda function in python?
Answer : 1)Try-except block: You can use a try-except block to catch exceptions raised by the lambda function and handle them appropriately.
2)Return an error: You can return an error response from the lambda function, such as a tuple or a dictionary containing an error message and status code.
3)Use a logging library: You can use a logging library to log errors and exception raised by the lambda function, this way you can have a more detailed error message and track the error.
41)Can you use a lambda function with a decorator in Python?
Answer: Yes, a lambda function can be used with a decorator in Python. A decorator is a function that takes another function as its argument and extends the behavior of that function. In this case, the lambda function is passed as an argument to the decorator function, and the decorator function returns the lambda function with the added behavior.
42)How can you use a lambda function to find the intersection of two lists without using the set() or & operator?
Answer:
list1 = [1, 2, 3, 4, 5]
list2 = [3, 4, 5, 6, 7]
intersection = lambda x, y: [val for val in x if val in y]
print(intersection(list1, list2))
The lambda function uses a list comprehension to iterate through the values in the first list and check if the value is also in the second list. The values that are present in both lists are added to a new list and returned.
43) How can you use a lambda function to find the Cartesian product of two lists without using itertools.product()?
Answer:
list1 = [1, 2, 3]
list2 = ['a', 'b']
cartesian_product = lambda x, y: [(a, b) for a in x for b in y]
print(cartesian_product(list1, list2))
The lambda function uses a nested list comprehension to iterate through the values in the first list and the second list, and creates a tuple of each combination of values. The resulting list of tuples represents the Cartesian product of the two lists.
44)what is the difference between the filter and reduce function in python lambdas?
Answer: The filter() function in Python is used to filter a sequence (e.g. list, tuple, etc.) based on a certain condition, and return an iterator that only contains the elements that satisfy the condition.
The reduce() function in Python is used to apply a binary function to a sequence in a cumulative way, so as to reduce the sequence to a single value.
The filter() function is used to filter elements from an iterable based on a given condition and the reduce() function is used to apply a binary operation to all elements in an iterable so as to reduce them to a single value.
45)How can a lambda function be used to sort a list of items?
a) list.sort(lambda x: x)
b) list.sort(key=lambda x: x)
c) list.sort(by=lambda x: x)
d) list.sort(func=lambda x: x)
Answer: b) list.sort(key=lambda x: x)
Explaination: A lambda function can be used to sort a list of items by passing it as the "key" argument to the built-in "sort()" method of a list.
The key argument is a function that takes one input and returns one output. This function is applied to each element of the iterable (list in this case) before sorting, and the returned values are used for sorting.
Code snippet:
words = ['foo', 'bar', 'baz', 'qux', 'quux']
words.sort(key=lambda x: len(x))
print(words)
46)What is the correct syntax for defining a lambda function in Python?
a) def lambda_func():
b) lambda:
c) lambda func:
d) def func():
Answer: b) lambda:
Explaination: The keyword lambda is used to define the function, followed by one or more arguments separated by commas. The arguments are followed by a colon (:) and the function's expression, which is evaluated and returned when the function is called.
Code Snippet:
lambda x, y: x + y
47)What is the output of the following code?
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = filter(lambda x: x % 2 == 0, numbers)
print(list(result))
a) [1, 3, 5, 7, 9]
b) [2, 4, 6, 8, 10]
c) [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
d) []
Answer: b) [2, 4, 6, 8, 10]
48)What is the output of the following code?
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
result = reduce(lambda x, y: x * y, numbers)
print(result)
a) 3628800
b) 0
c) 10
d) 55
Answer: a) 3628800
49)What is the main use of lambda functions in Python?
a) To create anonymous functions that can be used only once
b) To define small, throwaway functions for specific tasks
c) To create complex, multi-line functions
d) To create reusable functions that can be used multiple times
Answer: b) To define small, throwaway functions for specific tasks
Explaination: The main use of lambda functions in Python is to define small, throwaway functions for specific tasks. These functions are known as anonymous functions because they don't have a name.
They are used to perform a specific, often simple operation, and are typically passed as an argument to other functions, such as the built-in map() and filter() functions. They are often used to quickly define small functions for one-time use, without the need to define a separate, named function.
50)How can a lambda function be passed as an argument to the map() function?
a) map(lambda x: x * 2)
b) map(x: x * 2)
c) map(function(x) x * 2)
d) map(x => x * 2)
Answer: a) map(lambda x: x * 2)
With the help of this article at OpenGenus, you will have an idea on what lambda function in python is, what it does, and how to use it in python. These questions can help you to prepare and revise for this topic before an interview.