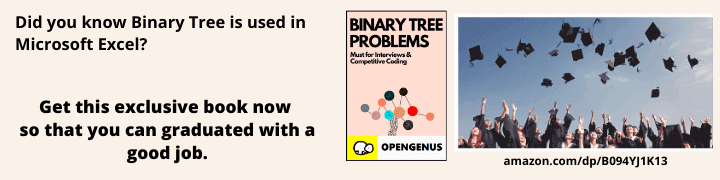
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In software engineering, a design pattern is a general repeatable solution to a commonly occurring problem in software design. Design patterns can speed up the development process by providing tested, proven development paradigms. This makes it a very in demand knowledge in the tech industry. This article contains top interview questions on Design Pattern.
Table of Content
- Multiple Choice Questions
- Descriptive Questions
- Practical Questions
Multiple Choice Questions
1. In which of the following pattern, a class behavior changes based on its state?
a. State Pattern
b. Null Object Pattern
c. Strategy Pattern
d. Template Pattern
Ans: Option a. State Pattern
Explanation: State pattern is a behavioral design pattern. It is used when an Object changes its behavior based on its internal state.
2. Which of the below isn't a legitimate design pattern?
a. Singleton
b. Java
c. Command
d. Factory
Ans: Option b. Java
Explanation: In software design, a design pattern could be a generic, repeatable solution to a standard issue. There are numerous patterns that may be applied to common coding issues.
3. Which design pattern ensures that just one object of particular class gets created?
a. Singleton pattern
b. Filter pattern
c. State pattern
d. Bridge pattern
Ans: Option a. Singleton pattern
Explanation: Singleton pattern involves one class which is responsible to form an object while ensuring that only 1 object gets created. This class provides the way to access the object which could be accessed directly without the nexessity to instantiate another object of the identical class.
4. Which design pattern allows access to all the objects in a collection?
a. Iterator pattern
b. Facade pattern
c. Builder pattern
d. Bridge pattern
Ans: Option a. Iterator pattern
Explanation: Iterator pattern allows access to the elements of a collection object in serial manner without the requirement to understand its underlying representation.
5. What does MVC pattern stands for?
a. Mock View Control
b. Model view Controller
c. Mock View Class
d. Model View Class
Ans: Option b. Model view Controller
Explanation: Model–view–controller (MVC) refers to a software architectural pattern commonly used for developing user interfaces that divide the related program logic into three interconnected elements.
6. Which of the following design patterns works on data and action taken based on the data provided?
a. Singleton pattern
b. Command pattern
c. MVC pattern
d. Facade pattern
Ans: Option b. Command pattern
Explanation: Command pattern refers to a data driven design pattern which comes under behavioral pattern design. A request is enclosed within an object as command and passed to the invoker object. The invoker object looks for an object which could handle this command and passes this command to the object which runs the command.
7. Which design pattern provides a single class that provides simplified methods required by client and delegates call to those methods?
a. Adapter pattern
b. Builder pattern
c. Facade pattern
d. Prototype pattern
Ans: Option c. Facade pattern
Explanation: Facade pattern conceals the complexities of the system and provides an interface to the user to access the system.
8. Which design pattern suggests multiple classes through which request is passed and multiple but only relevant classes perform operations on the request?
a. Singleton pattern
b. Chain of responsibility pattern
c. State pattern
d. Bridge pattern
Ans: Option b. Chain of responsibility pattern
Explanation: Chain of responsibility pattern is a behavioral pattern that creates a series of receiver objects for a specific request. The sender and receiver of request are decoupled supported the sort of request.
9. Design patterns are divided into how many fundamental groups.
a. 2
b. 5
c. 3
d. 8
Ans: Option c. 3
Explanation: Design patterns are divided into 3 fundamental groups - Behavioral pattern, Creational pattern, and Structural pattern.
10. Which of the following describes the Interpreter pattern correctly?
a. Here, a class represents functionality of another class.
b. It creates a chain of receiver objects for a request.
c. It provides a way to evaluate language grammar or expression.
d. A request is wrapped under an object as command and passed to invoker object in this pattern.
Ans: Option c. It provides a way to evaluate language grammar or expression.
Explanation: Interpreter pattern is a behavioral pattern that provides a way to evaluate language grammar or expression. This pattern implements an expression interface which tells to interpret a particular context.
11. What represents the (static) structure and (dynamic) behavior of pattern?
a. Name
b. Application
c. Consequences
d. Form
Ans: Option d. Form
Explanation: Form represents (static) structure and (dynamic) behavior of pattern.
12. What benefits does patterns provide?
a. Novice designers can learn solution patterns that experts use, without needing design experience.
b. Expert designers can can broaden their repertoire of patterns and deepen their understanding of the known patterns.
c. All of the mentioned
d. None of the mentioned
Ans: Option c. All of the mentioned
Explanation: Both novice and expert designers benefit from design patters.
13. Which one of these design patterns focus on the design patterns movement?
a. Architectural Styles
b. Mid-Level Design Patterns
c. Data Structures and Algorithms
d. Programming Idioms
Ans: Option b. Mid-Level Design Patterns
Explanation: Mid-Level Design Patterns emphasize on design patterns movement.
14. Which type of design patterns are specifically concerned with the presentation tier?
a. Creational Design Patterns
b. Structural Design Patterns
c. Behavioral Design Pattern
d. J2EE Design Patterns
Ans: Option d. J2EE Design Patterns
Explanation: J2EE Design Patterns are specifically concerned with the presentation tier.
15. If you wish to reduce development cost by reusing methods, which design pattern would you choose?
a. Adapter Pattern
b. Singleton Pattern
c. Delegation pattern
d. Immutable Pattern
Ans: Option c. Delegation pattern
Explanation: The delegation pattern is a design pattern in OOP where an object delegates that task to an associated helper object, rather than performing one among stated tasks.
16. Which mechanism is applied to use a design pattern in an OO system?
a. Inheritance
b. Composition
c. All of the mentioned
d. None of the mentioned
Ans: Option c. All of the mentioned
Explanation: Inheritance is used to make an existing design pattern a template for a new subclass. Composition leads to aggregate objects.
17. The recurring aspects of designs in software developemtn are called design ______.
a. patterns
b. documents
c. structures
d. methods
Ans: Option a. patterns
Explanation: A pattern is an outline of a reusable approach to a general problem encountered in a specific context.
18. Which of these patterns prevents one from creating more than one instance of a variable?
a. Factory Method
b. Singleton
c. Observer
d. None of the mentioned
Ans: Option b. Singleton
Explanation: In singleton pattern, the class itself is accountable for keeping track of its instance. Thus it ensures that only one instance is formed.
19. Which of these design patterns defines one-to-many dependency among objects?
a. Singleton pattern
b. Facade Pattern
c. Observer pattern
d. Factory method pattern
Ans: Option c. Observer pattern
Explanation: Observer pattern defines one-to-many dependency among objects. When one object modifies its state, all of its dependents are notified.
20. Which of the following involves communication between objects?
a. J2EE Design Patterns
b. Creational Design Pattern
c. Behavioral Design Patterns
d. Structural Design Patterns
Ans: Option c. Behavioral Design Patterns
Explanation: Behavioral design patterns identify common communication patterns among objects. They help in increasing flexibility to carry out communication.
Descriptive Questions
1. What are design patterns?
In software development, design patterns are the reusable solutions that solve commonly occurring problems. These problems may include repetitive code, redundant functions and logic, etc. These help to avoid wasting considerable effort and time required by the developers while developing software. Design patterns are commonly employed in object-oriented software products by incorporating best practices and promoting reusability for developing robust code.
2. What are the advantages of Java Design Patterns?
Design patterns are template-based reusable solutions to help developers while software development. In Java, the design patterns are flexible. They help to identify unwanted repetitive code. The architecture of the software can be customized as per the requirements.
Advantages:
- They're reusable and may be utilized in multiple projects.
- They supply template solutions for outlining system architecture.
- They supply transparency to software design.
- They're well-tested and proven means of developing robust solutions effortlessly.
3. What are the types of design patterns in Java?
The three types of design patterns are:
- Creational Patterns: These patterns provide the flexibility to choose between creating objects by hiding the logic. The objects built are decoupled from the implemented system. E.g. - Builder design, Prototype design, Factory design pattern, Singleton design, Abstract Factory design.
- Structural Patterns: These patterns help in defining how the structures of classes and objects should be like for outlining the composition between classes, interfaces and objects. E.g. - Facade design, Decorator design, Adaptor design, proxy design etc.
- Behavioural Patterns: These patterns help to define how the objects should communicate and interact with each other. E.g. - Iterator pattern, Observer pattern, Command pattern, Strategy pattern, etc.
4. What is Gang of Four (GOF)?
In 1994, four authors Richard Helm, Ralph Johnson, Erich Gamma, and John Vlissides published the Design Patterns - Elements of Reusable Object-Oriented Software which introduced the concept of Design Pattern in Software development. These authors are collectively called Gang of Four (GOF).
5. What Is Inversion of Control?
In software engineering, Inversion of Control is a principle which transfers the control of objects or portions of a program to a container or framework. It is frequently used in the context of object-oriented programming.
In contrast with conventional programming, in which our custom code makes calls to a library, IoC enables a framework to take control of the flow of a program and make calls to our custom code. To enable this, frameworks use abstractions with additional inbuilt behavior. If we wish to input our own behavior, we could extend the classes of the framework or plugin our own classes.
We can achieve Inversion of Control through various mechanisms such as: Service Locator pattern, Factory pattern, Strategy design pattern, and Dependency Injection (DI).
6. What are the advantages of Inversion of Control?
- It helps in decoupling the execution of a task from its implementation
- It makes it easier to switch between various implementations
- It provides greater modularity of a program
- It provides greater ease in testing a program by isolating a component or mocking its dependencies, and allowing components to communicate through contracts
7. What are some of the design patterns used in Java’s JDK library?
Some design patterns used in the JDK library are:
- Decorator pattern used by Wrapper classes.
- Singleton pattern used in classes like Runtime and Calendar.
- Factory pattern used for methods like Integer.valueOf function in wrapper classes.
- Observer pattern used for handling event frameworks like swing, awt, etc.
8. How are design principles different from design patterns?
-
Design principles are the rules that are followed while designing software systems for any platform using any programming language. SOLID principles are the guidelines to develop extensible, robust, and scalable software systems. These principles apply to all aspects of programming.
-
Design Patterns are the reusable template solutions for common problems that can be customized according to the problem requirements. These are well-implemented approaches that are tested and are secure. E.g., Singleton pattern, Factory Design Pattern, Strategy patterns.
9. How are design patterns different from algorithms?
Algorithms and Design Patterns both outline typical solutions to a variety of issues. The major distinction, though, is that while a design pattern offers a high-level description of any solution, an algorithm specifies a precise set of steps for achieving a goal. Even though two separate problems may use the same design pattern, the implementation logic will vary depending on the needs.
10. What are the SOLID Principles?
SOLID are the Object-Oriented principles introduced by Robert C. Martin in his paper “Design Principles and Design patterns”. SOLID stands for:
- S - Single Responsibility Principle (SRP): This rule assures that each class or module is responsible only one functionality. Any change in class should have only one justification.
- O - Open Closed Principle (OCP): Every class has the option of being extended but not modified. Here, we can increase the behaviour of the entities without changing anything in the existing source code.
- L - Liskov Substitution Principle(LSP): According to the LSP principle, subtype instances can take the place of objects without impacting the program's accuracy.
- I - Interface Segregation Principle (ISP): According to this concept, many interfaces can be used that are specific to the needs of the customer rather than developing only one broad interface. Customers shouldn't be pressured to use functionalities that are not needed.
- D - Dependency Inversion Principle: According to this principle, the high-level modules should depend on the abstractions rather than depending on the lower level modules or concrete implementations.
11. What is a Factory Design Pattern?
In Java, one of the most commonly used design patterns is the factory pattern. It falls under the category of a creational pattern as it offers one of the finest ways to make an object. The Virtual Constructor is another name for the Factory Method Pattern.
In the factory design, we build objects without disclosing the creation logic to the client and make use of a standard interface to refer to recently created objects.
According to the factory method pattern or factory pattern, an interface or abstract class has to be specified to create an object. The subclasses choose which class to instantiate.
12. State the advantages of a factory design pattern.
- By utilising interfaces and factory classes, this design pattern enables the application's development mechanism to be concealed.
- By employing mock or stubs, it enables testing of the application's seamlessness.
- It enables flexibility in the execution of functions when new classes are created, which introduces loose coupling in the application.
13. What is a Adapter Design Pattern?
The adapter pattern comes under structural pattern since it combines the functionality of two different interfaces. The adapter pattern transforms a class's interface into a different interface according to clients expectations. Classes that would not have been able to interact due to conflicting interfaces can now do so because of adapters. In this design, one class is responsible to connect the capabilities of separate or incompatible interfaces. It functions as a link between two disparate interfaces.
14. Give an example for Adapter Design Pattern.
Consider that an AudioPlayer class implements a MediaPlayer Interface. By default, the AudioPlayer can play mp3 files. The MP4Player class, which plays mp4 files, and WAVPlayer, which plays wav formats, both implement the AdvancedPlayer interface. The MediaAdapter class, which implements the MediaPlayer Interface and leverages the AdvancedPlayer objects for playing the necessary formats, is used to make the AudioPlayer class play other formats.
15. What is a Singleton Design Pattern?
One of the simplest design patterns in Java is the singleton pattern. This kind of design pattern falls under the category of a creational pattern because it offers one of the finest ways to construct an object. It is a design pattern that limits the number of objects that can be created from an instance of a class to one.
In this pattern, a single class is responsible for creating an object while ensuring that only one object is produced. This class offers a method of directly accessing the class's sole object, eliminating the need to instantiate it first.
16. How can you create Singleton class in java?
It involves two steps. To prevent the class from being instantiated by the new operator, first make the constructor private. If null, build the object and return it via a method; otherwise, return an object of the object.
17. How can you achieve thread-safe singleton patterns in Java?
In the presence of several threads, object initialization is made easier by the creation of a thread-safe singleton class. Many methods can be used to do it:
-
Using Enums: Since Java already comes with built-in synchronisation functionality, using Enums is the easiest way to create a thread-safe singleton class in Java. Enums are always final by default, which aids in avoiding repeated initializations during serialisation.
-
Using Static Field Initialization: An instance can also be produced during class loading to create a thread-safe singleton. Static fields are used to do this since the Classloader ensures that instances are initialised at the time of class loading and are hidden until they are fully constructed.
-
Using the synchronised keyword: The getInstance method allows us to utilise the synchronised keyword. Since we use synchronised keyword, the object initialization is thread-safe and we can achieve lazy initialization using this method.
The only issue is that when using several threads, the performance suffers because the entire process is synchronised. -
Double-check locking: In this case, rather than making the entire method synchronised, a synchronized block of code inside the getInstance method is used. This guarantees that just a small number of threads must wait just once, preventing performance degradation.
18. What is a Chain of Responsibility pattern?
In chain of responsibility, a request is sent to a chain of objects by the sender. Any object in the chain can respond to the request.
The pattern suggests that just "prevent coupling the sender and receiver of a request by allowing multiple objects a chance to handle the request,". The Chain of Responsibility design pattern, for instance, is used in the money transaction by an ATM.
In other words, each receiver typically contains a reference to another receiver. The request is passed to the next receiver if the first object is unable to handle it, and so on.
19. State the advantages of Chain of Responsibility pattern.
Advantages:
- When responsibilities are given to objects, it improves flexibility.
- It causes coupling to reduce.
- It enables a group of classes to function as one; via composition, events generated in one class can be forwarded to other handler classes.
20. What is Decorator Design Pattern?
A structural pattern called the decorator design pattern enables new features to be added to an existing object without altering its structure. By maintaining the class method signatures, this pattern generates a new class called the decorator class that serves as a wrapper for the original class. In order to implement the wrapper, it uses abstract classes and interfaces with composition. As we categorise functionalities into classes with different issues, they are mostly utilised to implement the Single Responsibility Principle (SRP). This pattern resembles the chain of responsibility pattern, in terms of structure.
Implementation of the decorator design pattern:
- Develop an interface and concrete classes that use it.
- Build a abstract decorator class that implements the interface.
- Build a concrete decorator class that extends the abstract class created in the previous step.
- Check the results after using the concrete decorator class to decorate the interface objects.
21. What is an Observer Design Pattern?
One of the behavioural design pattern used for specifying the one-to-many interdependence between the objects is the observer design pattern. It is especially helpful when we wish to be informed of any changes to an object's state. In this pattern, each dependant item receives automatic notification whenever the state of one object is changed. The dependents are known as the Observers, whilst the monitored object is known as the Subject. Java's java.util.Observable
class and java.util.Observer
interface can be used to implement this pattern.
22. What is Bridge pattern?
When we want to separate an abstraction from implementation so that they can change independent of each other, we employ a Bridge. Because it creates a bridge structure between implementation class and abstract class, this sort of design pattern falls within the structural pattern category.
With the use of an interface that serves as a bridge, concrete classes using this pattern can function independently of interface implementer classes. Structure changes to either type of class can be made without having an impact on the other.
23. What is Facade pattern?
The facade pattern conceals the system's complexity and offers the client an interface via which they can access the system. Since it adds an interface to an existing system to conceal its complexity, this form of design pattern falls under the structural pattern category.
This pattern makes use of a single class that offers clients the simplified methods they need while delegating calls to methods of pre-existing system classes.
24. What is Strategy pattern?
A class's behaviour or algorithm can be altered at runtime under the Strategy pattern.
In Strategy pattern, we design objects that represent different strategies as well as a context object whose behaviour changes depending on the strategy object. The context object's execution algorithm is modified by the strategy object.
25. What is Builder pattern?
According to the Builder Pattern, a complex object is created from simple ones by applying a step-by-step technique. It is typically used when an object cannot be produced in a single step, such as when a complex object is being de-serialized.
In order for the same production process to provide different representations, the builder pattern seeks to separate the development of a complicated object from its representation. It is used to step by step build complex objects, with the final step returning the object. For the purpose of producing various representations of the same object, the construction process for an object should be generic.
26. Mention advantage of Builder design pattern in Java?
The following are some benefits of builder design patterns.
- It provides enhanced control over the process of cosntruction and makes it easier to distinguish between the making of an object and its representation.
- The constructor parameter is condensed and presented in method calls that are very readable.
- The object is instantiated in a complete state always when using a design pattern. * Immutable objects can be swiftly built in the object construction process using the Builder design pattern.
27. How is Bridge pattern is different from the Adapter pattern?
The Adapter pattern aims to make one or more classes' interfaces resemble one another. Whereas, the Bridge pattern separates a class's interface from its implementation so that the implementation can be changed or replaced without affecting the client code.
28. What are J2EE Patterns?
These design patterns are specifically concerned with the presentation tier. These patterns are identified by Sun Java Center.
Examples:
- MVC Pattern
- Business Delegate Pattern
- Composite Entity Pattern
- Data Access Object Pattern
- Front Controller Pattern
- Intercepting Filter Pattern
- Service Locator Pattern
- Transfer Object Pattern
29. What is MVC pattern?
The Model-View-Controller Pattern, also known as the MVC pattern, is employed to divide the concerns of an application.
- The Model - The Model does not include any logic detailing how to show the data to a user; it merely holds the pure application data.
- The View - The user can see the data of the model presented by the View. The view is aware of how to get to the data, but it is unaware of what this data signifies or how the user might be able to alter it.
- The Controller - Between the model and the view, lies the controller. It listens to events that the view (or some other external source) triggers and responds to them appropriately. The response is often to invoke a method on the model. The outcome of this action is then immediately reflected on the view since the view and the model are linked by a notification system.
30. What would happen if we do not have a synchronized method for returning Singleton instance in a multi-threaded environment?
If we have a non-synchronized method for returning instances in a multi-threaded environment, there is a possibility that the method will create more than one instance. Assume that there are two threads and that they both enter the condition to see if the instance is already present. Both threads will create instance of the class upon discovering that the instance has not been built, which violates the Singleton pattern's principles. Therefore, using synchronised checks in a multi-threaded context is advised.
Practical questions
1. Which Design Pattern Does The Following Code Snippet Implement?
public class A{
private static A instance = null;
private A(){}
public static A getInstance(){
if(instance == null){
instance = new A();
}
return instance;
}
}
The above code implements a Singleton design pattern. It forbids a class from creating multiple instances. A class is defined so that just one instance of the class is created.
2. What will be the output of the following code?
interface A{
void printResult();
}
class B implements A {
@Override
public void printResult()
{
System.out.println("Class B");
}
}
class C implements A {
@Override
public void printResult()
{
System.out.println("Class C");
}
}
class Factory {
public A showResult(int i)
{
if(i == 0){
return new B();
}
else{
return new C();
}
}
}
class FactoryPattern{
public static void main(String[] args) {
Factory fact = new Factory();
A obj = fact.showResult(0);
obj.printResult();
}
}
Output: Class B
Two concrete classes, B and C, are implementing the interface A. A factory class Factory is created to get an object of A. In the factory design pattern, an interface (or an abstract class) is defined for creating object and the subclasses decide which class to instantiate.
3. What is the problem with the following implementation of the Singleton pattern?
class Singleton
{
private static Singleton obj;
private Singleton() {}
public static Singleton getInstance()
{
if (obj==null)
obj = new Singleton();
return obj;
}
}
This is the classic implementation of Singleton Pattern. This implementation is not thread safe as it's execution creates two objects for singleton.
4. Identify the design pattern that the following code implements.
interface Shape {
void draw();
}
class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Rectangle");
}
}
class Circle implements Shape {
@Override
public void draw() {
System.out.println("Shape: Circle");
}
}
class Display {
private Shape circle;
private Shape rectangle;
public Display() {
circle = new Circle();
rectangle = new Rectangle();
}
public void drawCircle(){
circle.draw();
}
public void drawRectangle(){
rectangle.draw();
}
}
class Demonstration {
public static void main(String[] args) {
Display obj = new Display();
obj.drawCircle();
obj.drawRectangle();
}
}
The above code is an example of Facade design pattern. It involves a single class which provides simplified methods required by client and delegates calls to methods of existing system classes. The aim of the pattern is to hide the complexities of the system from the user.
5. Write a program to buy an item that does not cost more than 5000 using Proxy Design Pattern.
interface Purchase {
void buyItem();
}
class PurchaseItem implements Purchase {
@Override
public void buyItem() {
System.out.println("Item Bought");
}
}
class DesignPattern implements Purchase {
private PurchaseItem object;
int price;
DesignPattern(int amt){
this.price = amt;
}
@Override
public void buyItem() {
if (price <= 5000) {
object = new PurchaseItem();
object.buyItem();
}
else{
System.out.println("Can not purchase item");
}
}
}
class Client {
public static void main(String args[]) {
Purchase obj = new DesignPattern(3000);
obj.buyItem();
}
}
Output: Item Bought
6. Write a program to print the name of the shape given a the number of sides of the shape using Factory Design Pattern. 3 <= N <= 5
interface Shape{
void getType();
}
class Triangle implements Shape {
@Override
public void getType()
{
System.out.println("It's a Triangle");
}
}
class Square implements Shape {
@Override
public void getType()
{
System.out.println("It's a Square");
}
}
class Pentagon implements Shape {
@Override
public void getType()
{
System.out.println("It's a Pentagon");
}
}
class Factory {
public Shape getShape(int sides)
{
if(sides == 3){
return new Triangle();
}
else if(sides == 4){
return new Square();
}
else{
return new Pentagon();
}
}
}
class FactoryPattern{
public static void main(String[] args) {
Factory fact = new Factory();
Shape obj = fact.getShape(4);
obj.getType();
}
}
Output: It's a Square
7. Complete the code to implement a Singleton class.
class Singleton {
private static volatile Singleton instance = null;
private ___________{}
______________ Singleton getInstance() {
if (instance == null) {
synchronized(Singleton.class) {
if (instance == null)
________ = new Singleton();
}
}
________ instance;
}
}
- Singleton()
- public static
- instance
- return
8. Identify the Design Pattern implemeted by the following code
interface ToImplement {
void display();
}
class RealServer implements ToImplement {
@Override
public void display() {
System.out.println("Real Class");
}
}
class Placeholder implements ToImplement{
private RealServer serverObj;
@Override
public void display() {
if(serverObj == null){
System.out.println("Creating Real Server object");
serverObj = new RealServer();
}
serverObj.display();
}
}
class Client {
public static void main(String[] args) {
ToImplement obj = new Placeholder();
obj.display();
obj.display();
}
}
The above code implements Proxy Pattern. According to GoF, a Proxy Pattern provides the control for accessing the original object. It is also known as Placeholder or Surrogate.
9. Complete the following code to implement a Facade Design Pattern.
interface A {
void printClass();
}
class B implements A {
___________
public void printClass() {
System.out.println("This is class B");
}
}
class C implements A {
@Override
public void printClass() {
System.out.println("This is class C");
}
}
class DesignPattern {
private ___ objectC;
private A objectB;
public DesignPattern() {
objectC = new C();
objectB = new B();
}
public void getB(){
objectB._________();
}
public void getC(){
objectC.printClass();
}
}
- @Override
- A
- printClass()
10. Complete the following implementation of Factory Pattern
_________ Car{
void getCompany();
}
______ Hyundai implements Car {
@Override
public void getCompany()
{
System.out.println("Brand Name : Hyundai");
}
}
class Ford ________________ {
@Override
public void getCompany()
{
System.out.println("Brand Name : Ford");
}
}
class Honda implements Car {
@Override
public void _________()
{
System.out.println("Brand Name : Honda");
}
}
class Factory {
public Car getCarBrand(String type)
{
switch(type){
case "Sedan": return new Honda();
case "SUV" : return new Hyundai();
default : return new Ford();
}
}
}
- interface
- class
- implements Car
- getCompany()
11. Identify the design pattern implemented by the following code
interface State {
public void display();
}
class ConcreteStateA implements State {
public void display() {
System.out.println("Concrete State A");
}
}
class ConcreteStateB implements State {
public void display() {
System.out.println("Concrete State B");
}
}
class Context {
private State state;
public Context(){
state = new ConcreteStateA();
}
public void setState(State s){
this.state = s;
}
public void getState(){
state.display();
}
}
class StatePatternDemo {
public static void main(String[] args) {
Context obj = new Context();
obj.getState();
obj.setState(new ConcreteStateB());
obj.getState();
}
}
The code implements State Design Pattern. It is a behavioral design pattern as its Object changes its behavior based on its internal state.
12. Write a program to design a Door with two states - opened and closed using State Design Pattern
interface Door {
public void displayState();
}
class Close implements Door {
public void displayState() {
System.out.println("Door is closed");
}
}
class Open implements Door {
public void displayState() {
System.out.println("Door is opened");
}
}
class Context {
private Door state;
public Context(){
state = new Close();
}
public void setState(Door s){
state = s;
}
public void openDoor(){
state = new Open();
}
public void closeDoor(){
state = new Close();
}
public void getState(){
state.displayState();
}
}
With this article at OpenGenus, you must have a strong foundation in Design Patterns.